How to Implement User Authentication in QuickBlox: A Step-by-Step Guide
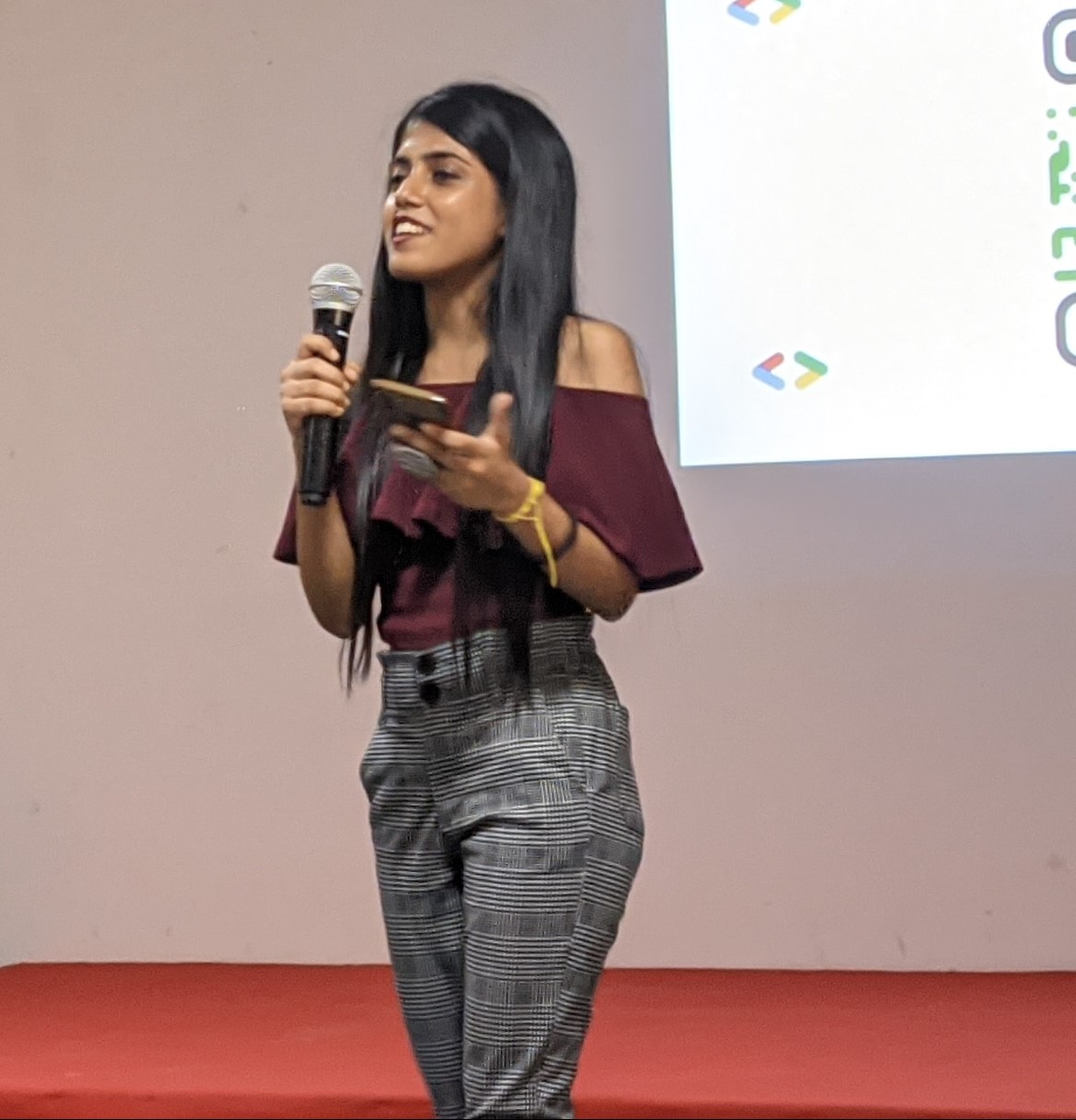

Introduction
Have you ever seen a full-blown chat application without an authentication page?
Authentication is crucial because it ensures secure communication and manages user sessions effectively. QuickBlox is a powerful and easy-to-use platform for adding real-time communication to apps.
Whether you're building a chat app, a customer support tool, or any other communication service, QuickBlox offers authentication features to handle user sessions efficiently.
This guide will walk you through the steps to set up authentication in QuickBlox, including login, signup, and session management.
Project Structure
To keep the project organized, use the following structure:
quickblox-auth-project/
│── index.html # Main HTML file with login/signup forms
│── styles.css # CSS file for styling the authentication pages
│── script.js # JavaScript file for handling authentication logic
│── config.js # Configuration file with QuickBlox credentials
│── README.md # Documentation and project setup instructions
File Breakdown
index.html: Contains the structure for the login and signup forms.
styles.css: Styles the authentication forms for a better user experience.
config.js: Stores QuickBlox credentials to keep them separate from the main logic.
script.js: Manages user authentication, including login, signup, and session handling.
README.md: Provides instructions on setting up and running the project.
Setting Up QuickBlox Authentication
config.js (QuickBlox Initialization)
Before using QuickBlox, you need to initialize it with your credentials. Replace the placeholders with your actual QuickBlox credentials.
const APPLICATION_ID = -1;
const AUTH_KEY = 'your_auth_key';
const AUTH_SECRET = 'your_auth_secret';
const ACCOUNT_KEY = 'your_account_key';
QB.init(APPLICATION_ID, AUTH_KEY, AUTH_SECRET, ACCOUNT_KEY);
console.log('QB initialized successfully');
script.js (Handling Authentication)
Step 1: Implementing User Login
Handles user authentication using QuickBlox login methods.
const loginForm = document.getElementById('loginForm');
loginForm.addEventListener('submit', (e) => {
e.preventDefault();
const userLogin = document.getElementById('login').value;
const userPassword = document.getElementById('password').value;
QB.createSession({ login: userLogin, password: userPassword }, function (error, result) {
if (error) {
console.log('Error in creating session', error);
} else {
console.log('Session created:', result);
QB.login({ login: userLogin, password: userPassword }, function (error, result) {
if (error) {
console.log('Login failed', error);
} else {
console.log('User logged in:', result);
localStorage.setItem('qb_session', QB.service.getSession().token);
}
});
}
});
});
Step 2: Implementing User Signup
Handles user registration.
const signupForm = document.getElementById('signupForm');
signupForm.addEventListener('submit', (e) => {
e.preventDefault();
const username = document.getElementById('username').value;
const email = document.getElementById('email').value;
const password = document.getElementById('signupPassword').value;
QB.users.create({ login: username, password, email }, (error, user) => {
if (error) {
console.log('Signup failed:', error);
} else {
console.log('User created successfully:', user);
}
});
});
Step 3: Implementing Logout
Clears the session and logs out the user.
const logoutButton = document.getElementById('logoutButton');
logoutButton.addEventListener('click', () => {
QB.logout((error, response) => {
if (error) {
console.log('Logout failed:', error);
} else {
localStorage.removeItem('qb_session');
console.log('User logged out successfully');
}
});
});
Step 4: Managing User Sessions
Checks if a user is already logged in by verifying the session token in localStorage
.
if (localStorage.getItem('qb_session')) {
console.log('User is already logged in');
}
Conclusion
This guide walks you through setting up authentication in QuickBlox using a structured approach. You now have a project template that separates concerns and keeps authentication logic clean and manageable. Check out the QuickBlox documentation for more details!
Subscribe to my newsletter
Read articles from Sayantani Deb directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
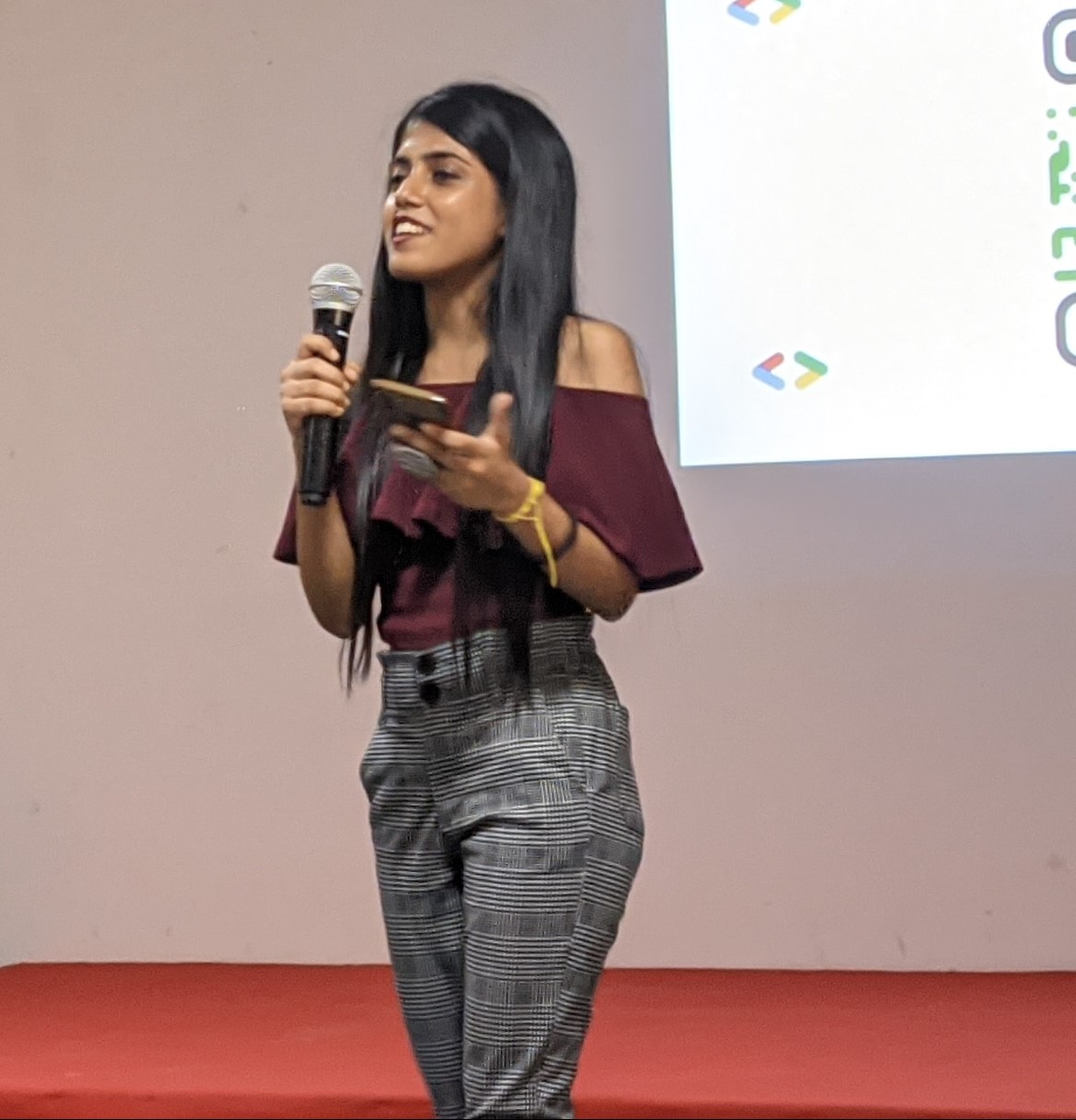
Sayantani Deb
Sayantani Deb
A Javascript Porgrammer who loves to build cool projects in the weekends!