Building My Dream Cricket Team with JavaScript Array Methods
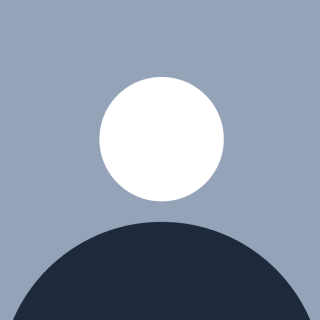
Imagine you are the selector of an international cricket team. You have the power to build the ultimate squad, make last-minute changes, and analyze player stats—all using JavaScript! Sounds exciting? Let’s explore how we can use some powerful array methods to craft our dream team.
The Team Selection Begins
It’s a bright morning, and you are finalizing the squad for an upcoming tournament. You start with a few key players.
1. push()
- Adding a Star Player
let team = ["Virat", "Rohit", "Bumrah"];
team.push("Dhoni");
console.log(team); // ["Virat", "Rohit", "Bumrah", "Dhoni"]
You decide to add the legendary Dhoni to the team. With push()
, he joins the squad at the last moment.
2. sort()
- Arranging Players Alphabetically
team.sort();
console.log(team); // ["Bumrah", "Dhoni", "Rohit", "Virat"]
To maintain order in the team list, you sort the players alphabetically.
Strengthening the Batting Lineup
You want to add an experienced opener at the top of the order.
3. concat()
- Merging Additional Players
let newPlayers = ["Sachin", "Sehwag"];
let fullTeam = team.concat(newPlayers);
console.log(fullTeam); // ["Bumrah", "Dhoni", "Rohit", "Virat", "Sachin", "Sehwag"]
You bring in more legends to strengthen the squad.
4. reverse()
- Changing the Batting Order
fullTeam.reverse();
console.log(fullTeam); // ["Sehwag", "Sachin", "Virat", "Rohit", "Dhoni", "Bumrah"]
To shake things up, you reverse the batting order.
Strategic Adjustments
As the tournament nears, some changes are needed.
5. some()
- Checking if the Team Has Any Bowlers
let hasBowler = fullTeam.some(player => player === "Bumrah");
console.log(hasBowler); // true
You verify if the squad includes at least one bowler.
6. every()
- Ensuring All Players Are String Names
let allPlayersValid = fullTeam.every(player => typeof player === "string");
console.log(allPlayersValid); // true
You ensure that every entry in the list is a valid player name.
Data-Driven Selection
You analyze the squad to ensure balance.
7. indexOf()
- Finding a Player’s Position
let playerIndex = fullTeam.indexOf("Virat");
console.log(playerIndex); // 2
You locate where a key player is positioned in the lineup.
8. includes()
- Checking if a Specific Player is in the Squad
let isPlayerInTeam = fullTeam.includes("Sachin");
console.log(isPlayerInTeam); // true
You confirm whether Sachin is in the team.
9. join()
- Creating a Readable Team List
let teamString = fullTeam.join(", ");
console.log(teamString); // "Sehwag, Sachin, Virat, Rohit, Dhoni, Bumrah"
You create a well-formatted string to display the team lineup.
10. fill()
- Replacing the Team for a New Match
let newTeam = new Array(5).fill("New Player");
console.log(newTeam); // ["New Player", "New Player", "New Player", "New Player", "New Player"]
Before the next game, you reset the squad with new players.
Final Thoughts
Managing a cricket team is a lot like coding—strategic, analytical, and fun! JavaScript arrays provide the perfect tools to select, modify, and analyze data dynamically. Whether you’re managing a sports team or handling complex datasets, these methods are invaluable.
Which of these methods do you find most useful? Share your thoughts in the comments below!
Subscribe to my newsletter
Read articles from Rahul Kumar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by