Optimizing Node.js Applications for Performance and Scalability
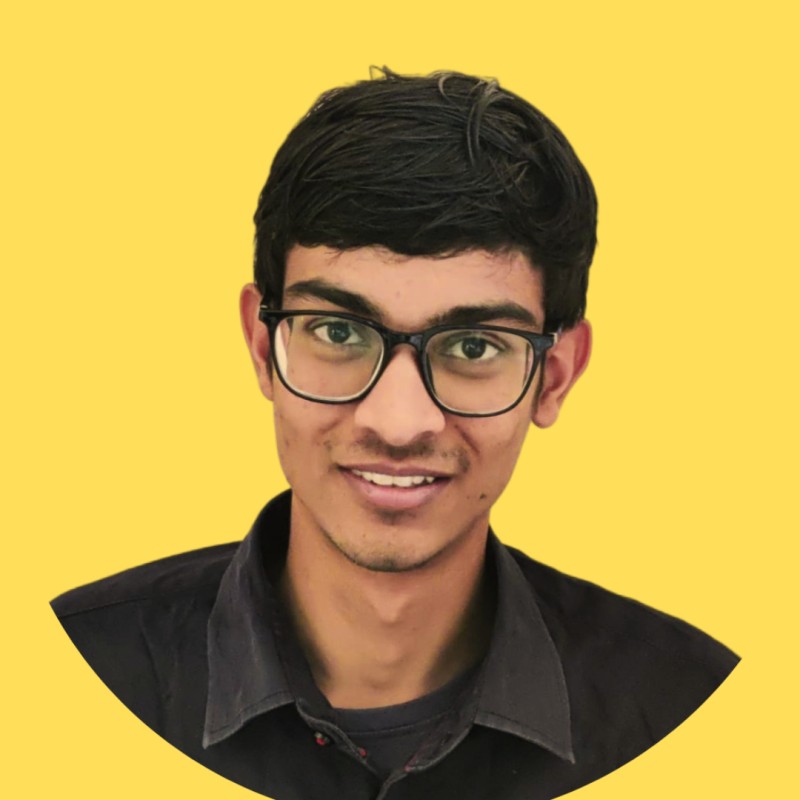
Table of contents
- Strategies to Handle High Traffic and Improve Efficiency
- Implement Smart Caching Techniques
- Offload Non-Critical Tasks to Background Processes
- Avoid Holding State in Memory
- Optimizing Node.js APIs with Timeouts and Asynchronous Functions
- Harnessing Asynchronous Functions for Improved Performance
- Minimize Unnecessary Computations
- Follow the Single Responsibility Principle
- Final Thoughts

Strategies to Handle High Traffic and Improve Efficiency
To deliver a seamless user experience and maintain system reliability, it’s essential to build Node.js applications that are both robust and efficient. A well-optimized application can manage traffic spikes, reduce response times, and minimize resource consumption.
In this guide, we’ll explore key strategies for optimising the performance of Node.js applications by focusing on:
Caching mechanisms to speed up response times
Efficient resource management to avoid unnecessary computations
Handling session data effectively to scale effortlessly
By implementing these best practices, you can enhance the efficiency of your Node.js applications and ensure long-term scalability.
Implement Smart Caching Techniques
One of the most effective ways to improve performance is through caching. A Content Delivery Network (CDN) can speed up the delivery of static assets, but there are additional caching techniques that can optimize data retrieval across various layers of the application.
Multi-Level Caching
Caching should be implemented at multiple levels to minimize redundant processing:
Database Query Caching: Store frequent query results to avoid hitting the database repeatedly. Tools like
async-cache-dedupe
can manage query caching and invalidation.import redis from 'redis'; import { promisify } from 'util'; const client = redis.createClient(); const getAsync = promisify(client.get).bind(client); const setAsync = promisify(client.set).bind(client); async function getUser(id: string) { const cacheKey = `user:${id}`; const cachedUser = await getAsync(cacheKey); if (cachedUser) { return JSON.parse(cachedUser); } const user = await db.getUserById(id); // Fetch from DB await setAsync(cacheKey, JSON.stringify(user), 'EX', 3600); // Cache for 1 hour return user; }
Component-Level Caching: If a database query produces consistent output, the rendered component can also be cached.
import NodeCache from 'node-cache'; const cache = new NodeCache({ stdTTL: 300 }); function renderComponent(data) { const cacheKey = `component:${JSON.stringify(data)}`; const cachedComponent = cache.get(cacheKey); if (cachedComponent) { return cachedComponent; } const rendered = `<div>${data.name}</div>`; // Sample rendering cache.set(cacheKey, rendered); return rendered; }
Full Page Caching: If all individual components remain unchanged, the entire page can be cached for faster loading times.
import express from 'express'; import apicache from 'apicache'; const app = express(); const cache = apicache.middleware; app.get('/products', cache('5 minutes'), async (req, res) => { const products = await db.getAllProducts(); res.json(products); });
However, cache invalidation is crucial. If a database entry is updated, all dependent cached components must be invalidated to ensure data consistency.
Offload Non-Critical Tasks to Background Processes
Certain tasks do not need to be processed synchronously within a request cycle. Using message queues can offload these tasks, improving responsiveness and efficiency.
Common Use Cases for Background Processing:
Sending emails asynchronously to prevent slow API response times
Processing large datasets without blocking the main thread
Managing scheduled tasks such as report generation
import { Queue } from 'bullmq';
const emailQueue = new Queue('emailQueue');
async function sendEmail(emailData) {
await emailQueue.add('sendEmail', emailData);
}
import { Worker } from 'bullmq';
const worker = new Worker('emailQueue', async (job) => {
console.log(`Sending email to ${job.data.to}`);
// Email sending logic here
});
By utilizing message queues like mqemitter
, tasks can be handled as system resources allow, ensuring better reliability and reducing downtime risks.
Avoid Holding State in Memory
For applications running multiple processes or scaling across multiple servers, session state management is crucial. Storing session data in-memory can lead to inconsistencies and load balancing issues. Instead, consider:
Using Redis or Valkey to store session data persistently
import session from 'express-session'; import connectRedis from 'connect-redis'; import redis from 'redis'; const RedisStore = connectRedis(session); const redisClient = redis.createClient(); app.use(session({ store: new RedisStore({ client: redisClient }), secret: 'your-secret-key', resave: false, saveUninitialized: false, }));
Leveraging JWTs (JSON Web Tokens) to manage user authentication without requiring session storage
import jwt from 'jsonwebtoken'; const token = jwt.sign({ userId: 123 }, 'your-secret-key', { expiresIn: '1h' }); console.log(token);
Minimizing in-memory state ensures efficient memory usage, reducing garbage collection overhead and improving request processing speed.
Optimizing Node.js APIs with Timeouts and Asynchronous Functions
The Power of Timeouts in Node.js APIs
In the world of API optimization, timeouts are essential for managing user expectations and preventing indefinite wait times. Without a proper timeout mechanism, users may experience frustrating delays, leading to poor user experience and potential application failures.
Node.js provides built-in support for handling timeouts in HTTP requests, and libraries like Axios or Got make it easy to set a maximum waiting time for responses. If the API does not respond within the specified duration, a timeout error is triggered, allowing the application to handle failures gracefully.
Implementing timeouts ensures that your API does not keep waiting indefinitely for external services or database queries. Instead, it proactively communicates with users, maintaining reliability and responsiveness.
Here’s how you can enforce timeouts in a Node.js API using Axios:
typescriptCopyEditimport axios from "axios";
const fetchData = async () => {
try {
const response = await axios.get("https://api.example.com/data", {
timeout: 5000, // Set a 5-second timeout
});
console.log(response.data);
} catch (error) {
if (error.code === "ECONNABORTED") {
console.error("Request timed out. Please try again later.");
} else {
console.error("An error occurred:", error.message);
}
}
};
fetchData();
In this example, if the external API does not respond within 5 seconds, the request is aborted, preventing excessive waiting time. A proper error message is logged, ensuring a smoother user experience.
Harnessing Asynchronous Functions for Improved Performance
Asynchronous functions are a fundamental aspect of Node.js that enable non-blocking operations. In APIs that perform I/O-heavy tasks such as database queries, file processing, or external API calls, using async/await
allows the system to handle multiple requests concurrently without blocking the main event loop.
Think of asynchronous functions as a restaurant with multiple chefs. If only one chef handles all orders sequentially, customers must wait longer. However, with multiple chefs working in parallel, food is prepared faster, reducing wait times. Similarly, using asynchronous programming in Node.js APIs enhances performance and scalability.
Here’s how you can optimize a Node.js API using async functions:
typescriptCopyEditimport express from "express";
import { PrismaClient } from "@prisma/client";
const app = express();
const prisma = new PrismaClient();
// Example API endpoint with async function
app.get("/users", async (req, res) => {
try {
const users = await prisma.user.findMany(); // Fetch users from database
res.json(users);
} catch (error) {
console.error("Error fetching users:", error);
res.status(500).json({ message: "Internal Server Error" });
}
});
// Start server
app.listen(3000, () => {
console.log("Server is running on port 3000");
});
Here, the async
function ensures that the API remains responsive while fetching data from the database. The request is handled efficiently without blocking other incoming requests, improving overall API performance.
By combining timeouts and asynchronous functions, you can build highly optimized Node.js APIs that are both performant and user-friendly. 🚀
Minimize Unnecessary Computations
Unoptimized applications often perform redundant work. Identifying and eliminating unnecessary computations can significantly enhance efficiency.
Optimization Techniques:
Reduce redundant queries by caching responses
Optimize database indexing to speed up data retrieval
Avoid unnecessary service calls by restructuring data flows
// OPTIMIZE DATABASE QUERIES
await db.collection('users').createIndex({ email: 1 }, { unique: true });
For instance, rather than querying a database on every request, consider pre-fetching and caching frequently accessed data.
Follow the Single Responsibility Principle
Keeping functions and components small and focused makes applications easier to manage and optimize. When each function handles a specific task:
Performance bottlenecks become easier to identify
Code becomes easier to test and maintain
Execution paths are more predictable, reducing unexpected latency
class EmailService {
sendVerificationEmail(user) {
console.log(`Sending verification email to ${user.email}`);
}
}
Instead of writing complex multi-purpose functions, break them down into smaller, reusable building blocks.
Final Thoughts
Improving the efficiency of a Node.js application is an ongoing process. By leveraging caching, optimizing resource management, and structuring code for scalability, you can build high-performance applications that handle traffic spikes effectively.
Regularly monitoring and profiling your application can help identify bottlenecks and drive further optimizations. As your user base grows, continuously refine your strategies to maintain smooth and reliable performance.
Subscribe to my newsletter
Read articles from Sushil Kumar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
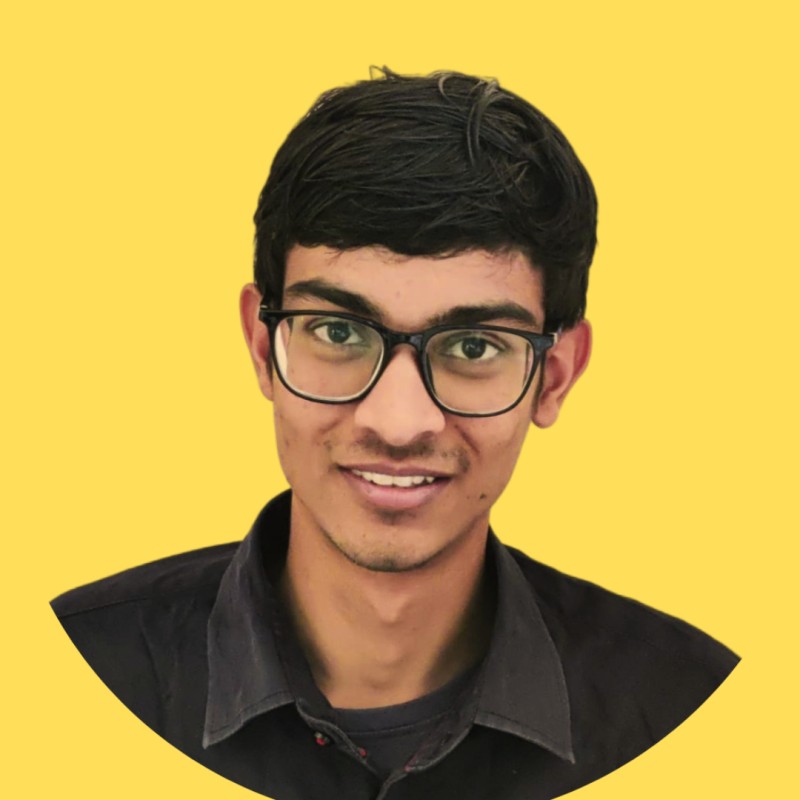
Sushil Kumar
Sushil Kumar
Hey devs, I am a Fullstack developer from India. Working for over 3 years now. I love working remote and exploring new realms of technology. Currently exploring AI and building scalable systems.