JavaScript Teleportation: Unraveling Serialization and Deserialization

Table of contents
- What is Serialization? - "Demystifying Serialization: Transforming Data for Transmission"
- What is Deserialization? - "Decoding Deserialization: Reconstructing Data Structures"
- Why we use Serialization and Deserialization? - "The Necessity of Serialization and Deserialization in Data Exchange"
- Formats other than JSON - "Beyond JSON: Exploring Alternative Serialization Formats"
- Conclusion
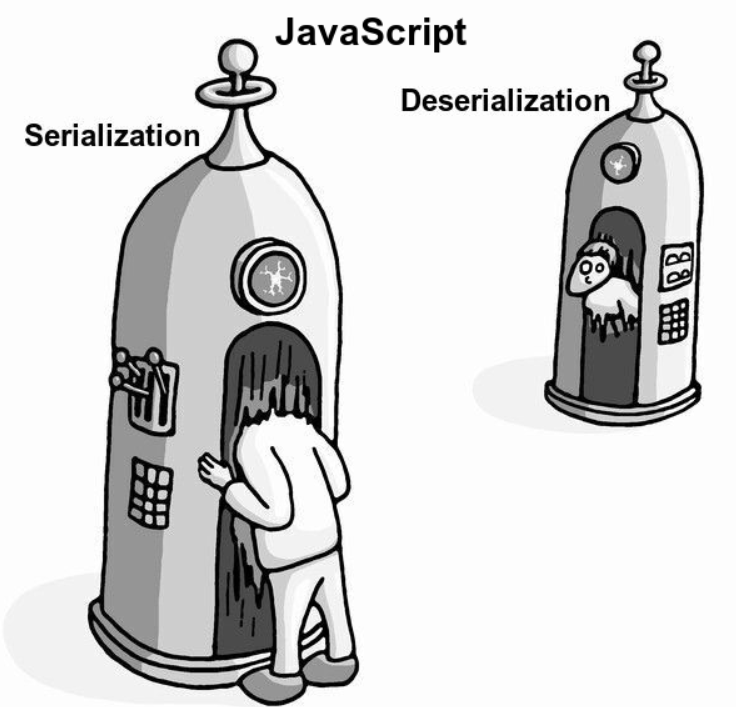
In our world, human teleportation has always fascinated many people, including me. However, the technology to teleport humans is still far off in the future. To teleport humans from one place to another, we would need to first convert them into some form of energy or object, send them through a network, and then convert this energy or object back into a human. In web development, we do something similar by teleporting objects (data structures) in JavaScript. We convert them into strings or other formats, send them through a network, and once they reach their destination, we convert them back to their original objects. Let's explore the concept of serialization and deserialization in JavaScript using the analogy of human teleportation.
What is Serialization? - "Demystifying Serialization: Transforming Data for Transmission"
Serialization is the process of converting a data structure, such as JavaScript object or array, into a format that can easily be stored and transmitted. Lets understand it using an example,
In movies like "Star Trek," teleportation is done using a machine called "The Transporter." The Transporter first converts the human body into an energy pattern (a process called "dematerialization") and then sends it ("beaming") to the target location. Similarly, a JavaScript object is converted into JSON format.
const person = {
name: "Himannshu",
age: 20,
weight: "60 Kg"
}
const JSON_string = JSON.stringify(person)
// output : '{"name":"Himanshu", "age":20, "weight":"60 Kg"}'
In this code, we use the JSON.stringify()
method to convert the person
object into a JSON string.
What is Deserialization? - "Decoding Deserialization: Reconstructing Data Structures"
Deserialization is the opposite of serialization. In this process, the serialized data is turned back into a data structure or object. In JavaScript, this means converting a JSON string into a JavaScript object.
When The Transporter sends the energy pattern to the intended location, then the energy pattern in converting into matter ("rematerialization"). The command often used to request activation of the transporter is "Energize."
const JSON_string = '{"name":"Himanshu", "age":20, "weight":"60 Kg"}'
const person = JSON.parse(JSON_string)
// output : {name: "Himanshu", age:20, weight: "60 Kg"}
In this code, we convert the JSON string back to object using JSON.parse()
.
Why we use Serialization and Deserialization? - "The Necessity of Serialization and Deserialization in Data Exchange"
Now that you know what Serialization and Deserialization are, you might be asking, "Why do we need them?" Let's explore the reasons using a messaging application as an example.
Imagine you are developing a messaging app that lets users send and receive messages instantly. Each message includes data like the sender's name, the message itself, and a timestamp. To send this data over the network, we need to convert it into a format that can be easily transmitted (using Serialization) and then change it back to the original data (using Deserialization) on the receiver's end.
From this example, we understand that we use serialization to convert data into a format that is easy to send and receive. We use deserialization to change the data back to its original form.
Formats other than JSON - "Beyond JSON: Exploring Alternative Serialization Formats"
Let's first understand what JSON is.
What is JSON? - "Understanding JSON: The Universal Data Interchange Format"
JSON stands for JavaScript Object Notation. It is a lightweight data interchange format that easy for humans to read and write, and easy for machines to parse and generate. It is a text based format that is used to represent structured data based on JavaScript syntax. Despite its origin from JavaScript, JSON is language-independent and is widely used for data interchange between different systems and programming language.
{
"name": "Himanshu",
"age": 20,
"weight": "60 Kg",
}
JSON is commonly used in web applications for exchanging data between a client and a server, often in the context of RESTful APIs. Its simplicity and wide support make it a preferred choice for many developers when working with data interchange.
Other Formats - "Diving into Alternative Formats"
There are many formats other than JSON which can serialize and deserialize the data.
BSON
BSON is a binary-encoded data interchange format designed to be more efficient in terms of storage and transmission compared to JSON. It extends JSON with additional data types and is typically used in the context of MongoDB.
Example of serialization and deserialization of BSON using bson
package :
import { BSON, ObjectId } from 'bson'
const data = { _id: new ObjectId(), name: "John", age: 30}
// serialization
const bytes = BSON.serialize(data)
// output : <Buffer 2e 00 00 00 07 5f 69 64 00 64 ca 53 01 9d 14 77 b6 a1 a2 89 e4 02 6e 61 6d 65 00 05 00 00 00 4a 6f 68 6e 00 10 61 67 65 00 1e 00 00 00 00>
// deserialization
const doc = BSON.deserialize(bytes)
// Output: {
// _id: new ObjectId("64ca53019d1477b6a1a289e4"),
// name: 'John',
// age: 30
// }
EJSON
Extended JSON (EJSON) is a superset of JSON, which means it includes all features of standard JSON and extends it additional conventions for representing certain data type and MongoDB specific constructs.
Example of serialization and deserialization of EJSON using bson
package :
import { EJSON } from 'bson'
const data = {"_id": {"$oid": "613e8693499f15328a17e8b1" }, "name": "John", "age": 30 }
// serialization
const jsonString = EJSON.stringify(data, {relaxed: false})
// Output: '{"_id":{"$oid":"613e8693499f15328a17e8b1"},"name":"John","age":{"$numberInt":"30"}}'
// Deserialization
const parsedData = EJSON.parse(jsonString);
console.log("Deserialized Data:", parsedData);
// Output: {
// _id: new ObjectId("613e8693499f15328a17e8b1"),
// name: 'John',
// age: new Int32(30)
// }
These are some the formats used other than JSON.
Conclusion
In conclusion, serialization and deserialization are essential processes in web development that allow data to be efficiently transmitted and reconstructed across different systems. By converting data structures into formats like JSON, BSON, or EJSON, developers can ensure seamless data exchange between clients and servers. Understanding these processes not only enhances the efficiency of data handling but also broadens the scope of interoperability between various programming languages and platforms. As we continue to explore and utilize different serialization formats, we can optimize data storage and transmission, much like the futuristic concept of teleportation, making data transfer as seamless and efficient as possible.
Subscribe to my newsletter
Read articles from himanshu directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
