Future-Proof Your Backend: The Expert’s Guide to Building a Rock-Solid System 🚀

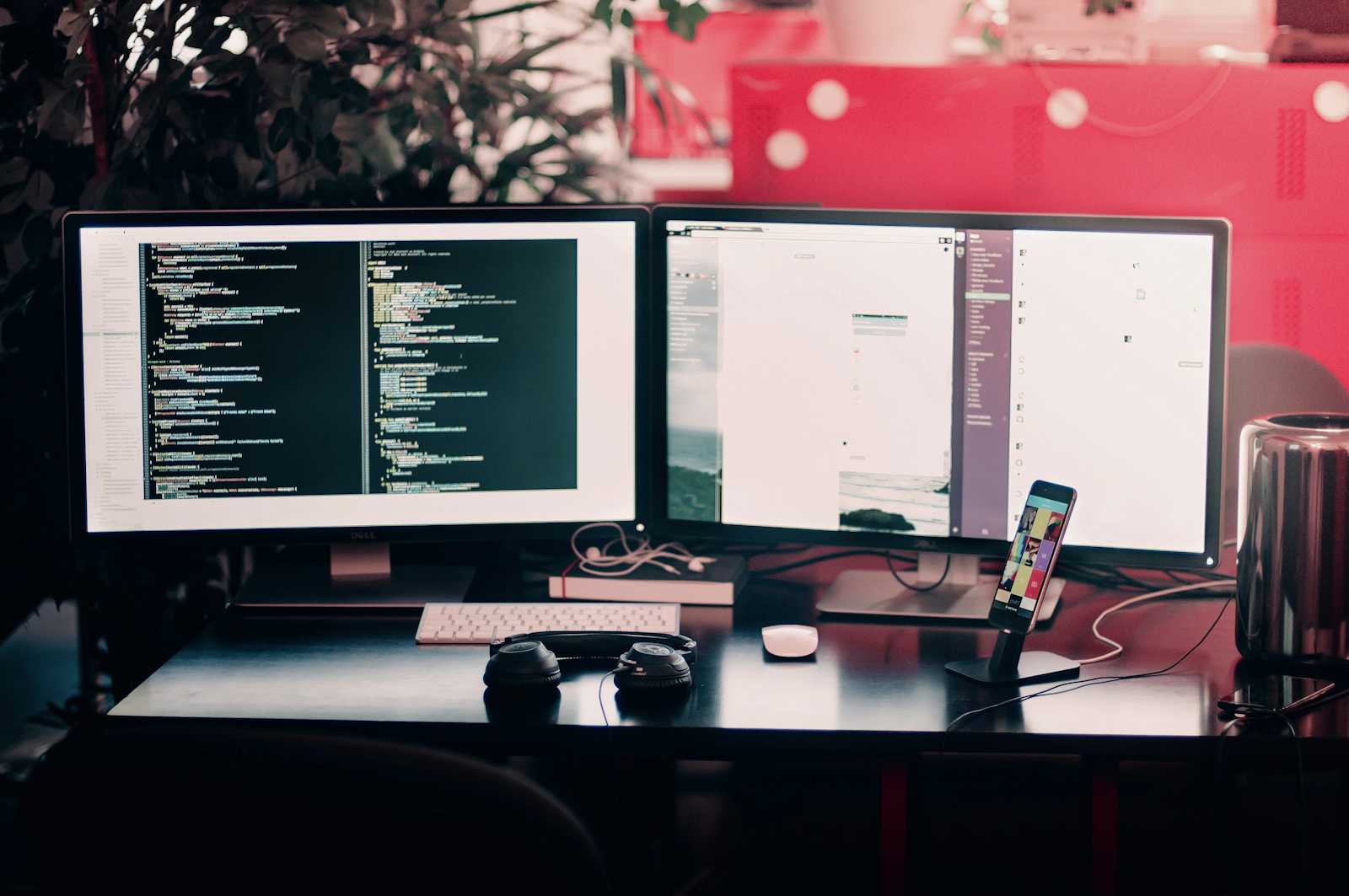
If you're starting a backend application, there are some must-have features that every developer should include. Based on my experience, these are essential for building a secure, scalable, and robust backend. Let’s dive in!
1. Authentication 🛡️
What It Is: Authentication is the process of verifying a user’s identity on your platform.
Why It’s Important: It ensures that only legitimate users can access your application, protecting it from unauthorized access and potential security breaches.
How to Do It: Use JWT for stateless authentication or OAuth 2.0 for third-party logins. Both have their pros and cons:
JWT: Lightweight and suitable for microservices.
OAuth 2.0: Great for allowing access through external platforms (e.g., Google, Facebook).
Also, ensure secure password handling by hashing passwords using algorithms like bcrypt.
2. Authorization 🔒
What It Is: Authorization defines what actions a user can perform or which resources they can access after their identity is verified. It’s a key security layer for your application.
Example: An admin can manage all resources, such as user data and system settings, while a manager may only access team-related information. This ensures users interact only with resources they are permitted to use, minimizing security risks.
How to Do It: Implement a role-based access control (RBAC) system:
Assign roles (e.g., Admin, Manager, User) to users.
Map each role to specific permissions.
Use middleware to dynamically check permissions for every user request.
Pro Tip: Follow the principle of least privilege—users should only have the minimum permissions they need.
3. Validate User Input 🛡️
Why It’s Important: The golden rule of backend development: never trust user data.
What to Do: Always validate incoming data to ensure it meets your expectations. Define exactly what data your API should accept, and reject anything that doesn’t match. Here’s how you can secure your backend:
Add strict validation checks for all inputs.
Specify required fields and their types (e.g., strings, numbers).
Reject extra keys or unexpected data.
Pro Tip: By validating inputs, you prevent hackers from exploiting vulnerabilities like sending malicious data to break or compromise your system. This simple step significantly strengthens your backend’s security.
4. Global Exception Handler 🛠️
What It Is: A centralized way to handle unexpected errors in your application.
Why It’s Important: While try-catch
blocks are great for handling known errors, a Global Exception Handler catches any unhandled errors, ensuring your application remains stable.
How to Do It:
Implement a global error-handling middleware (e.g., in Express, use
app.use()
).Standardize error messages to avoid exposing sensitive system details.
Return generic messages like “Something went wrong. Please try again later.”
Pro Tip: Log detailed error information internally for debugging but never send sensitive data to clients.
5. Effective Logging 🖋️
What It Is: Logs help you debug and understand your application’s behavior in production.
Why It’s Important: Good logging practices enable faster debugging and provide insights into how your application is performing.
How to Do It:
Use tools like Winston or Log4j for structured logging.
Include the following in your logs:
API endpoint called.
Payload (avoid sensitive info).
Key steps in the process.
Request ID and user details.
Final response sent to the user.
Pro Tip: Avoid logging excessive data, such as full responses. It clutters logs and can impact performance.
6. Request Limiter 🚦
What It Is: A mechanism to prevent API abuse by limiting the number of requests a user can make within a certain time frame.
Why It’s Important: This helps protect your system from misuse, such as DDoS attacks, and ensures fair usage of resources.
How to Implement:
Use Redis to store request counts for each user.
Implement middleware (e.g.,
express-rate-limit
) to block excessive requests.Example: Limit a user to 100 requests per minute to a specific API.
Pro Tip: Prevent multiple simultaneous API calls from the same user to avoid performance bottlenecks.
7. Docker 🐳
What It Is: Docker makes your backend application portable and easy to run anywhere.
Why Use It:
Avoid manual environment setup—Docker ensures your application runs consistently across machines.
Simplifies deployment on cloud platforms like AWS, GCP, or Azure.
How to Do It:
Write a
Dockerfile
to define your application’s environment.Use Docker Compose for managing multi-container applications (e.g., backend + database).
Optimize your Docker images by using lightweight base images like Alpine Linux.
Pro Tip: Always test your Dockerized app locally before deploying it to the cloud.
Final Thoughts
Adding these features to your backend will make it more secure, reliable, and scalable. Whether you're building for a startup or scaling for enterprise-level traffic, these modules will set you up for success.
Stay tuned for deeper dives into these topics in future blogs. Got questions or suggestions? Drop them in the comments! 😊
Subscribe to my newsletter
Read articles from Habib Qureshi directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
