The Role of WebAssembly (WASM) in Modern Frontend Applications ๐

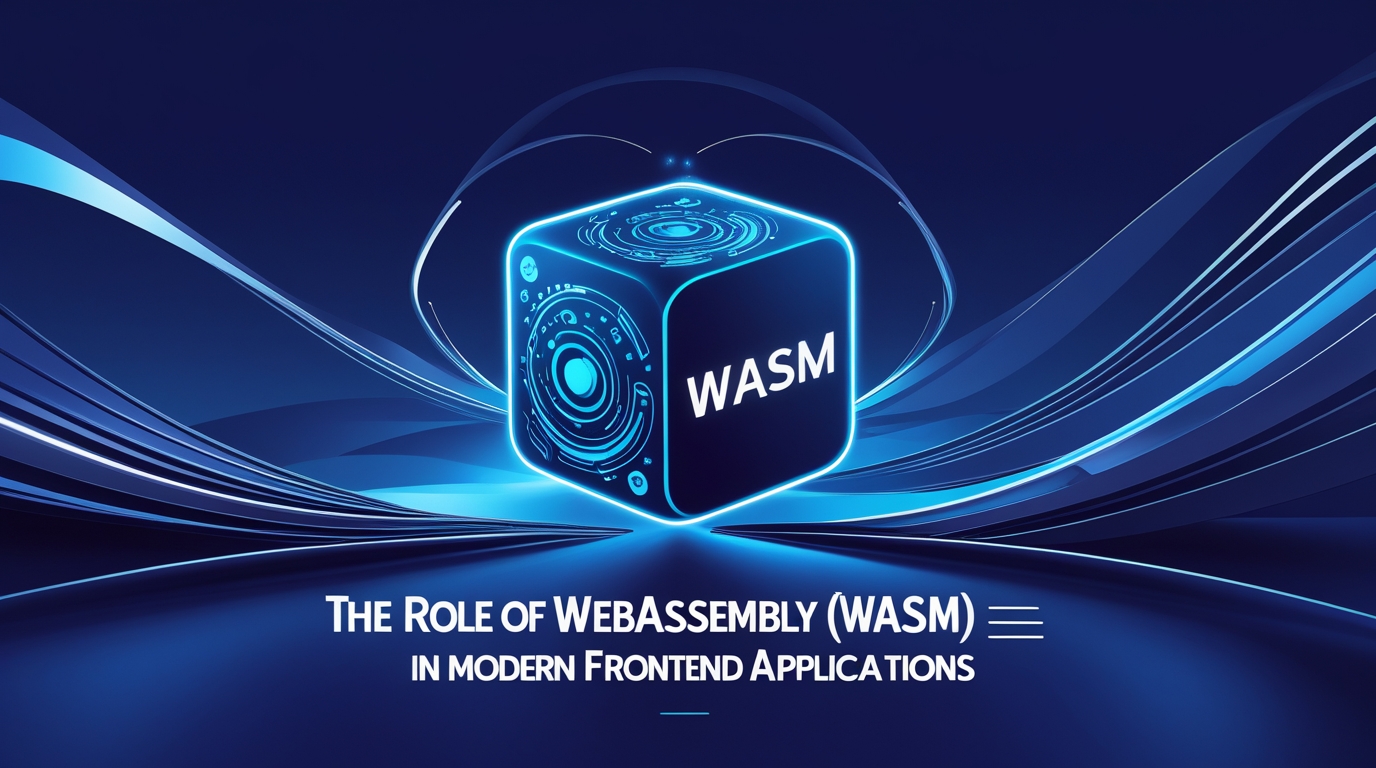
๐ Introduction
The web has come a long way in the past decade. JavaScript has been the king of web development, powering everything from simple websites to complex applications. However, JavaScript has its limitations, especially when dealing with high-performance tasks like gaming, video processing, and complex computations. This is where WebAssembly (WASM) comes in.
WebAssembly is a technology that allows developers to run code written in languages like C, C++, and Rust directly in the browser at near-native speed. In this article, weโll explore what WASM is, why it matters for frontend development, and how you can use it in real-world applications.
๐ What is WebAssembly (WASM)?
WebAssembly is a binary instruction format that runs in web browsers alongside JavaScript. It is designed to be fast, secure, and portable. Unlike JavaScript, which is interpreted, WebAssembly is compiled, meaning it runs much faster.
Key features of WASM:
โก Fast Execution: Runs at near-native speed.
๐ Language Support: Can be written in languages like Rust, C, and C++.
๐ Interoperability: Works alongside JavaScript, not as a replacement.
๐ Security: Runs in a sandboxed environment to ensure safety.
๐ Why Use WebAssembly in Frontend Development?
WASM is not meant to replace JavaScript but to complement it. Here are some key reasons developers use WASM in modern frontend applications:
1. ๐ Performance Boost
WebAssembly is much faster than JavaScript when handling heavy calculations. This makes it ideal for:
๐ฅ Video and image processing
๐ฎ 3D rendering in web games
๐ Cryptographic operations
๐ Example: Figma, a popular design tool, uses WebAssembly to speed up its graphics rendering.
2. ๐ Running Code from Other Languages
With WASM, developers can bring existing C++ or Rust code to the web without rewriting it in JavaScript.
๐ Example: Google Earthโs web version uses WebAssembly to run C++ code in the browser.
3. ๐ Improved Security
WebAssembly code runs in a sandboxed environment, making it less vulnerable to security threats like cross-site scripting (XSS) attacks.
4. ๐ Cross-Platform Compatibility
Since WASM runs in all modern browsers (Chrome, Firefox, Safari, Edge), it provides a consistent experience across different devices.
๐ How WebAssembly Works
Write Code in a Supported Language: You can write code in C, C++, Rust, or other supported languages.
Compile to WebAssembly: The code is compiled into a
.wasm
file.Load in JavaScript: The
.wasm
file is imported into a JavaScript application.Execute in the Browser: The WebAssembly code runs inside the browserโs virtual machine.
๐ Getting Started with WebAssembly
Hereโs a simple example using Rust and WebAssembly:
Step 1: Install Rust and WASM Tools
# Install Rust
curl --proto '=https' --tlsv1.2 -sSf https://sh.rustup.rs | sh
# Install WebAssembly tool
cargo install wasm-pack
Step 2: Create a Rust Function
Create a new Rust project and add the following function:
#[wasm_bindgen]
pub fn add(a: i32, b: i32) -> i32 {
a + b
}
Step 3: Compile to WebAssembly
wasm-pack build --target web
Step 4: Use in JavaScript
import init, { add } from './pkg/my_wasm_project.js';
async function run() {
await init();
console.log(add(5, 10)); // Output: 15
}
run();
This example shows how we can use Rust to perform calculations and then call it from JavaScript.
๐ Why is WebAssembly Trending? (Statistics & Resources)
๐ Performance Benchmark: For computationally intensive tasks, WASM often executes code faster than JavaScript
๐ Adoption: Major companies like Google, Microsoft, and Adobe are integrating WebAssembly into their web apps.
๐ฎ Gaming Industry: Unity WebGL uses WASM to improve browser-based game performance.
๐ Resources to Learn More:
๐ Real-World Applications of WebAssembly
๐ฎ 1. Gaming
WASM enables high-performance 3D games to run in the browser without lag. Unity and Unreal Engine now support WebAssembly for web-based games.
๐ฅ 2. Video Editing and Image Processing
Applications like Photoshop and Figma use WebAssembly to handle image rendering efficiently in the browser.
๐ค 3. AI and Machine Learning
Libraries like TensorFlow.js leverage WebAssembly for faster AI model execution in the browser.
๐ 4. Blockchain and Cryptography
Crypto wallets and blockchain applications use WebAssembly for secure and fast cryptographic computations.
๐ WebAssembly vs. JavaScript: When to Use What?
Feature | JavaScript | WebAssembly |
โก Performance | Slower | Near-native |
๐ป Language | Only JavaScript | Rust, C, C++ |
๐ Security | Prone to XSS | Sandboxed |
๐ฏ Use Case | General-purpose | Heavy computation |
โ Use JavaScript for:
DOM manipulation
UI interactions
General web development
โ Use WebAssembly for:
Performance-intensive tasks
Running existing C++/Rust code in the browser
๐ฎ The Future
WebAssembly is continuously evolving. In the coming years, we can expect:
๐ Better Integration with JavaScript Frameworks like React and Vue.
๐ WASI (WebAssembly System Interface) for running WASM outside the browser.
๐ข Support for More Programming Languages.
๐ฏ Finally
WebAssembly is changing the way we think about frontend development. It allows developers to bring high-performance code to the web, making applications faster and more efficient. While it wonโt replace JavaScript, itโs a powerful tool for specific use cases like gaming, AI, and cryptography.
If youโre a frontend developer, now is the perfect time to explore WebAssembly and see how it can improve your projects. ๐
Subscribe to my newsletter
Read articles from sashen fernando directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

sashen fernando
sashen fernando
Full-Stack Developer