TypeScript Interface and Types
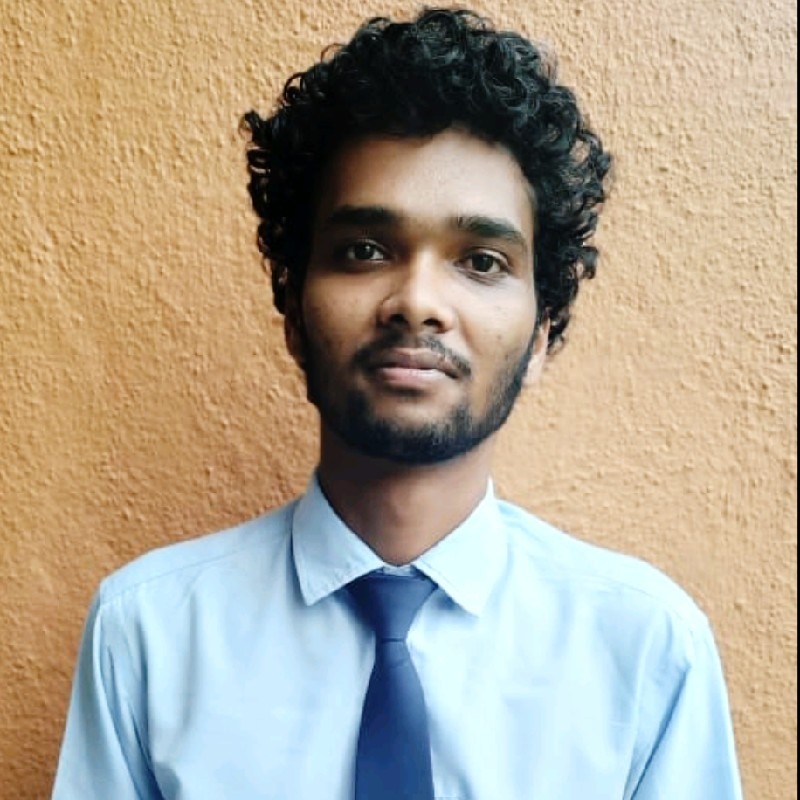
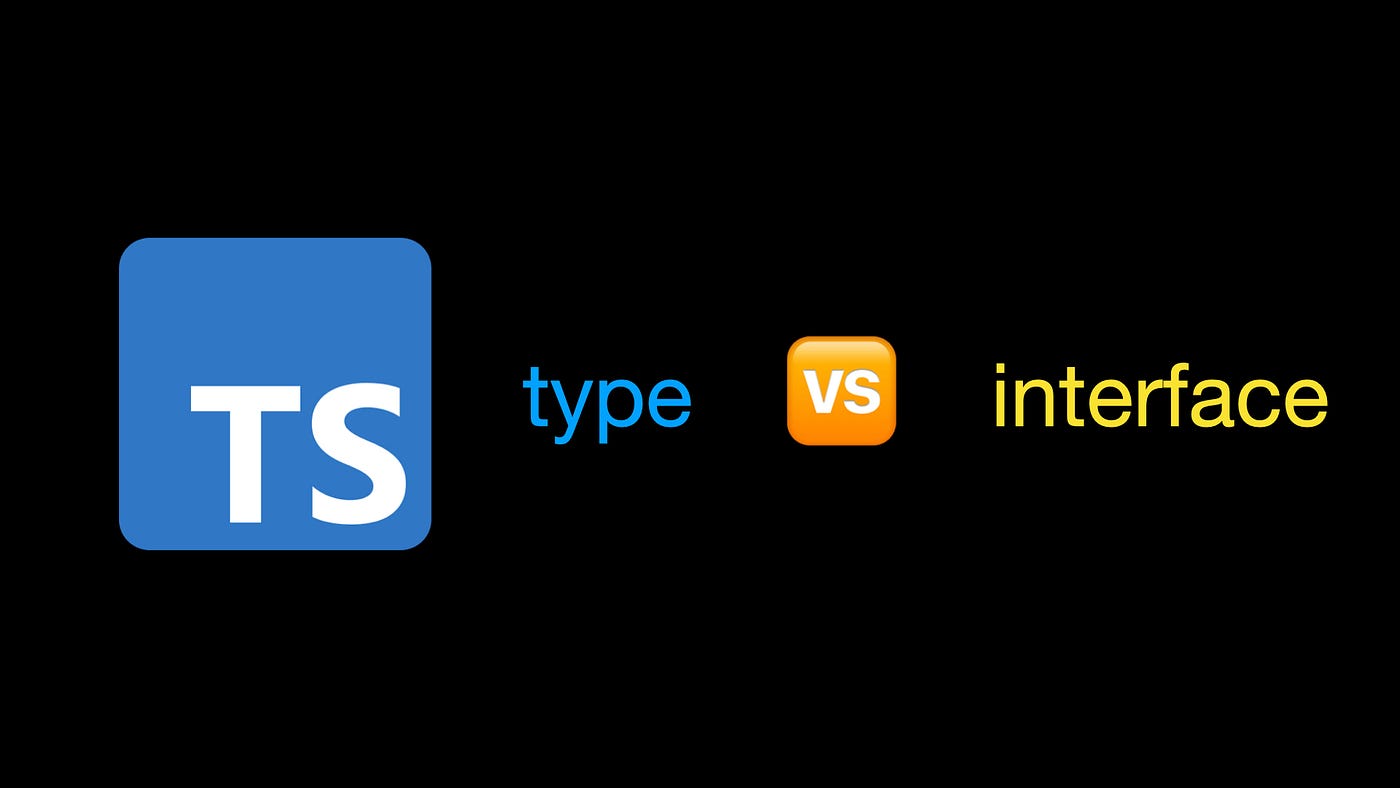
Introduction
Creating and using functions is a fundamental aspect of any programming language, and TypeScript is no different. Today, we are going to explore Interfaces and Types in TypeScript. While TypeScript shares a similar syntax with JavaScript, it introduces type safety and additional features that improve code maintainability and readability.
Interfaces in TypeScript
An interface in TypeScript is a syntactical contract that an entity must adhere to. It defines a standard structure that implementing classes or objects must follow. However, it does not contain any implementation details.
Key Features of Interfaces:
Defines the structure of an object.
Only contains property and method declarations, without implementations.
Can be implemented by classes or extended by other interfaces.
Example of an Interface:
interface User {
name: string;
age: number;
}
interface Manager {
department: string;
}
In the above example, we have two interfaces: User
and Manager
, defining the required properties.
Implementing Interfaces in Objects:
let employee: User = {
name: "John Doe",
age: 30
};
The employee
object strictly follows the User
interface, ensuring type safety.
Extending Interfaces:
Interfaces can be extended to create new interfaces with additional properties.
interface TeamLead extends User, Manager {}
let teamLead: TeamLead = {
name: "Alice",
age: 35,
department: "Engineering"
};
Here, TeamLead
extends both User
and Manager
, so any object following TeamLead
must include properties from both.
Types in TypeScript
The Type System in TypeScript describes the various types used in the language. These types can be categorized into three main sections:
1. Any Type
The any
type allows a variable to hold values of any type. While it provides flexibility, it negates TypeScript's type-checking benefits.
let randomValue: any = "Hello";
randomValue = 42;
randomValue = true;
2. Built-In Types
TypeScript includes built-in types such as:
string
number
boolean
null
undefined
void
Example:
let username: string = "Nehal";
let userAge: number = 24;
let isActive: boolean = true;
3. User-Defined Types
Apart from built-in types, TypeScript allows defining custom types using type
aliases.
Using Type Aliases:
type Employee = {
name: string;
age: number;
position: string;
};
let dev: Employee = {
name: "Nehal",
age: 24,
position: "Software Developer"
};
Combining Types (Union & Intersection):
- Union Types: A variable can hold multiple types.
let id: string | number;
id = 101;
id = "A102";
- Intersection Types: Combines multiple types.
type Manager = {
department: string;
};
type TeamLead = User & Manager;
let lead: TeamLead = {
name: "Sophia",
age: 40,
department: "IT"
};
Conclusion
Understanding Interfaces and Types in TypeScript is crucial for writing clean, maintainable, and scalable code.
Interfaces define object structure and can be extended or implemented.
Types allow flexibility in defining custom types, including unions and intersections.
By leveraging TypeScript’s powerful type system, developers can improve code quality and prevent potential runtime errors. Happy coding!
Subscribe to my newsletter
Read articles from Nehal Ingole directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
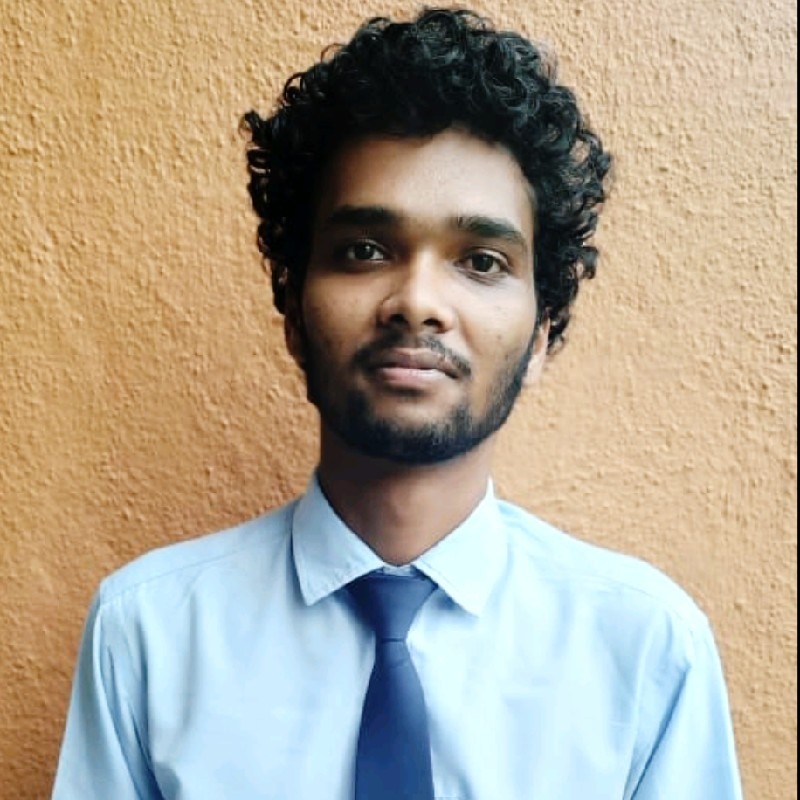
Nehal Ingole
Nehal Ingole
🚀 Greetings World! 🌐 Meet a dynamic Frontend Developer, UI/UX Designer, and avid explorer of Cloud & DevOps realms! Uncover the journey of a professional deeply passionate about crafting seamless user experiences, designing visually stunning interfaces, and navigating the cloud with a DevOps mindset. 🔧 Skills Snapshot: Frontend Mastery: HTML, CSS, and JavaScript expert, specializing in React, Angular, and Vue.js. Design Wizardry: Proficient in wireframing, prototyping, and Adobe Creative Suite and Figma for captivating designs. Cloud Maestro: Fluent in AWS, Azure, and Google Cloud Platform, adept at architecting scalable solutions. DevOps Guru: Skilled in Docker, Kubernetes, Jenkins, and Git, contributing to efficient development workflows. 🔗 Let's Connect: Open to collaborating on exciting projects and sharing industry insights, I invite connections for networking or discussions. Reach out for potential collaborations. 📧 Contact Me: GitHub: GitHub Profile Email: nehalingole2001@gmail.com Mobile: 7397966719 Figma: Figma Profile Twitter: Twitter Profile HashNode: HashNode Profile LinkedIn : LinkedIn Profile