Develop Custom WordPress CRUD Plugin
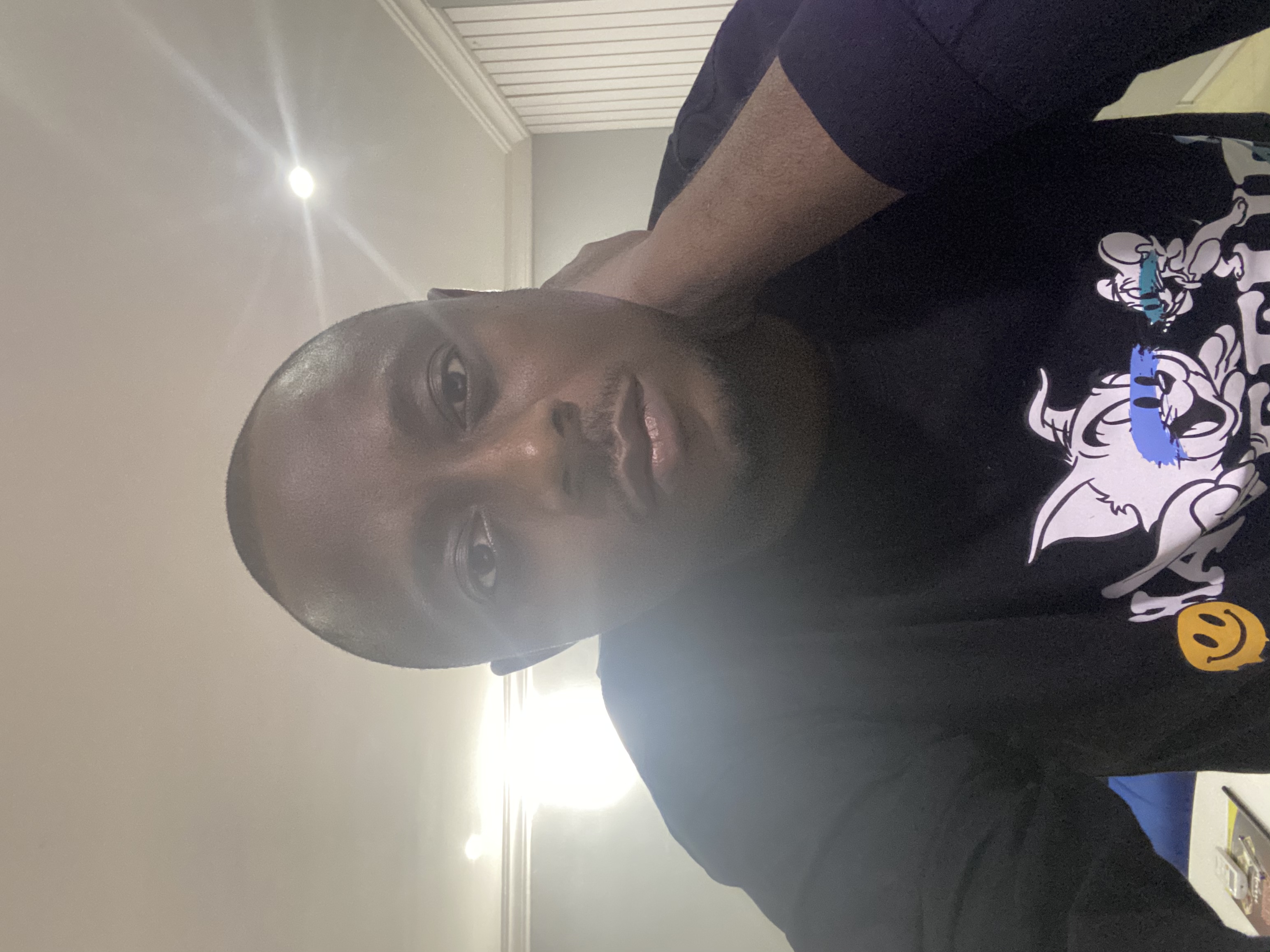

WordPress is a powerful CMS, but sometimes we need custom solutions for handling data beyond just posts and pages. A CRUD (Create, Read, Update, Delete) operation allows developers to build custom database-driven applications within WordPress. In this guide, we will walk through creating a WordPress CRUD plugin, handling data efficiently, and ensuring a seamless user experience.
What is CRUD?
CRUD stands for:
Create: Insert new records into a database.
Read: Retrieve and display existing records.
Update: Modify existing records.
Delete: Remove records from the database.
In WordPress, CRUD operations are commonly used for custom post types, user management, and interacting with custom database tables.
Why Use a Custom WordPress CRUD Plugin?
Better data organisation: Store information in a structured manner.
Admin panel management: Create an easy-to-use interface for managing data.
Flexibility: Control how data is stored, retrieved, and displayed.
๐ Folder Structure
You'll create the following folder and files inside your WordPress plugins directory:
/wp-content/plugins/wp-crud-plugin/
โโโ wp-crud-plugin.php # Main plugin file
โโโ includes/
โ โโโ db.php # Database setup
โ โโโ admin-page.php # Admin page UI & logic
โ โโโ crud-functions.php # CRUD operations
โโโ assets/
โ โโโ style.css # Styles for the admin UI
โโโ uninstall.php # Cleanup script for deleting data on uninstall
๐ Step 1: Create Plugin Folder & Main File
๐ Folder: /wp-content/plugins/wp-crud-plugin/
๐ File: /wp-content/plugins/wp-crud-plugin/wp-crud-plugin.php
๐ Content:
<?php
/**
* Plugin Name: Custom WP CRUD Plugin
* Plugin URI: https://example.com
* Description: A simple WordPress plugin for CRUD operations.
* Version: 1.0
* Author: Your Name
* License: GPL2
*/
if (!defined('ABSPATH')) {
exit; // Prevent direct access
}
// Define plugin path
define('WP_CRUD_PLUGIN_DIR', plugin_dir_path(__FILE__));
// Include files
require_once WP_CRUD_PLUGIN_DIR . 'includes/db.php';
require_once WP_CRUD_PLUGIN_DIR . 'includes/admin-page.php';
require_once WP_CRUD_PLUGIN_DIR . 'includes/crud-functions.php';
// Activation Hook (Create DB Table)
register_activation_hook(__FILE__, 'wp_crud_create_table');
// Enqueue Styles
function wp_crud_enqueue_assets() {
wp_enqueue_style('wp-crud-style', plugins_url('assets/style.css', __FILE__));
}
add_action('admin_enqueue_scripts', 'wp_crud_enqueue_assets');
๐ Step 2: Create Database Setup File
๐ Folder: /wp-content/plugins/wp-crud-plugin/includes/
๐ File: /wp-content/plugins/wp-crud-plugin/includes/db.php
๐ Content:
<?php
// Database Table Creation
function wp_crud_create_table() {
global $wpdb;
$table_name = $wpdb->prefix . 'custom_data';
$charset_collate = $wpdb->get_charset_collate();
$sql = "CREATE TABLE $table_name (
id INT NOT NULL AUTO_INCREMENT,
name VARCHAR(100) NOT NULL,
email VARCHAR(100) NOT NULL,
PRIMARY KEY (id)
) $charset_collate;";
require_once ABSPATH . 'wp-admin/includes/upgrade.php';
dbDelta($sql);
}
๐ Step 3: Create CRUD Operations File
๐ Folder: /wp-content/plugins/wp-crud-plugin/includes/
๐ File: /wp-content/plugins/wp-crud-plugin/includes/crud-functions.php
๐ Content:
<?php
// Insert Record
function wp_crud_insert($name, $email) {
global $wpdb;
$table_name = $wpdb->prefix . 'custom_data';
return $wpdb->insert($table_name, ['name' => sanitize_text_field($name), 'email' => sanitize_email($email)]);
}
// Get All Records
function wp_crud_get_all() {
global $wpdb;
$table_name = $wpdb->prefix . 'custom_data';
return $wpdb->get_results("SELECT * FROM $table_name");
}
// Get Single Record
function wp_crud_get($id) {
global $wpdb;
$table_name = $wpdb->prefix . 'custom_data';
return $wpdb->get_row($wpdb->prepare("SELECT * FROM $table_name WHERE id = %d", $id));
}
// Update Record
function wp_crud_update($id, $name, $email) {
global $wpdb;
$table_name = $wpdb->prefix . 'custom_data';
return $wpdb->update($table_name, ['name' => sanitize_text_field($name), 'email' => sanitize_email($email)], ['id' => $id]);
}
// Delete Record
function wp_crud_delete($id) {
global $wpdb;
$table_name = $wpdb->prefix . 'custom_data';
return $wpdb->delete($table_name, ['id' => $id]);
}
๐ Step 4: Create Admin Page
๐ Folder: /wp-content/plugins/wp-crud-plugin/includes/
๐ File: /wp-content/plugins/wp-crud-plugin/includes/admin-page.php
๐ Content:
<?php
// Add Admin Menu
function wp_crud_admin_menu() {
add_menu_page(
'Custom CRUD',
'Custom CRUD',
'manage_options',
'wp_crud',
'wp_crud_render_admin_page',
'dashicons-database',
25
);
}
add_action('admin_menu', 'wp_crud_admin_menu');
function wp_crud_render_admin_page() {
global $wpdb;
$table_name = $wpdb->prefix . 'custom_data';
// Handle Create & Update
if (isset($_POST['submit'])) {
if (!empty($_POST['id'])) {
wp_crud_update($_POST['id'], $_POST['name'], $_POST['email']);
} else {
wp_crud_insert($_POST['name'], $_POST['email']);
}
}
// Handle Delete
if (isset($_GET['delete'])) {
wp_crud_delete($_GET['delete']);
}
// Fetch Data
$results = wp_crud_get_all();
$edit_data = isset($_GET['edit']) ? wp_crud_get($_GET['edit']) : null;
?>
<div class="wrap">
<h1>Custom CRUD Operations</h1>
<form method="POST">
<input type="hidden" name="id" value="<?php echo $edit_data ? $edit_data->id : ''; ?>">
<input type="text" name="name" placeholder="Enter Name" value="<?php echo $edit_data ? esc_attr($edit_data->name) : ''; ?>" required>
<input type="email" name="email" placeholder="Enter Email" value="<?php echo $edit_data ? esc_attr($edit_data->email) : ''; ?>" required>
<?php if ($edit_data) : ?>
<input type="submit" name="submit" value="Update">
<a href="?page=wp_crud" class="button button-secondary">Cancel Update</a>
<?php else : ?>
<input type="submit" name="submit" value="Add Record">
<?php endif; ?>
</form>
<h2>Stored Data</h2>
<table class="wp-list-table widefat fixed striped">
<thead>
<tr>
<th>ID</th>
<th>Name</th>
<th>Email</th>
<th>Actions</th>
</tr>
</thead>
<tbody>
<?php foreach ($results as $row) : ?>
<tr>
<td><?php echo $row->id; ?></td>
<td><?php echo esc_html($row->name); ?></td>
<td><?php echo esc_html($row->email); ?></td>
<td>
<a href="?page=wp_crud&edit=<?php echo $row->id; ?>">Edit</a> |
<a href="?page=wp_crud&delete=<?php echo $row->id; ?>" onclick="return confirm('Delete this record?');">Delete</a>
</td>
</tr>
<?php endforeach; ?>
</tbody>
</table>
</div>
<?php
}
Step 5: Cleanup on Uninstall
Create uninstall.php
inside /wp-content/plugins/wp-crud-plugin/
:
๐ Folder: /wp-content/plugins/wp-crud-plugin/
๐ File: /wp-content/plugins/wp-crud-plugin
/uninstall.php
๐ Content:
<?php
if (!defined('WP_UNINSTALL_PLUGIN')) {
exit;
}
global $wpdb;
$table_name = $wpdb->prefix . 'custom_data';
$wpdb->query("DROP TABLE IF EXISTS $table_name");
Conclusion
๐ Step 6: Add CSS for Styling
๐ Folder: /wp-content/plugins/wp-crud-plugin/assets/
๐ File: /wp-content/plugins/wp-crud-plugin/assets/style.css
๐ Content:
input[type="text"], input[type="email"] {
padding: 5px;
width: 300px;
margin-right: 10px;
}
input[type="submit"] {
padding: 5px 10px;
background: #0073aa;
color: white;
border: none;
cursor: pointer;
}
โ Final Steps
Upload the
wp-crud-plugin
folder to/wp-content/plugins/
.Go to WordPress Admin > Plugins > Activate "Custom WP CRUD Plugin".
Access "Custom CRUD" from the sidebar.
๐ Your WordPress CRUD plugin is now fully functional! ๐
Subscribe to my newsletter
Read articles from Ogunuyo Ogheneruemu B directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
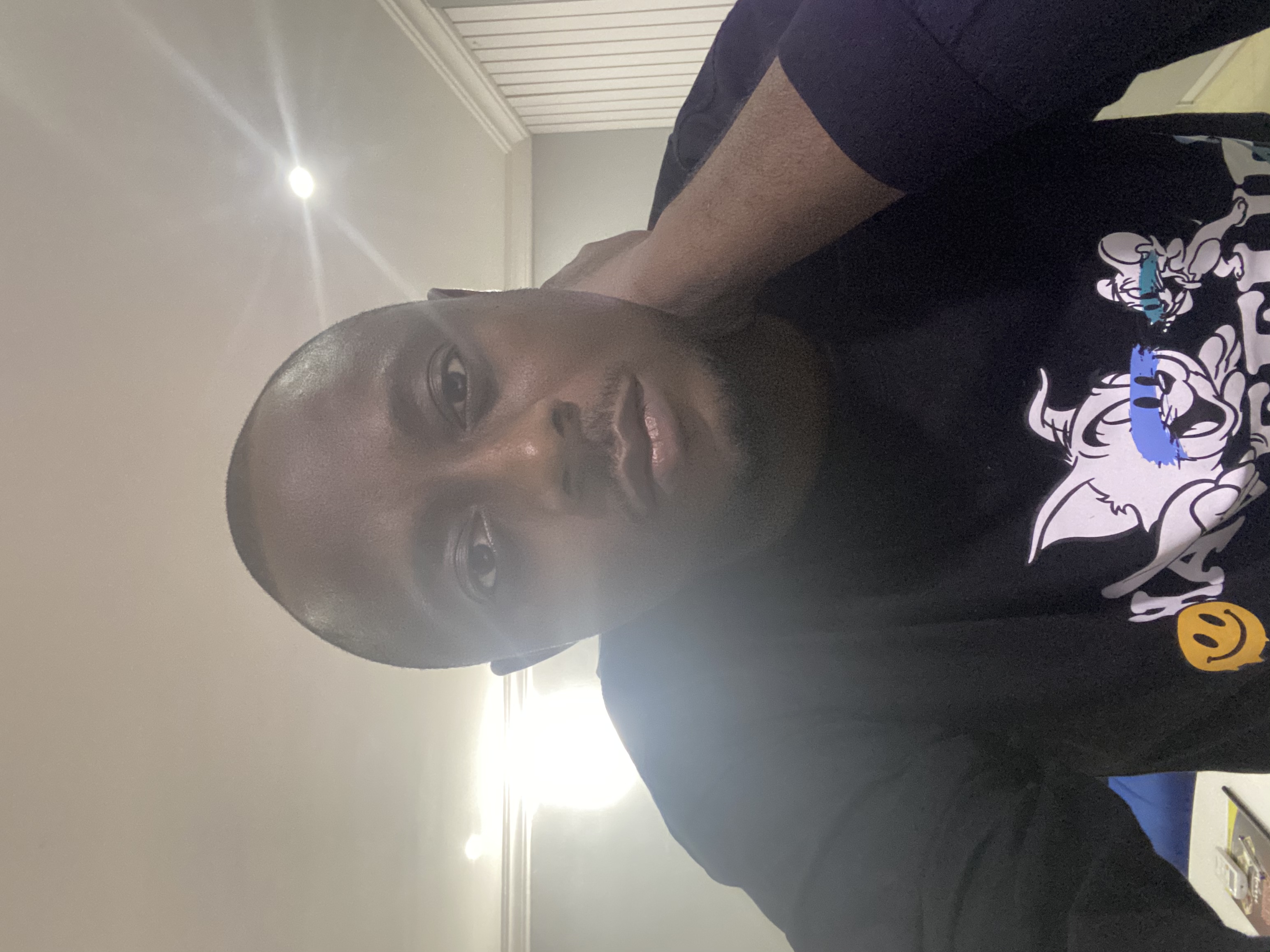
Ogunuyo Ogheneruemu B
Ogunuyo Ogheneruemu B
I'm Ogunuyo Ogheneruemu Brown, a senior software developer. I specialize in DApp apps, fintech solutions, nursing web apps, fitness platforms, and e-commerce systems. Throughout my career, I've delivered successful projects, showcasing strong technical skills and problem-solving abilities. I create secure and user-friendly fintech innovations. Outside work, I enjoy coding, swimming, and playing football. I'm an avid reader and fitness enthusiast. Music inspires me. I'm committed to continuous growth and creating impactful software solutions. Let's connect and collaborate to make a lasting impact in software development.