Make Users Smile: Optimize Your Flutter App's Performance
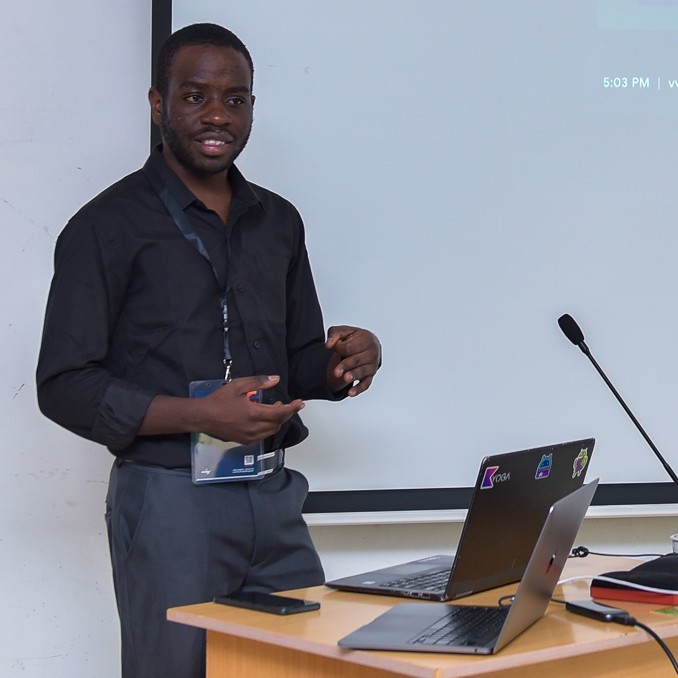
Table of contents
- INTRODUCTION
- Techniques You Apply to Achieve Optimization
- 1. Detect Performance Bottlenecks Proactively
- 2. Optimize Animations for Low-End Devices
- 3. Optimize Resource Management
- 4. Offload Heavy Tasks with Isolates
- 5. Advanced Optimization Techniques (Airbnb’s Secret Sauce)
- A. Shader Precompilation
- B. State Management Tuning
- C. Network Optimization
- 6. Test Like Airbnb: Real-World Validation
- Key Takeaways

INTRODUCTION
Performance optimization isn’t just a nice-to-have—it’s a necessity for large scale mobile apps like Airbnb that cater to millions of users across diverse devices. Slow apps frustrate users, increase uninstall rates, and harm brand reputation. Before we dive in into how we can achieve optimization for our Flutter apps we will first look at why we need the optimization to begin with.
Why Performance Optimization Matters
1. User Retention & Engagement
Fact: 70% of users abandon apps that take more than 3 seconds to load.
Impact: Slow apps frustrate users, leading to uninstalls. For example, Pinterest improved perceived load times by 40%, boosting user engagement by 15%.
2. Global Market Penetration
Emerging Markets: Over 60% of smartphone users in regions like India and Africa use low-RAM devices (<4GB). A laggy app alienates this massive audience.
Case Study: Lite apps have been built specifically for low-end devices, resulting in a 30% increase in installations in emerging markets.
3. Conversion Rates & Revenue
E-commerce Impact: Amazon found that every 100ms delay in load time cost them 1% in sales.
App Example: Walmart saw a 2% increase in conversions for every 1-second improvement in load time.
4. Battery Efficiency
User Loyalty: Apps draining battery life are uninstalled 2.5x faster.
Technical Angle: Heavy animations and background tasks force CPUs/GPUs to work harder, shortening battery life.
5. Search Engine Rankings (SEO)
Web Apps: Google’s Core Web Vitals metrics (e.g., Largest Contentful Paint) directly affect SEO rankings. Slow Flutter web apps lose visibility.
Data: Pages meeting Core Web Vitals thresholds have a 24% lower bounce rate.
6. Competitive Differentiation
Stand Out: Apps like Instagram and Airbnb prioritize performance to stay ahead. A smooth app can be your unique selling point (USP) in crowded markets.
Example: TikTok’s success is partly due to its seamless scroll and load times, even on low-end devices.
7. Reduced Support Costs
Hidden Savings: Poor performance leads to 5x more support tickets for crashes and lag. Optimizing reduces maintenance overhead.
Netflix Example: By optimizing startup time, Netflix cut support queries by 20%.
8. Accessibility & Inclusivity
Broader Reach: Slow apps exclude users with older devices or disabilities.
WCAG Compliance: Performance optimizations (e.g., reduced animations) align with Web Content Accessibility Guidelines (WCAG).
9. App Store Rankings
Algorithm Boost: Both Google Play and Apple’s App Store prioritize apps with lower crash rates and faster load times.
Data: Apps with a 5-star rating (often tied to performance) get 4.6x more downloads than 1-star apps.
10. Environmental Impact
- Sustainability: Efficient code reduces energy consumption. For example, Google reduced YouTube’s carbon footprint by 50% via performance tweaks.
Techniques You Apply to Achieve Optimization
The Hidden Cost of Ignoring Performance reveals concerning statistics: 88% of users are less likely to return to a site or app after a bad experience according to Adobe, and a user who uninstalls your app costs 5x more to reacquire than to retain. While Airbnb achieves smooth performance natively, you can accomplish the same in Flutter. In this article, I'll dissect how Airbnb ensures smooth performance in their apps and translate those strategies into actionable steps for Flutter developers. Let's dive in!
1. Detect Performance Bottlenecks Proactively
Monitor Frame Rates with SchedulerBinding
.
Airbnb uses real-time metrics to detect lag. In Flutter, you can track frame rendering times to dynamically adjust UI complexity:
void checkPerformance() {
SchedulerBinding.instance.addPostFrameCallback((_) {
final frameTime = SchedulerBinding.instance.drawFrameDuration;
if (frameTime > const Duration(milliseconds: 16)) { // 60 FPS threshold
setState(() => isLowPerformance = true);
}
});
}
Why this works: Flutter targets 60 FPS (16ms per frame). If rendering exceeds this, simplify the UI.
Tools to Profile Performance
Flutter DevTools: Identify junk with the Performance Overlay (
flutter run --profile
).Firebase Performance Monitoring: Track metrics like startup time and frame rates in production.
2. Optimize Animations for Low-End Devices
Dynamically Disable Animations
Use TickerMode
to toggle animations based on device health:
TickerMode(
enabled: !isLowPerformance,
child: SlideTransition(
position: _animationController.drive(CurveTween(curve: Curves.easeOut)),
),
);
Alternative Strategies
Simplify Animations: Replace complex Lottie animations with static SVGs.
Lower Frame Rates: Reduce
AnimationController
duration for low-end devices:
_animationController.duration = isLowPerformance
? const Duration(milliseconds: 300)
: const Duration(milliseconds: 500);
3. Optimize Resource Management
Efficient List Rendering
ListView.builder
is your friend, but go further:
Use
itemExtent
: Fix item height to avoid expensive layout recalculations.Avoid
shrinkWrap
: It forces widgets to compute their size upfront, causing delays.
Image Optimization
- Lazy Loading: Use
cached_network_image
with placeholders:
CachedNetworkImage(
imageUrl: "https://example.com/image.jpg",
placeholder: (context, url) => Container(color: Colors.grey[300]),
);
- Resize Images: Serve lower-resolution images for slow devices using URL parameters (e.g.,
image.jpg?width=400
).
4. Offload Heavy Tasks with Isolates
Prevent UI Freezes
Long-running tasks (e.g., data parsing, complex calculations) should run in isolates:
Future<void> processData() async {
final receivePort = ReceivePort();
await Isolate.spawn(_heavyTask, receivePort.sendPort);
}
void _heavyTask(SendPort sendPort) {
// Your compute-heavy logic here
sendPort.send(result);
}
When to Use Isolate
vs compute()
compute()
: Short-lived tasks (< 5 seconds). Simple syntax.Isolate
: Long-running tasks. More control (e.g., two-way communication).
5. Advanced Optimization Techniques (Airbnb’s Secret Sauce)
A. Shader Precompilation
Avoid first-run junk by pre-warming shaders during app launch:
void warmUpShaders() {
WidgetsFlutterBinding.ensureInitialized();
final FlutterView view = WidgetsBinding.instance.platformDispatcher.views.first;
await FragmentProgram.precompile(view.context, assetKey: 'shaders/my_shader.frag');
}
B. State Management Tuning
Use
const
widgets to minimize rebuilds.Adopt
Provider
orRiverpod
for granular state updates.
C. Network Optimization
Batch API Requests: Reduce round trips for data-heavy screens.
Cache Strategically: Use
dio
orhttp
withshared_preferences
for offline support.
6. Test Like Airbnb: Real-World Validation
Device Lab Testing: Run on low-end devices (e.g., Android Go Edition).
User Feedback Loops: Monitor app store reviews for performance complaints.
Automate Profiling: Integrate performance checks into CI/CD pipelines.
Key Takeaways
Monitor Early: Use
SchedulerBinding
to detect lag before users do.Adapt Dynamically: Simplify UI/animations for low-end devices.
Isolate Wisely: Keep the UI thread free for rendering.
Test Relentlessly: Performance is a feature—build it into your workflow.
By adopting these strategies, you’re not just coding—you’re engineering resilient, user-first apps. As Airbnb proves, performance optimization isn’t magic; it’s a mindset. Ready to make your Flutter app bulletproof? � Start optimizing today!
Further Reading:
Airbnb Engineering Blog (for native optimization insights)
Subscribe to my newsletter
Read articles from Siro Daves directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
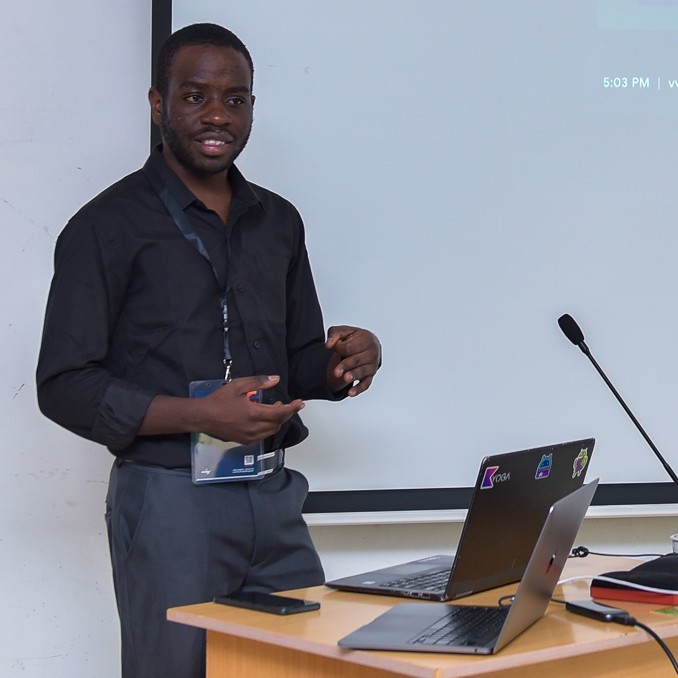
Siro Daves
Siro Daves
Software engineer and a Technical Writer, Best at Flutter mobile app development, full stack development with Mern. Other areas are like Android, Kotlin, .Net and Qt