Auth.js in a nextjs app
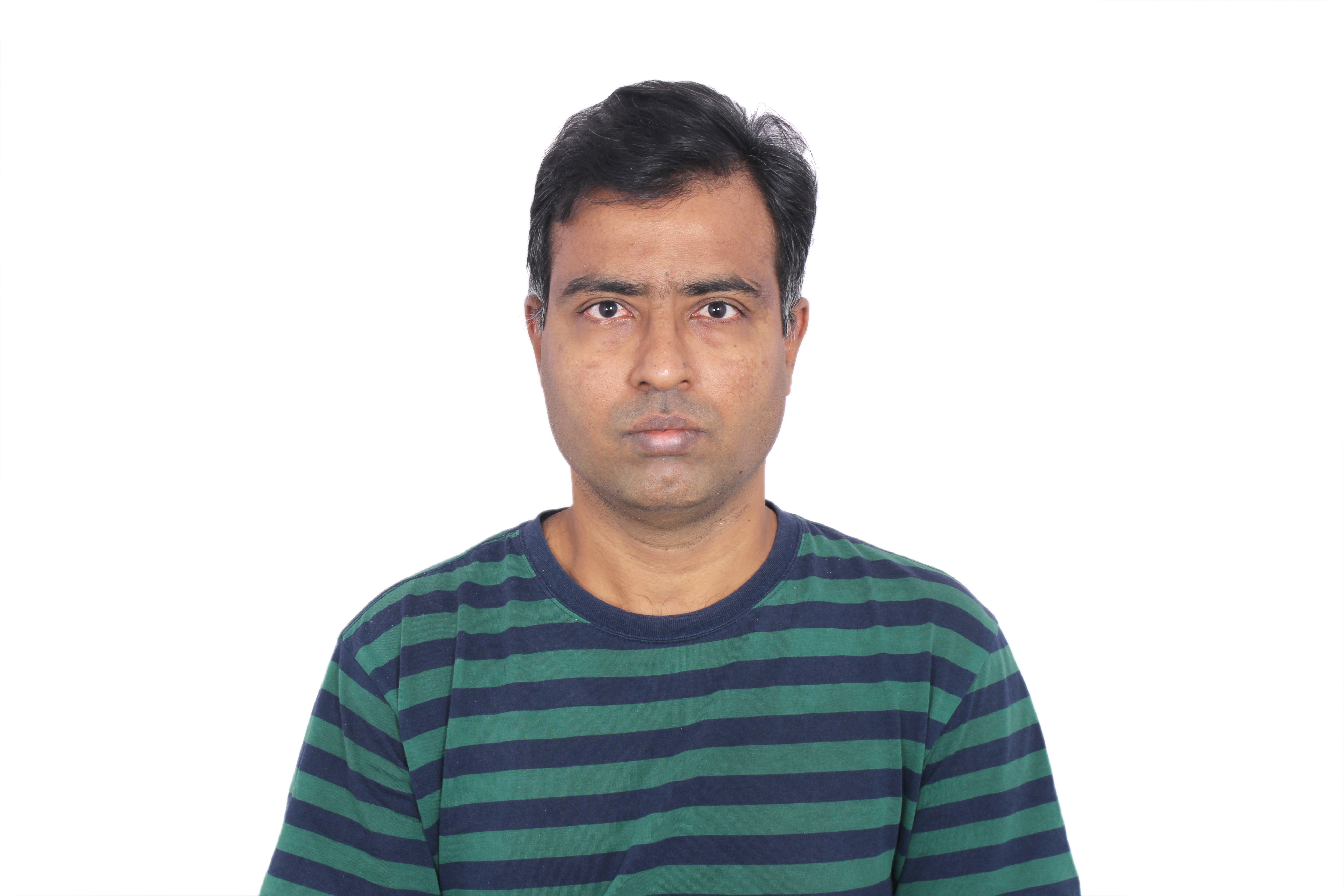

Auth.js is a popular framework for authentication in a NextJS app. The basic configuration is shown below
import NextAuth from "next-auth"
import { PrismaAdapter } from "@auth/prisma-adapter"
import { prisma } from "@/prisma"
import Google from "next-auth/providers/google"
export const { handlers, auth, signIn, signOut } = NextAuth({
adapter: PrismaAdapter(prisma),
providers: [Google],
})
There can be multiple oAuth providers. In this case, we have only one - Google. It is also possible to configure Email or magic links as a provider.
We should also provide an adapter. This is how Auth.js stores information about authenticated users. In this case, we are using a Prisma adapter. Prisma is an ORM that works with a wide variety of databases.
Regarding the return object from NextAuth call, there are four useful properties. signIn and signOut are fairly straight-forward. When the login button is clicked, do a signIn(‘google’)
and when the logout button is clicked, do a signOut()
The handlers property implements the callback method for oAuth provider (/api/auth/google/callback).
The auth property is interesting. On the server, if you want to get the session, simply use
import { auth } from "@/lib/auth"
const session = await auth()
This will give the user object if authenticated or null if unauthenticated.
In the client side (components), we get the session using
import { useSession } from "next-auth/react"
const session = useSession()
Hope this article on nextjs was helpful.
Subscribe to my newsletter
Read articles from Vijay Thirugnanam directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
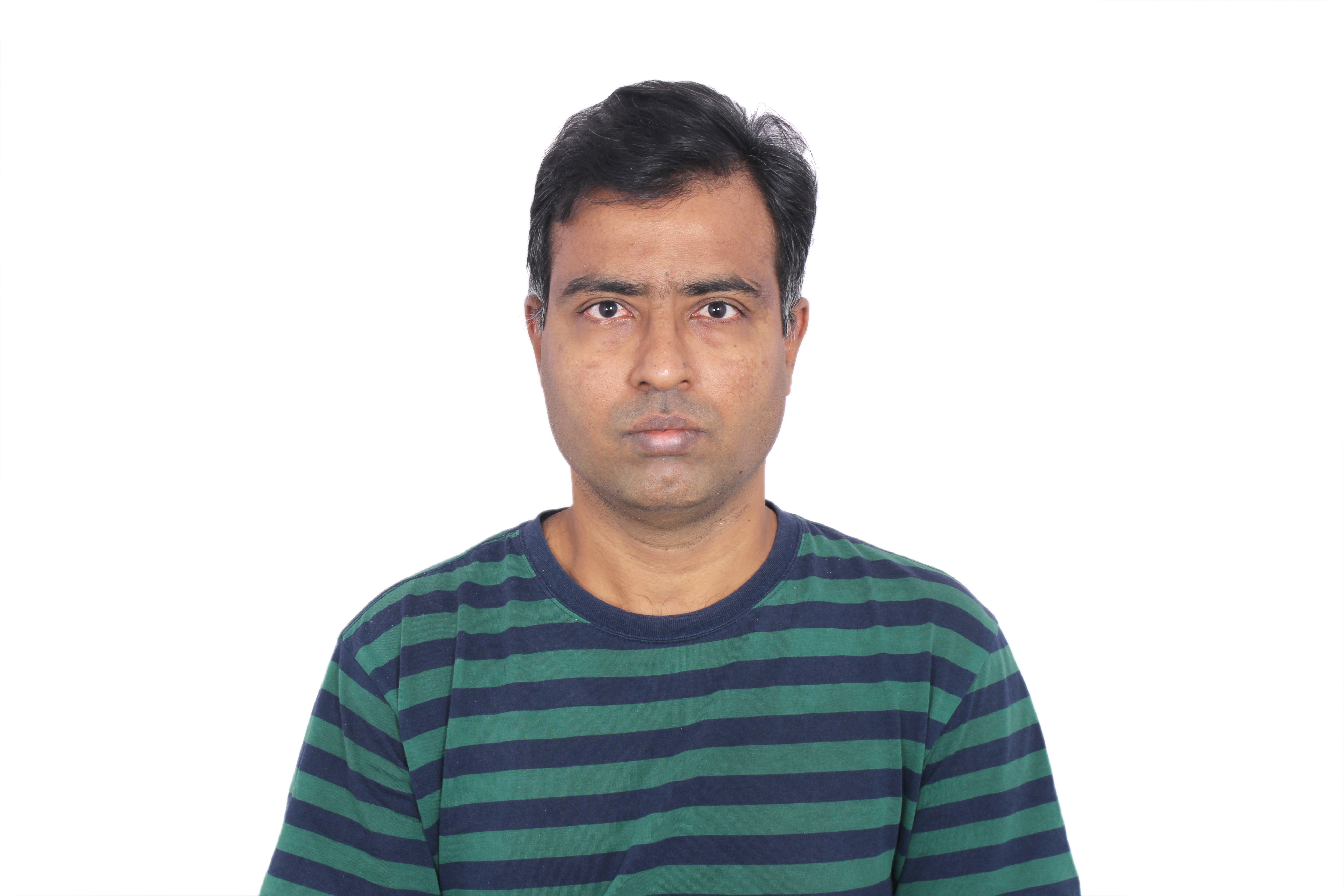
Vijay Thirugnanam
Vijay Thirugnanam
Engineer working in Cerebras. Latest work includes Cerebras Inference. I love writing code in React, TypeScript, C++, Python. I spend most of my work hours tinkering with code.