Self-Executing Functions
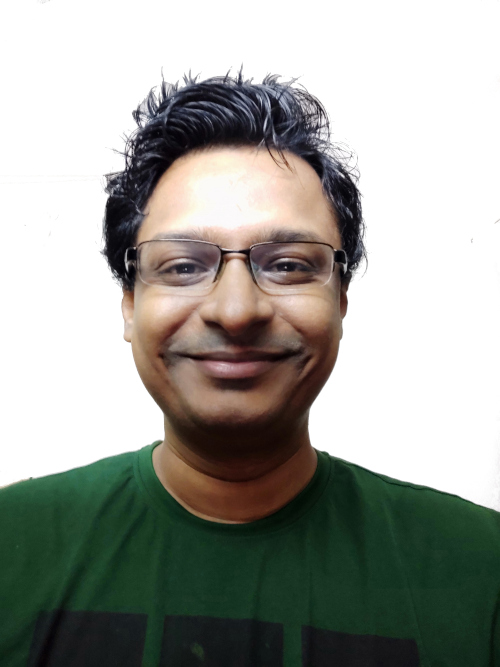
Let's say you wanted to call a function using await
(let's call this function get_usd2inr
) by calling it as in like - await get_usd2inr();
, you may get this error:
Uncaught SyntaxError: await is only valid in async functions and the top level bodies of modules (at main.js:15:1)
We solve this using self-executing functions - ()();
with async
.
let xe = 0;
const get_usd2inr = async () =>
{
const url = "https://open.er-api.com/v6/latest/USD";
try
{
const response = await fetch(url);
const data = await response.json();
const usd2inr = Number(data.rates.INR.toFixed(2));
return usd2inr;
}
catch (error)
{
console.error('Error fetching exchange rate:', error);
throw error;
}
}
// self-executing function
(async () =>
{
xe = await get_usd2inr();
console.log(xe); // 86.97 as of 13th February 2025
}
)();
console.log(xe); // 0 - at this point of time, get_usd2inr() is not yet done executing
In case you prefer a server-side fetch of open.er-api.com if at all they start blocking CORS all of a sudden :
def exchangerate_usd2inr():
url = "https://open.er-api.com/v6/latest/USD"
response = requests.get(url)
usd2inr = response.json()['rates']['INR']
usd2inr = round(usd2inr, 2)
return usd2inr
If you want to look into an example using workers check out a future blog post on using Google’s Comlink.
Subscribe to my newsletter
Read articles from Anjanesh Lekshminarayanan directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
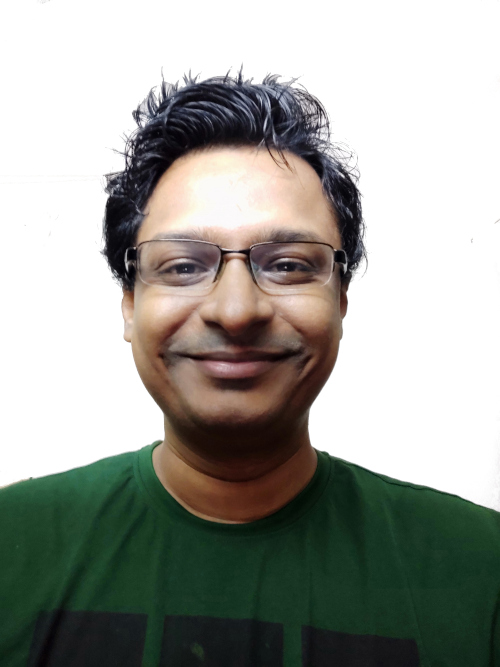
Anjanesh Lekshminarayanan
Anjanesh Lekshminarayanan
I am a web developer from Navi Mumbai. Mainly dealt with LAMP stack, now into Django and getting into Laravel and Google Cloud. TensorFlow in the near future. Founder of nerul.in and gaali.in