🌟 Is No-Build Even a Possibility in Today’s Complex JavaScript Ecosystem?
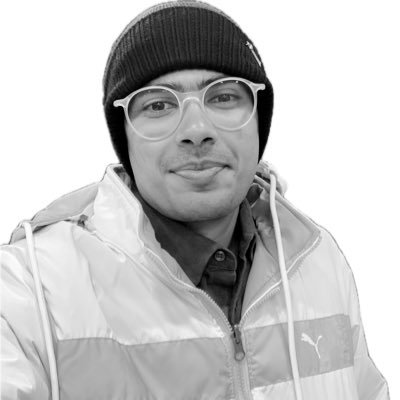

The JavaScript world today is often synonymous with complex build processes. Webpack, Babel, PostCSS, TypeScript—it feels like every new project comes bundled with a monstrous configuration file. As much as these tools have improved our workflow and application performance, they’ve also added significant complexity. Is there another way? Can we go back to simpler, no-build setups?
The answer is YES! And believe it or not, some of the most successful modern web applications are doing just that. HEY.com, for instance, a premium email service from Basecamp making millions of dollars in revenue, follows a no-build philosophy—shipping raw JavaScript and CSS files without minification, bundling, or transpiling.
Let’s explore how this works, what makes no-build an attractive choice, and how you can implement it for your own projects.
🌐 What Is No-Build?
The no-build approach is exactly what it sounds like: No bundling, no transpiling, no complex build pipelines. You write your JavaScript, CSS, and HTML as-is, and your browser consumes it without any intermediate steps.
Imagine this: You edit your code, save, and refresh. That’s it. No waiting for Webpack to finish. No mysterious build errors. Instant feedback. 🔄
<script type="module">
import { greet } from './utils.js';
greet('Welcome to the No-Build Era!');
</script>
This is how we used to write JavaScript before the era of massive build processes. What’s changed? Thanks to native ES Modules, CSS custom properties, and modern JavaScript APIs, no-build is making a comeback, and it’s more powerful than ever.
🏢 Real-World Example: HEY.com 🚀
HEY.com is a great example of a no-build success story. Their engineering team intentionally avoids the complexity of modern build tools. They ship their JavaScript without bundling or minifying it, meaning any developer can open the browser’s developer console and see the original JavaScript source code.
Go ahead, visit https://hey.com, open the console, and you’ll see it yourself! No Webpack bundles, no source maps—just clean, readable JavaScript.
Why would they do this?
Simplicity: Fewer moving parts mean fewer bugs.
Instant Iteration: Faster feedback loops for development.
Maintainability: Code is easier to understand and debug.
Developer Happiness: No spending hours tweaking build configurations! 🎉
This proves that no-build isn’t just for small side projects. It can work for full-blown production apps.
🛠️ No-Build with Lit and Web Components
If you’re thinking, “Okay, this sounds cool, but how do I actually implement it?”, let’s look at Lit (from lit.dev), a library for building web components with zero build tools required.
Building a Simple Component with Lit (No Build Required) 💡
import { LitElement, html, css } from 'https://unpkg.com/lit?module';
class MyComponent extends LitElement {
static styles = css`
:host {
display: block;
background: #f4f4f4;
padding: 1rem;
border-radius: 8px;
}
`;
static properties = {
message: { type: String },
};
constructor() {
super();
this.message = 'Hello, no-build world!';
}
render() {
return html`<p>${this.message}</p>`;
}
}
customElements.define('my-component', MyComponent);
All you need is native ES modules and Lit from a CDN, and you’re ready to go. No bundlers, no build process—just drop this in your HTML, and it works.
<!DOCTYPE html>
<html lang="en">
<head>
<script type="module" src="my-component.js"></script>
</head>
<body>
<my-component></my-component>
</body>
</html>
🛡️ But What About TypeScript? Use JSDoc for Typing!
Many developers feel tied to TypeScript, but did you know you can achieve most of the benefits of TypeScript with plain JavaScript and JSDoc? No build step required!
/**
* @param {string} name - The name to greet.
* @returns {string} A greeting message.
*/
function greet(name) {
return `Hello, ${name}!`;
}
Modern editors like VSCode provide full autocompletion and type-checking with just JSDoc annotations. This means you get the safety of types without the complexity of a TypeScript build step.
🔧 More Tools for No-Build Setups
Here are a few more tools that help you build with a no-build mindset:
Deno 🦕: A modern runtime for JavaScript and TypeScript—no bundlers or package managers needed.
deno run https://deno.land/std/examples/welcome.ts
ES Modules in Browsers: Native support for
import
/export
is now available in all modern browsers. Use it!Vite 🌞: While not entirely no-build, Vite offers a lightning-fast dev server with minimal bundling for production.
npm create vite@latest
📈 When Does No-Build Make Sense?
No-build isn’t for everyone, but it works surprisingly well in many scenarios:
Simple Websites and Prototypes: Perfect for quick experiments or small projects.
Internal Tools: Why over-engineer when you can keep it simple?
Modern Web Apps with Web Components: Leverage Lit and native browser APIs for powerful no-build solutions.
⚖️ Trade-Offs and Challenges
Of course, no-build setups come with trade-offs:
Limited Compatibility: Older browsers may require polyfills.
No Advanced Optimization: Minification and tree-shaking are missing.
Scaling Issues: For large apps, you may still want bundlers to manage complex dependencies.
But… for many projects, the simplicity outweighs these concerns.
🎯 Final Thoughts
The JavaScript ecosystem is full of tools that promise to make development easier—but often add unnecessary complexity. No-build is a refreshing alternative that forces you to focus on the essentials.
If you’re tired of waiting for builds and want a simpler, more joyful developer experience, give no-build a try. With modern browser capabilities, Lit, and JSDoc, you can create powerful applications without the hassle of complicated build pipelines.
Are you ready to ditch the build step? Give it a try and see how much fun coding can be again! 💻✨
Subscribe to my newsletter
Read articles from Vivek directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
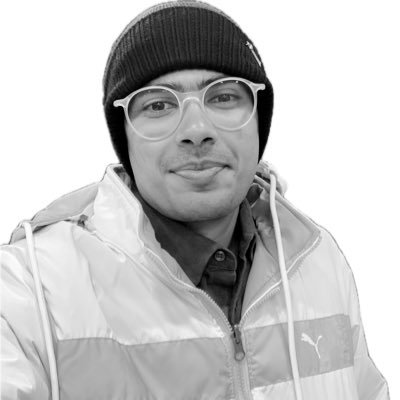
Vivek
Vivek
Curious Full Stack Developer wanting to try hands on ⌨️ new technologies and frameworks. More leaning towards React these days - Next, Blitz, Remix 👨🏻💻