Simple Guide to Setting Up OAuth Authentication in Next.js Apps
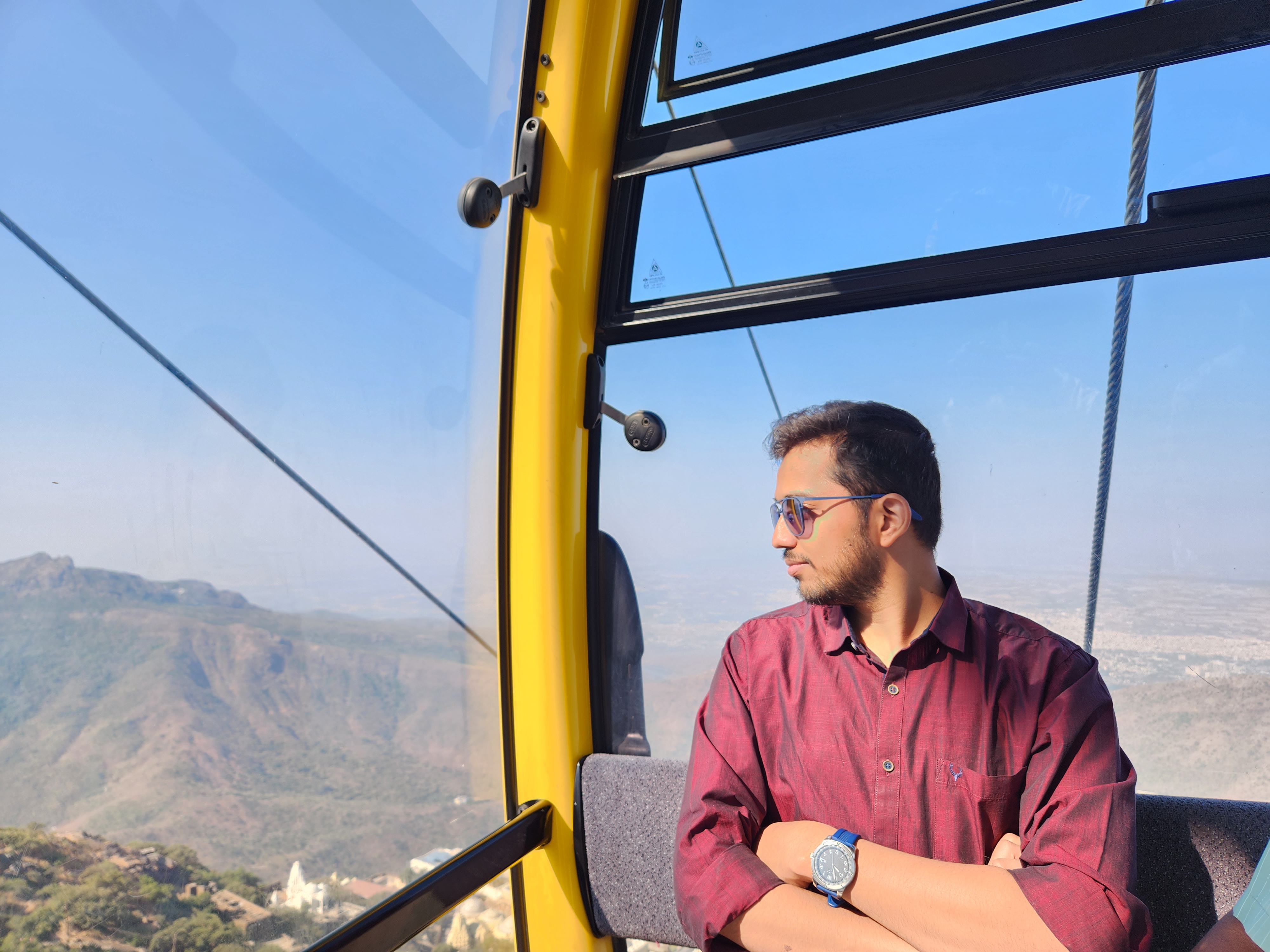

Authentication is a critical part of modern web applications, and OAuth has become the go-to standard for secure and scalable user authentication. If you're building a Next.js application and want to integrate OAuth, you're in the right place! In this blog, we'll walk through how to implement OAuth authentication in a Next.js app using the next-auth
library.
What is OAuth?
OAuth is an open standard for access delegation. It is commonly used to grant websites or applications access to user information without exposing their credentials. Platforms like Google, GitHub, and Facebook use it to allow users to log in to third-party apps securely.
Why Use Next.js for Authentication?
Next.js is a powerful React framework that supports server-side rendering (SSR), static site generation (SSG), and API routes. Its built-in features make it an excellent choice for implementing authentication, especially when combined with libraries like next-auth
.
Prerequisites
Before we dive in, make sure you have the following:
Basic knowledge of React and Next.js.
Node.js and npm/yarn installed.
A GitHub, Google, or any OAuth provider account (for testing).
Step 1: Setting Up a Next.js Project
Let’s start by creating a new Next.js project. By running the following command in our terminal:
npx create-next-app nextjs-oauth
cd nextjs-oauth
This will set up a new Next.js project. Once the setup is complete, we need to install the next-auth
library:
npm install next-auth
Step 2: Configure next-auth
Part 1: Register the App with an OAuth Provider
For this example, we’ll use GitHub as our OAuth provider. Follow these steps to get your clientId
and clientSecret
:
Click New OAuth App.
Fill in the required details (e.g., Application Name, Homepage URL, Authorization Callback URL).
After registering, you’ll receive a
clientId
andclientSecret
.
Part 2: Configure next-auth
Create a file called route.js
in the app/api/auth/[...nextauth]
directory:
import NextAuth from "next-auth";
import GitHubProvider from "next-auth/providers/github";
const handler = NextAuth({
providers: [
GitHubProvider({
clientId: process.env.GITHUB_CLIENT_ID,
clientSecret: process.env.GITHUB_CLIENT_SECRET,
}),
],
});
export { handler as GET, handler as POST };
Here, we’re using GitHub as our OAuth provider. You’ll need to register your application on GitHub to get the clientId
and clientSecret
.
Step 3: Setting Up Environment Variables
To keep sensitive information secure, we need to store our clientId
and clientSecret
in a .env.local
file:
GITHUB_CLIENT_ID=your_github_client_id
GITHUB_CLIENT_SECRET=your_github_client_secret
Also, we have to make sure that we add .env.local
to our .gitignore
file to avoid exposing our credentials.
Step 4: Adding Authentication to the UI
Now that next-auth
is configured, let’s add a login button to our application. So for that, we have to open the page.js
file in the app
directory and add the following code:
"use client";
import { useSession, signIn, signOut } from "next-auth/react";
export default function Home() {
const { data: session } = useSession();
return (
<div style={{ padding: "20px" }}>
{!session ? (
<>
<p>You are not signed in</p>
<button onClick={() => signIn("github")}>Sign in with GitHub</button>
</>
) : (
<>
<p>Welcome, {session.user.name}!</p>
<button onClick={() => signOut()}>Sign out</button>
</>
)}
</div>
);
}
This code checks if the user is logged in. If not, it displays a "Sign in with GitHub" button. Once logged in, it welcomes the user and provides a sign-out option.
Step 5:. Protecting Routes
To protect specific routes, we can use the getServerSession
function provided by next-auth
. For example, to protect a dashboard page, we can create a dashboard/page.js
file in the app
directory:
import { getServerSession } from "next-auth";
import { authOptions } from "@/app/api/auth/[...nextauth]/route";
import { redirect } from "next/navigation";
export default async function Dashboard() {
const session = await getServerSession(authOptions);
if (!session) {
redirect("/");
}
return (
<div>
<h1>Dashboard</h1>
<p>Welcome to dashboard, {session.user.name}!</p>
</div>
);
}
Step 6: Customizing the Authentication Flow
next-auth
allows us to customize the authentication flow. For example, we can:
Add multiple OAuth providers (e.g., Google, Facebook).
Customize the login page.
Use a database to store user sessions.
Here’s an example of adding Google as a provider:
import NextAuth from "next-auth";
import GitHubProvider from "next-auth/providers/github";
import GoogleProvider from "next-auth/providers/google";
const handler = NextAuth({
providers: [
GitHubProvider({
clientId: process.env.GITHUB_CLIENT_ID,
clientSecret: process.env.GITHUB_CLIENT_SECRET,
}),
GoogleProvider({
clientId: process.env.GOOGLE_CLIENT_ID,
clientSecret: process.env.GOOGLE_CLIENT_SECRET,
}),
],
});
export { handler as GET, handler as POST };
Step 7: Deploying the Application
Once our application is ready, we can deploy it to platforms like Vercel or Netlify. Also, we need to make sure to set the environment variables (clientId
and clientSecret
) in our production environment.
Conclusion
Congratulations! Now we have successfully implemented OAuth authentication in a Next.js application using the App Router and next-auth
. This setup provides a secure and scalable way to handle user authentication in our app.
If you want to explore further, check out the next-auth documentation for advanced features like database adapters, email authentication, and more.
Subscribe to my newsletter
Read articles from Anurag Singh directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
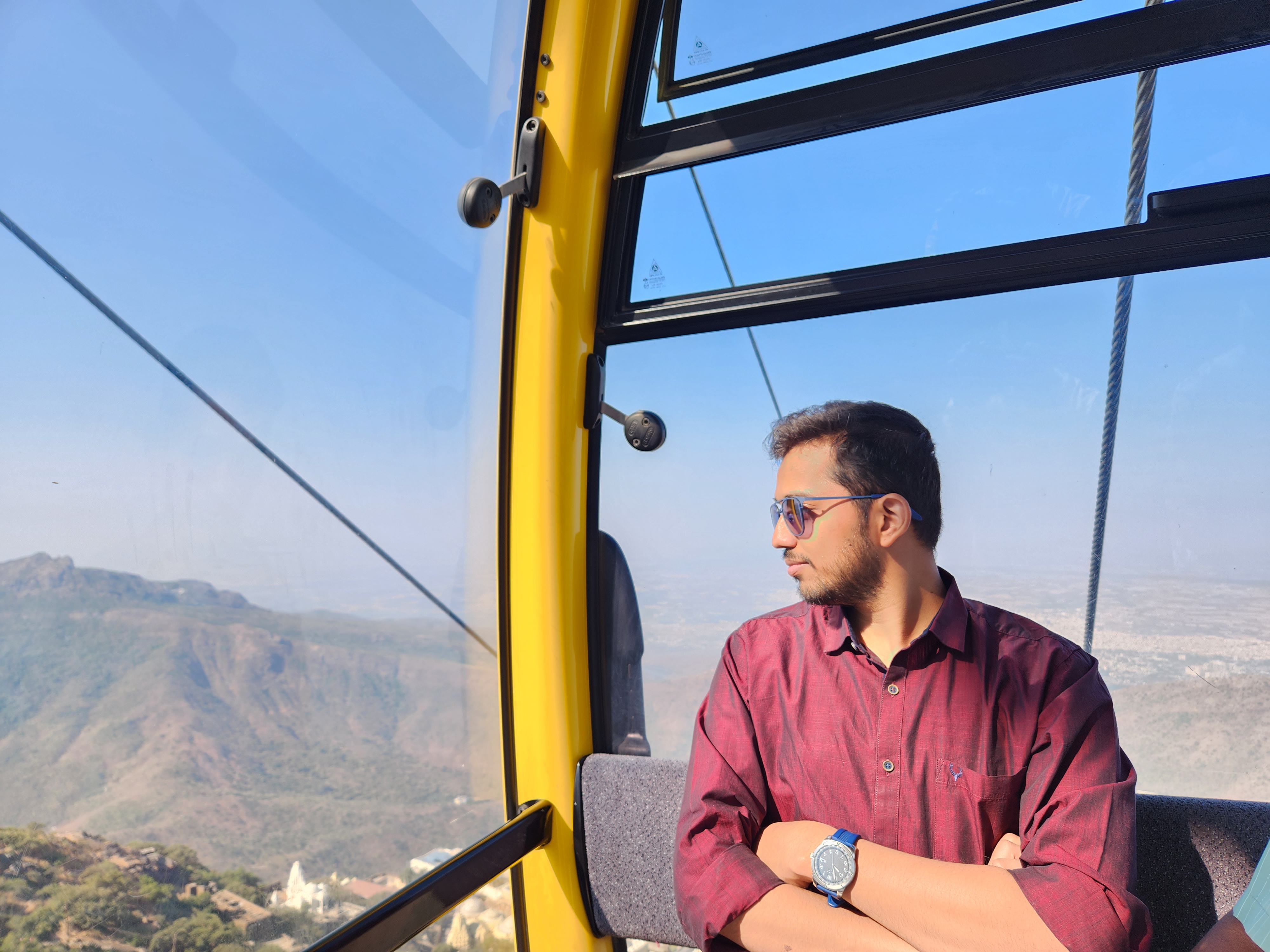
Anurag Singh
Anurag Singh
Software Engineer