How to Create a Rock Paper Scissors Game with HTML, CSS, and JavaScript
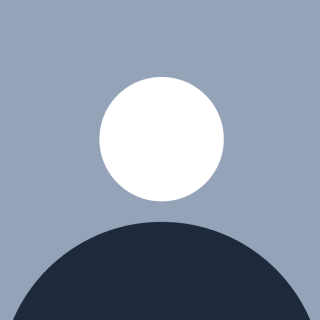

Introduction :
Creating a Rock Paper Scissors game using HTML, CSS, and JavaScript is a fun and engaging way to enhance your web development skills. This classic hand game, known for its simplicity and strategic depth, provides an excellent opportunity to practice coding fundamentals and explore interactive web design. In this tutorial, you'll learn how to build a fully functional game interface, implement game logic, and add dynamic styling to create an enjoyable user experience. Whether you're a beginner looking to learn the basics or an experienced developer seeking a quick project, this guide will walk you through each step of the process. Let's dive in and start coding your own Rock Paper Scissors game!
Work-Flow :
Set Up the HTML Structure:
Create a basic HTML file with a structure that includes a section for displaying the game options (Rock, Paper, Scissors) and a section for showing the results.
Add buttons or images for each of the game choices that the user can click on.
Style with CSS:
Use CSS to style the game interface, making it visually appealing. This includes setting up the layout, colors, fonts, and any animations or transitions for the buttons.
Ensure the game is responsive and looks good on different screen sizes.
Implement Game Logic with JavaScript:
Write JavaScript functions to handle the game logic. This includes:
Capturing the user's choice when they click a button.
Randomly generating the computer's choice.
Comparing the user's choice with the computer's choice to determine the winner.
Updating the results section to display the outcome of each round.
Add Interactivity:
Use event listeners to detect when a user clicks on a game option and trigger the game logic.
Update the user interface dynamically based on the game results, such as highlighting the winning choice or displaying a message.
Enhance User Experience:
Add features like score tracking to keep a tally of wins, losses, and ties.
Consider adding sound effects or animations to make the game more engaging.
Test and Debug:
Test the game thoroughly to ensure all features work as expected.
Debug any issues that arise and refine the code for better performance and readability.
Deploy and Share:
Once the game is complete and tested, deploy it to a web server or platform where others can play it.
Share the game with friends or on social media to get feedback and enjoy the creation.
exmaple : github page , netlify etc.
HTML CODE
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Stone Paper Scissors - Sudhir Patel</title>
<link rel="stylesheet" href="stone.css">
</head>
<body>
<div>
<h1>Rock, Paper, and Scissors Game</h1>
</div>
<div class="choice">
<div class="img" id="paper">
<img src=".//paper.png" alt="Paper">
</div>
<div class="img" id="rock">
<img src=".//rock.png" alt="rock">
</div>
<div class="img" id="scissors">
<img src=".//scissors.png" alt="Scissors">
</div>
</div>
<div class="result">
<div class="user-score">
<p id="user-score">User Score: 0</p>
</div>
<div class="comp-score">
<p id="comp-score">Computer Score: 0</p>
</div>
</div>
<div class="msg-container">
<p id="msg">Get Ready For The Battle</p>
</div>
<script src="stone.js"></script>
</body>
</html>
The given code is an HTML document for a "Rock, Paper, Scissors" game. It includes a basic structure with a title and links to a CSS file for styling. The body contains a header with the game title and three sections for the player's choices: paper, rock, and scissors, each represented by an image. There are also two sections to display the scores for the user and the computer, both starting at zero. A message container displays a prompt to get ready for the game. At the end, a JavaScript file is linked to handle the game's logic.
CSS CODE
*{
margin: 0;
padding: 0;
background-color: #1A1A1D;
}
h1{
text-align: center;
background-color: #1A1A1D;
font-family: 'Segoe UI', Tahoma, Geneva, Verdana, sans-serif;
color: aliceblue;
padding-bottom: 20px;
padding-top: 20px;
text-shadow: rgb(247, 28, 75) 0px 0px 892.419px,
rgb(247, 28, 75) 0px 0px 509.954px,
rgb(247, 28, 75) 0px 0px 297.473px,
rgb(247, 28, 75) 0px 0px 148.736px,
rgb(247, 28, 75) 0px 0px 42.4961px,
rgb(247, 28, 75) 0px 0px 21.2481px;
}
.img{
height: 200px;
width: 200px;
border-radius: 50px;
}
img{
height: 180px;
width: 180px;
border-radius: 50%;
}
.choice{
display: flex;
align-items: center;
justify-content: center;
margin-top: 50Px;
gap : 20px;
}
#paper:hover{
background-color: rgb(135, 206,235);
border-radius: 100px;
justify-content: center;
align-items: center;
cursor: pointer;
box-shadow: rgb(135, 206,235) 0px 0px 100px ,
rgb(135, 206,235) 0px 0px 40px ,
rgb(135, 206,235) 0px 0px 40px ,
rgb(135, 206,235) 0px 0px 40px ;
}
#rock:hover{
background-color: lightpink;
border-radius: 100px;
justify-content: center;
align-items: center;
cursor: pointer;
box-shadow:
rgb(255, 192, 203) 0px 0px 100px,
rgb(255, 192, 203) 0px 0px 40px,
rgb(255, 192, 203) 0px 0px 40px,
rgb(255, 192, 203) 0px 0px 40px;
}
#scissors:hover{
background-color: yellow;
border-radius: 100px;
justify-content: center;
align-items: center;
cursor: pointer;
box-shadow:
rgb(255, 255, 0) 0px 0px 100px,
rgb(255, 255, 0) 0px 0px 40px,
rgb(255, 255, 0) 0px 0px 40px,
rgb(255, 255, 0) 0px 0px 40px;
}
.result{
display: flex;
justify-content: center;
margin-top: 100px;
gap: 50px;
font-size: 40px;
color: aqua;
text-decoration-color: black;
text-shadow: blue;
}
#msg{
text-align: center;
font-size: 40px;
margin-top: 15px;
color: red;
}
The given code is a set of CSS styles for a webpage. It defines how certain elements should look and behave. Here's a breakdown:
There are styles for three elements:
#rock
,#rock:hover
, and#scissors:hover
.The
#rock
element has a light blue box shadow and a circular shape with a pointer cursor.When you hover over
#rock
, its background changes to light pink, and the box shadow changes to a pink color.When you hover over
#scissors
, its background changes to yellow, and the box shadow changes to a yellow color.The
.result
class is styled to display its content in a flexible, centered layout with a large font size and aqua color. It also has a blue text shadow.The
#msg
element is centered with a large font size and red color. It has a small top margin.
JS CODE:
let userScore = 0;
let compScore = 0;
const choices = document.querySelectorAll(".img");
const result = document.querySelector("#msg");
const gencompchoice = () => {
const options = ["rock", "paper", "scissors"];
const random = Math.floor(Math.random() * 3);
return options[random];
}
let userChoice;
let compChoice;
// Function for determining the winner
const showWinner = (userWin) => {
if (userWin) {
userScore++;
console.log("You Win !!!");
result.style.color = "white";
result.innerText = `You Win !!! Your ${userChoice} beats ${compChoice}`;
} else {
compScore++;
console.log("You Lose !!!");
result.style.color = "red";
result.innerText = `You Lose !!! Your ${userChoice} was beaten by ${compChoice}`;
}
document.getElementById("user-score").innerText = `User Score: ${userScore}`;
document.getElementById("comp-score").innerText = `Computer Score: ${compScore}`;
}
// For drawing the game
const Draw = () => {
console.log("The game was a draw.");
result.style.color = "orange";
result.innerText = "The Game was Draw !!! - Both options are the same";
}
// Function to play the game
const playgame = (userChoice) => {
console.log("User choice =", userChoice);
// Computer choice
compChoice = gencompchoice();
console.log("Computer choice =", compChoice);
if (userChoice === compChoice) {
// Draw the game
Draw();
} else {
let userWin = true;
if (userChoice === "rock") {
// Options: paper or scissors
userWin = compChoice === "paper" ? false : true;
} else if (userChoice === "paper") {
userWin = compChoice === "scissors" ? false : true;
} else {
userWin = compChoice === "rock" ? false : true;
}
showWinner(userWin);
}
}
// Event listeners for each choice
choices.forEach((choice) => {
choice.addEventListener("click", () => {
userChoice = choice.getAttribute("id");
playgame(userChoice);
});
});
The code is a simple game where a user plays against the computer by choosing between rock, paper, or scissors. Here's how it works:
The function
Draw
is called when the game ends in a tie. It logs a message to the console and updates the result text to indicate a draw, coloring it orange.The
playgame
function takes the user's choice as input. It generates a computer choice using thegencompchoice
function and logs both choices.If the user's choice matches the computer's choice, the game is a draw, and the
Draw
function is called.If the choices are different, the code determines if the user wins. It checks the user's choice against the computer's choice to decide the winner.
The
showWinner
function is called with the result of whether the user won or not.Event listeners are added to each choice option. When a user clicks on a choice, it triggers the
playgame
function with the selected choice.
Github Link : https://github.com/sudhirskp/Rock-paper-game
This article guides you through creating a Rock Paper Scissors game using HTML, CSS, and JavaScript. It covers setting up the HTML structure for game options and results, styling the interface for visual appeal, implementing game logic with JavaScript, and adding interactivity with event listeners. Additionally, it provides tips on enhancing user experience with features like score tracking and animations, testing and debugging the game, and deploying it on platforms like GitHub or Netlify for wider sharing.
Subscribe to my newsletter
Read articles from SUDHIR PATEL directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by