Serialization & Deserialization in JavaScript
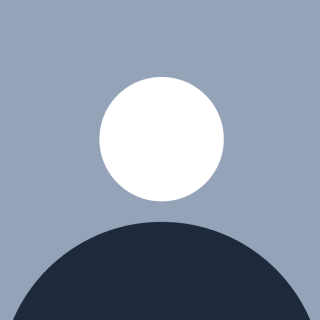
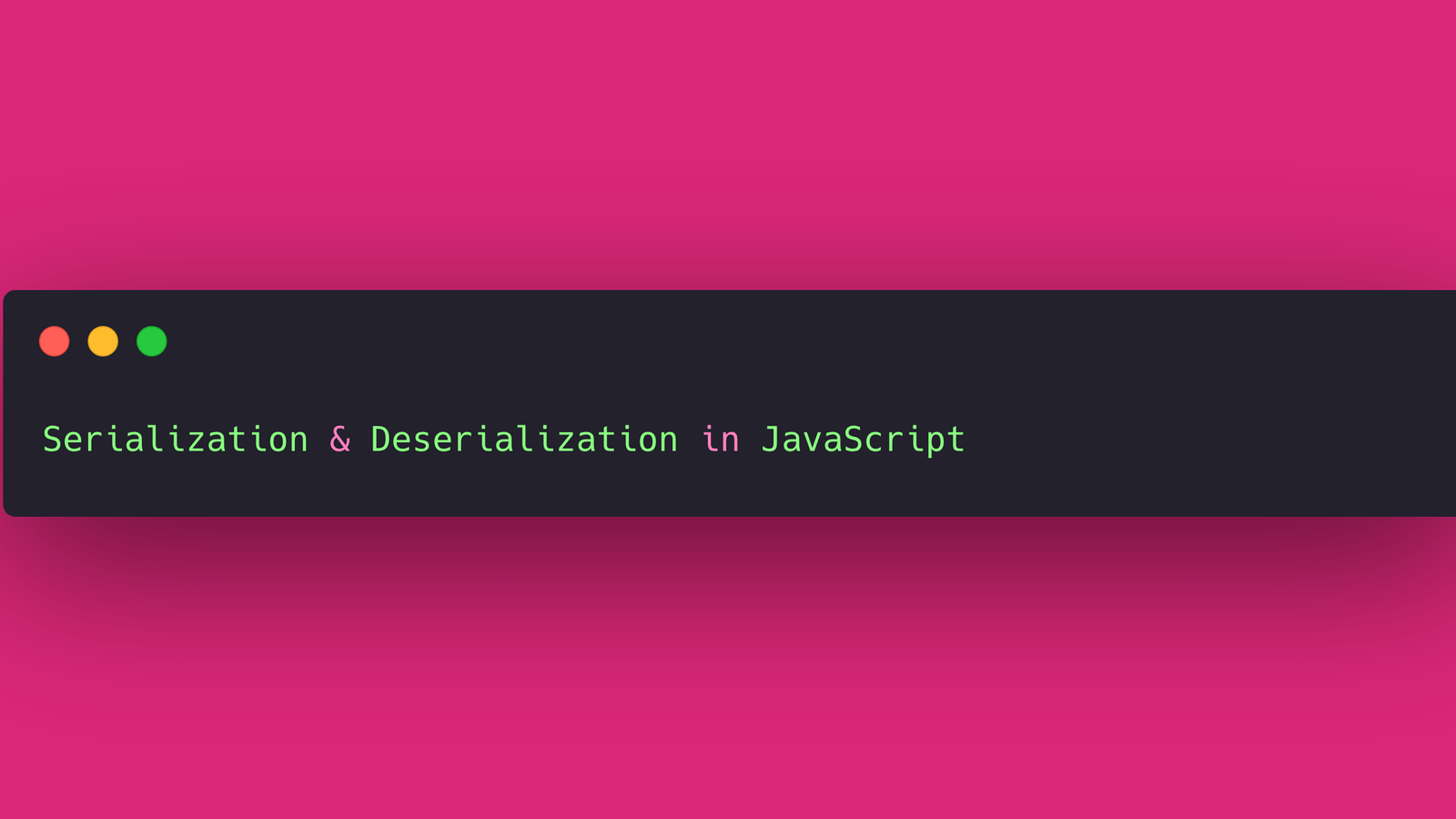
Serialization and deserialization are processes used to convert data into a format that can be stored or transmitted and then reconstruct it back into its original form.
1. Serialization
Serialization is the process of converting objects, arrays, or data structures into a format (like a string or binary) that can be stored or transferred.
In JavaScript, the most common serialization format is JSON (JavaScript Object Notation). The JSON format is easy for both human and machine to read and write.
Example: Converting an Object to JSON
const person = {
name: "Sumit",
age: 22,
skills: ["JavaScript", "Node.js", "React"]
};
const serializedData = JSON.stringify(person);
console.log(serializedData);
// Output: '{"name":"Sumit","age":22,"skills":["JavaScript","Node.js","React"]}'
Here, JSON.stringify()
converts the JavaScript object into a string, making it easier to send over a network or store in a file.
How It Works:
Reads object properties – It goes through each key-value pair in an object.
Converts values – Numbers, strings, and arrays are converted into JSON format.
Removes unsupported values –
functions
,undefined
, andSymbol
values are not included.
Limitations:
Functions and undefined are ignored
const obj = { name: "Sumit", age: undefined, greet: () => "Hello" }; console.log(JSON.stringify(obj)); // {"name":"Sumit"} // ( gree() funtion and age are removed )
- Date objects become strings
co
nst obj = { today: new Date() };
console.log(JSON.stringify(obj)); // {"today":"2025-02-11T12:00:00.000Z}
- Circular references cause errors
let obj = {};
obj.self = obj; // Circular reference
console.log(JSON.stringify(obj)); // ❌ Throws an error
This happens because JSON needs a clean and simple structure to work properly across different systems.
2. Deserialization
Deserialization is the process of converting serialized data back into an object.
It converts data in its serialized format into its original data structure, such as a JavaScript object or array, to make the data usable and accessible in the application.
Example: Parsing JSON Back into an Object:
const jsonData = '{"name":"Sumit","age":22,"skills":["JavaScript","Node.js","React"]}';
const userObj = JSON.parse(jsonData);
console.log(userObj);
// Output: { name: 'Sumit', age: 22, skills: [ 'JavaScript', 'Node.js', 'React' ] }
Here, JSON.parse()
converts the JSON string back into a JavaScript object.
How JSON Parsing Works (Under the Hood):
Reads the JSON string character by character.
Matches data types (like strings, numbers, and arrays) with JavaScript types.
Rebuilds objects and arrays as they were before serialization
3. Alternative Serialization Methods
Not only JSON, there are numerous serialization formats available, as listed below:
- BSON (Binary JSON) – Used in MongoDB, supports more data types.
// Example: MongoDB stores data in BSON format
db.collection.insertOne({ name: "Sumit", createdAt: new Date() });
- MessagePack – More compact than JSON.
const msgpack = require("msgpack-lite");
const data = { name: "Sumit", age: 22 };
const encoded = msgpack.encode(data); // Compress data
const decoded = msgpack.decode(encoded); // Decompress data
- Protocol Buffers (Protobuf) – Used in high-performance applications.
message User {
string name = 1;
int32 age = 2;
}
Conclusion
Serialization helps in converting JavaScript objects into a format that can be stored or transferred, while deserialization reconstructs them back. Understanding this is essential for full-stack development, API handling, and working with databases.
Subscribe to my newsletter
Read articles from sumit mokasare directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
sumit mokasare
sumit mokasare
Hi, I'm Sumit! A first-year BCA student passionate about full-stack development. I share my learning journey and coding concepts to help others grow with me.