Polyfills in Javascript

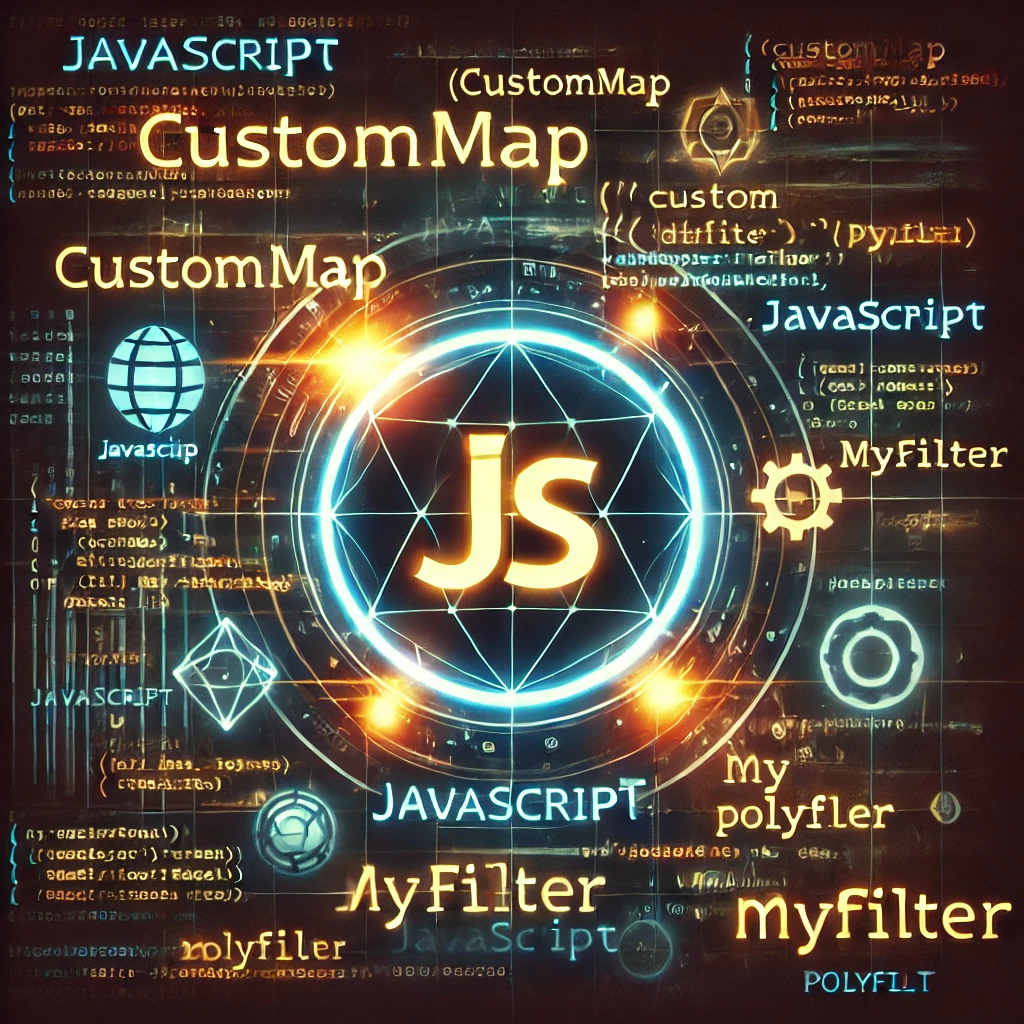
In this article, we explore how JavaScript's built-in methods work and learn how to create custom methods to extend their functionality. We introduce the concept of polyfills, enabling modern functionalities on older browsers. The article provides step-by-step examples to build custom implementations of popular array methods: customMap, myFilter, customForEach, mySlice, and customShift, revealing the inner workings of these methods and demonstrating how to replicate their behavior in JavaScript.
✨🚀 Ever Wondered How JavaScript's Built-in Methods Work? 🤔💡
Well, I got you! 🔥 Let's dive deep into the magic behind these methods and even build our own custom JavaScript methods from scratch! 🛠️💻
What is Polyfill?
A polyfill is a piece of code used to provide modern functionality on older browsers that do not natively support it.
Now we will se some examples of how we can build our own custom methods.
1) Example 1: CustomMap()
const arr = [2,3,4,5,6]
if(!Array.prototype.customMap){
Array.prototype.customMap = function(userFnc){
const res = [];
for(let i = 0; i< this.length; i++){
res.push(userFnc(this[i]))
}
return res;
}
}
const newArr = arr.customMap((el) => el * 3);
console.log(newArr);
//Output:- [6, 9, 12, 15, 18]
Here we built our own customMap method in which we returned a new array wit each element multiplied by 3.
2) Example 2: myFilter()
if(!Array.prototype.myFilter){
Array.prototype.myFilter = function(userFnc){
const res = [];
for(let i = 0; i<this.length; i++){
if(userFnc(this[i])){
res.push(this[i])
}
}
return res;
}
}
const arr = [2,3,4,5,6]
const newArr = arr.myFilter((el) => el % 2 === 0)
console.log(newArr);
//Output :- [2,4,6]
3) Example 3: customForEach()
if(!Array.prototype.customForEach){
Array.prototype.customForEach = function(fnc){
for(let i = 0; i< this.length; i++){
fnc(this[i], i, this);
}
}
}
const arr = ["mango", "apple","banana", "grapes","orange"]
arr.customForEach((el) => console.log(el))
//Output:- ["mango", "apple","banana", "grapes","orange"]
4) Example 4: customSlice()
if (!Array.prototype.mySlice) {
Array.prototype.mySlice = function (strtIndx = 0, endIndx = this.length) {
let result = [];
// Handle negative start index
if (strtIndx < 0) {
strtIndx = Math.max(this.length + strtIndx, 0);
}
// Handle negative end index
if (endIndx < 0) {
endIndx = this.length + endIndx;
}
// Keep endIndx within array length
endIndx = Math.min(endIndx, this.length);
// Extract elements
for (let i = strtIndx; i < endIndx; i++) {
result.push(this[i]);
}
return result;
};
}
let students = ["Ali", "Riya", "John", "Sara", "Aman"];
console.log(students.mySlice(-3, students.length)); // ["John", "Sara", "Aman"]
console.log(students.mySlice(1, 4)); // ["Riya", "John", "Sara"]
console.log(students.mySlice(-2)); // ["Sara", "Aman"]
console.log(students.mySlice()); // ["Ali", "Riya", "John", "Sara", "Aman"]
5) Example 5: customShift()
if(!Array.prototype.customShift){
Array.prototype.customShift = function(){
if(this.length == 0) return undefined;
let firstElement = this[0];
for(let i = 0; i< this.length; i++){
this[i] = this[i+1];
}
this.length = this.length-1;
return firstElement;
}
}
const arr = ["batman", "spiderman", "superman", "ironman", "winter soldier"];
arr.customShift()
console.log(arr);
//Output:- ["spiderman", "superman", "ironman", "winter soldier"]
🚀 Wrapping It Up: Unlocking JavaScript's Hidden Powers! 🔥
By now, you’ve not only uncovered how JavaScript’s built-in methods work but also mastered the art of building your own! 🛠️💡 From customMap()
to customShift()
, you now have the power to replicate and extend JavaScript’s core functionalities like a pro. 💻✨
Subscribe to my newsletter
Read articles from rohit directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
