Building a Simple Two-Axis Robotic Arm Using Potentiometers & Servo Motors
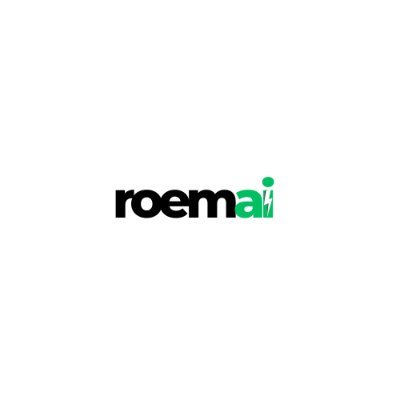

A robotic arm is a machine that can move and rotate like a human arm. In this guide, we will build a simple two-axis robotic arm using an Arduino, servo motors, and potentiometers. This project is perfect for beginners who want to learn about robotics and electronics.
Step 1: Understanding the Components
Before we start, let’s look at the key components we’ll be using:
Arduino Uno – The brain of our robotic arm, which controls everything.
Servo Motors (2x) – Motors that move the arm by rotating from 0 to 180 degrees.
Potentiometers (2x) – Knobs that control the position of the servo motors.
Breadboard and Jumper Wires – Used to connect all components without soldering.
Power Source – The Arduino will be powered via USB.
Now that we know the components, let’s move to wiring them together.
Step 2: Connecting the Circuit
We will connect our servo motors and potentiometers to the Arduino through a breadboard.
Step 2A: Connecting the Servo Motors
Each servo has three wires:
GND (black/brown) → Connect to Arduino GND.
VCC (red) → Connect to Arduino 5V.
Signal (yellow/white) → Connect one servo to Pin 9 and the other to Pin 10.
Step 2B: Connecting the Potentiometers
Each potentiometer has three pins:
GND (one side pin) → Connect to Arduino GND.
VCC (other side pin) → Connect to Arduino 5V.
Signal (middle pin) → Connect one to A0 and the other to A1.
Now, our circuit is ready!
Step 3: Writing the Code
Now, let’s program the Arduino to control the robotic arm. Open the Arduino IDE and upload the following code:
#include <Servo.h>
Servo servo1;
Servo servo2;
int potPin1 = A0;
int potPin2 = A1;
void setup() {
servo1.attach(9);
servo2.attach(10);
Serial.begin(9600);
}
void loop() {
int potValue1 = analogRead(potPin1);
int potValue2 = analogRead(potPin2);
int angle1 = map(potValue1, 0, 1023, 0, 180);
int angle2 = map(potValue2, 0, 1023, 0, 180);
servo1.write(angle1);
servo2.write(angle2);
Serial.print("Servo 1 Angle: ");
Serial.println(angle1);
Serial.print("Servo 2 Angle: ");
Serial.println(angle2);
delay(20);
}
Step 4: Testing the Robotic Arm
After uploading the code to the Arduino:
Turn the potentiometers to see the servo motors move.
The first potentiometer controls the first servo, and the second potentiometer controls the second servo.
Observe how smoothly the arm moves as you adjust the knobs.
Conclusion
Congratulations! You have built a simple two-axis robotic arm. This project teaches you the basics of electronics, coding, and robotics. You can now improve it by adding more joints, using a stronger frame, or controlling it wirelessly!
Happy building!
Watch full tutorial video on how to get it done on YouTube below:
Subscribe to my newsletter
Read articles from Roemai directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
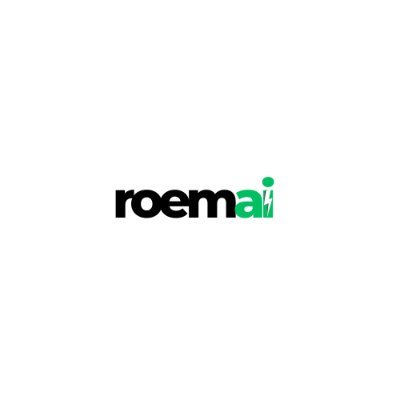
Roemai
Roemai
At Roemai we are empowering individuals through education, innovation, and technology solutions with robotics, embedded systems, and AI.