Securing Android WebView: Best Practices & Pitfalls to Avoid
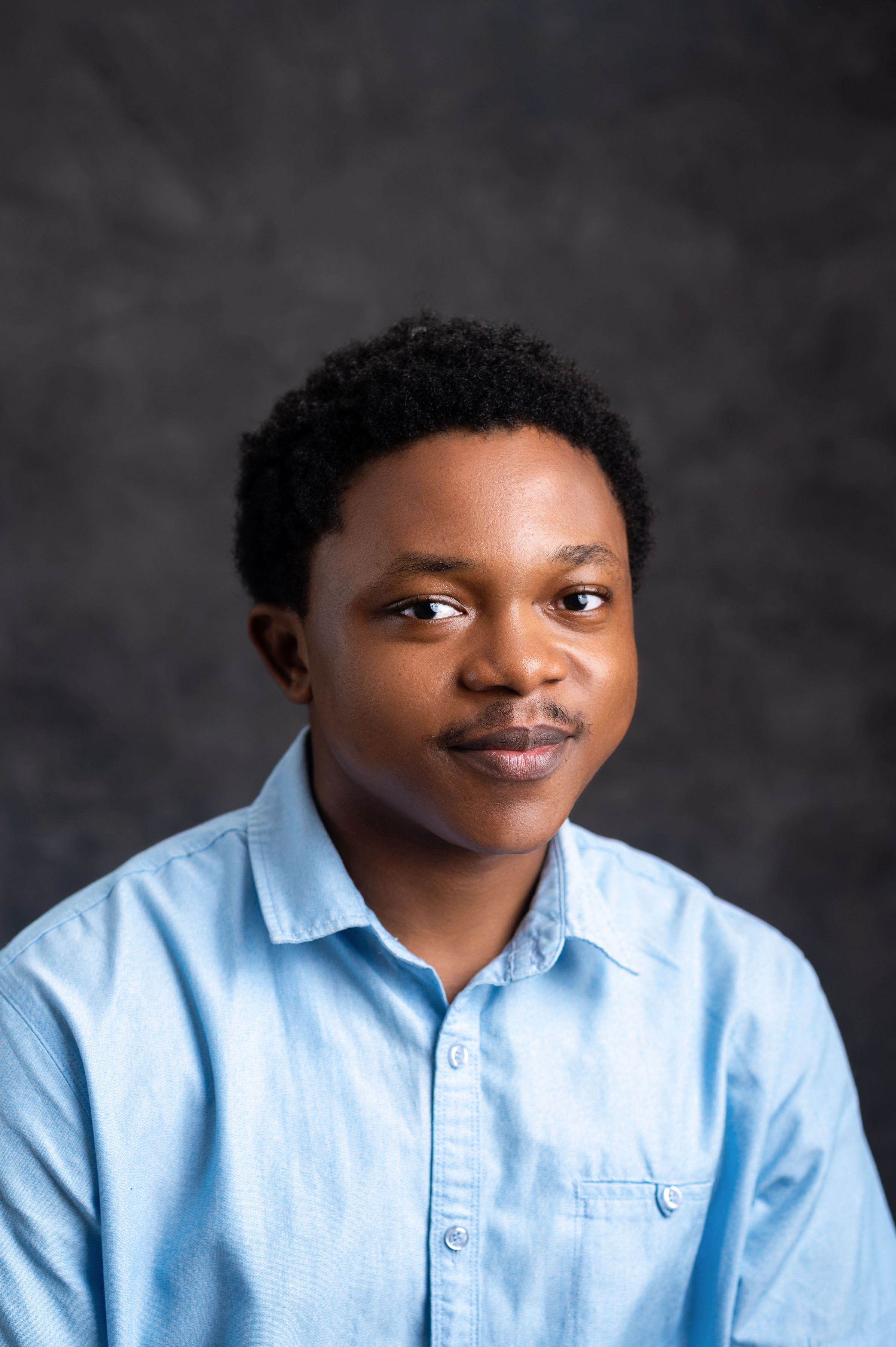

WebView is an indispensable tool for many Android apps, enabling developers to seamlessly embed web content into their native interfaces. However, its convenience comes with significant security challenges—from cross‐site scripting (XSS) attacks to unsafe JavaScript interfaces—that require careful handling. In this post, we’ll explore the key vulnerabilities associated with WebView and discuss concrete strategies for securing your apps.
Understanding the Risks
Common Vulnerabilities
XSS & Injection Attacks:
When JavaScript is enabled without proper controls, attackers can inject malicious scripts into your WebView. This can lead to stolen tokens or unauthorized actions.addJavascriptInterface Pitfalls:
Exposing native methods to JavaScript viaaddJavascriptInterface
can allow untrusted web content to invoke sensitive operations. This risk is amplified on devices below API level 21, where reflection could expose public fields.Local File Access Vulnerabilities:
Allowing file access or universal access from file URLs can expose your app’s internal resources. Disabling these settings is essential to prevent attackers from reading local files or launching file-based exploits.Man-in-the-Middle (MitM) & Insecure Communication:
Loading content over HTTP or failing to enforce SSL/TLS can leave your app vulnerable to MitM attacks. Secure communication is a must when integrating external web content.Unsafe File Access:
Allowing file or content access might let attackers read internal files.Insecure URL Loading:
Loading non-HTTPS URLs or failing to validate incoming URLs can lead to MitM attacks.
Best Practices
Disable Unnecessary Features:
Disable JavaScript, file access, and content access unless required.with(webView.settings) { javaScriptEnabled = false allowFileAccess = false allowContentAccess = false mixedContentMode = WebSettings.MIXED_CONTENT_NEVER_ALLOW }
Validate URLs:
Ensure that only HTTPS URLs are loaded:val secureUrl = if (Uri.parse(url).scheme.equals("https", ignoreCase = true)) url else { Log.e("SecureWebView", "Non-secure URL rejected: $url") return } webView.loadUrl(secureUrl)
Safe Browsing:
Enable Safe Browsing on supported devices (API 26+):if (Build.VERSION.SDK_INT >= Build.VERSION_CODES.O) { WebView.enableSafeBrowsing(context) { success -> Log.d("SecureWebView", "Safe Browsing enabled: $success") } }
Input Validation & CSP:
Always validate inputs that affect WebView content and, if possible, implement a Content Security Policy (CSP) on your server to limit resource loading.
Here's an example of a secure WebView embedded in a Compose UI:
@Composable
fun SecureWebView(url: String) {
// Validate that URL is secure (HTTPS) before loading
val secureUrl = if (Uri.parse(url).scheme.equals("https", ignoreCase = true)) url else {
Log.e("SecureWebView", "Non-secure URL rejected: $url")
return
}
AndroidView(factory = { context ->
WebView(context).apply {
settings.apply {
javaScriptEnabled = false // Disable JS if not needed
allowFileAccess = false // Disable file access
allowContentAccess = false // Disable content access
mixedContentMode = WebSettings.MIXED_CONTENT_NEVER_ALLOW
}
// Optionally, enable Safe Browsing on supported devices
if (Build.VERSION.SDK_INT >= Build.VERSION_CODES.O) {
WebView.enableSafeBrowsing(context) { success ->
Log.d("SecureWebView", "Safe Browsing enabled: $success")
}
}
loadUrl(secureUrl)
}
})
}
Additional Considerations
Input Validation: Always validate user inputs that affect WebView content.
SSL Pinning: Consider SSL pinning to secure communications further.
Content Security Policy (CSP): If you're loading HTML from your server, include CSP headers or meta tags to restrict resource loading.
By integrating these practices into your Compose-based app, you ensure that you maintain a high-security standard even when using AndroidView to host a WebView.
In summary, securing WebView in Jetpack Compose demands a proactive approach. By disabling unnecessary features, strictly validating URLs to enforce HTTPS, and enabling safe browsing, you minimize exposure to common vulnerabilities. Proper management of JavaScript interfaces and robust input validation further fortify your app against XSS and other injection attacks. Incorporating these best practices into your Compose-based UI not only protects your users but also ensures a more resilient application architecture. As mobile security threats continue to evolve, maintaining a vigilant, layered security strategy is essential for building trustworthy and robust Android applications.
Subscribe to my newsletter
Read articles from Daniel Alome directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
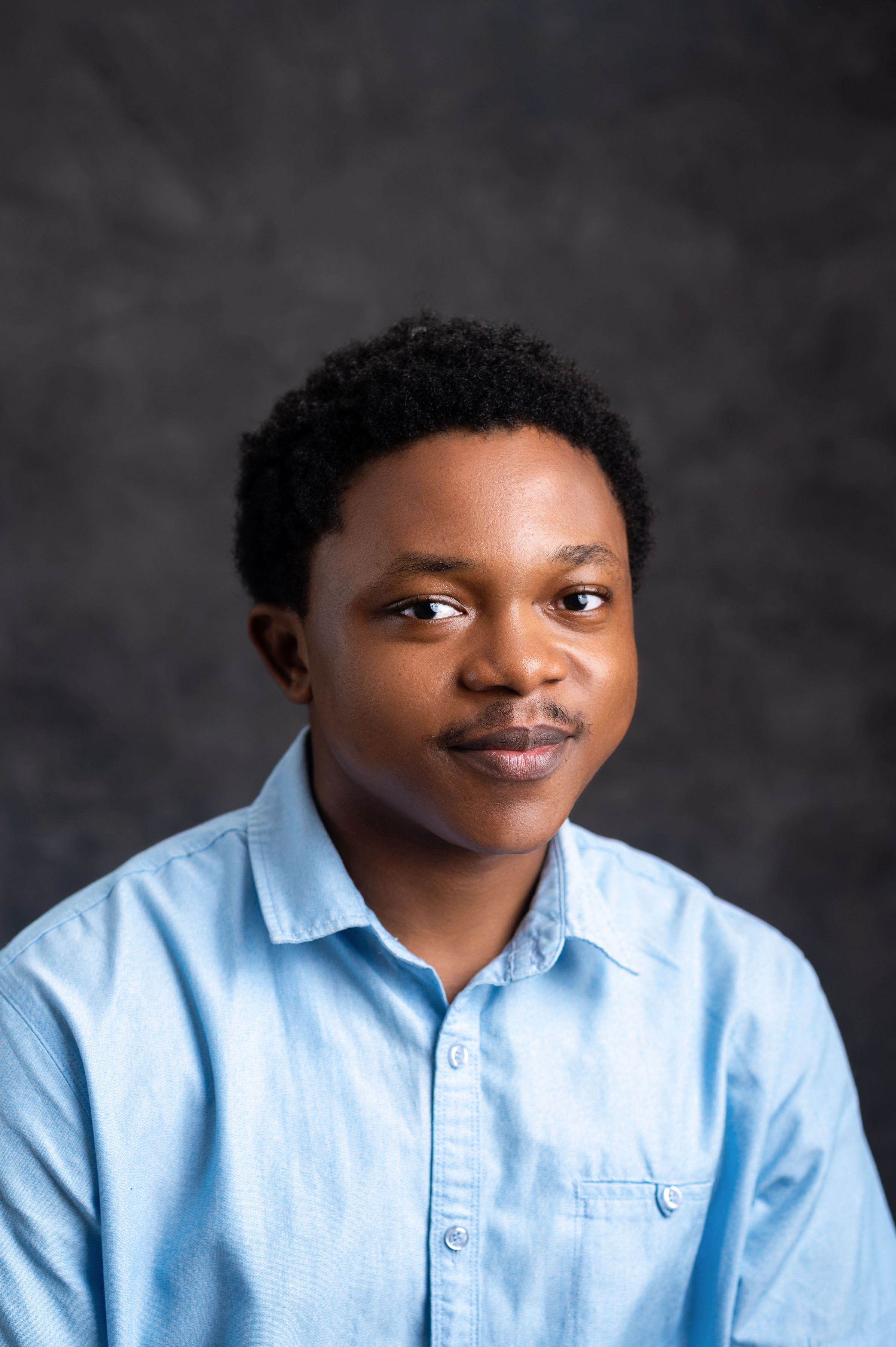
Daniel Alome
Daniel Alome
Software Engineer | IoT lover | Romans 8:38