Teleporting a human- Understanding Serialization and Deserialization in JavaScript.

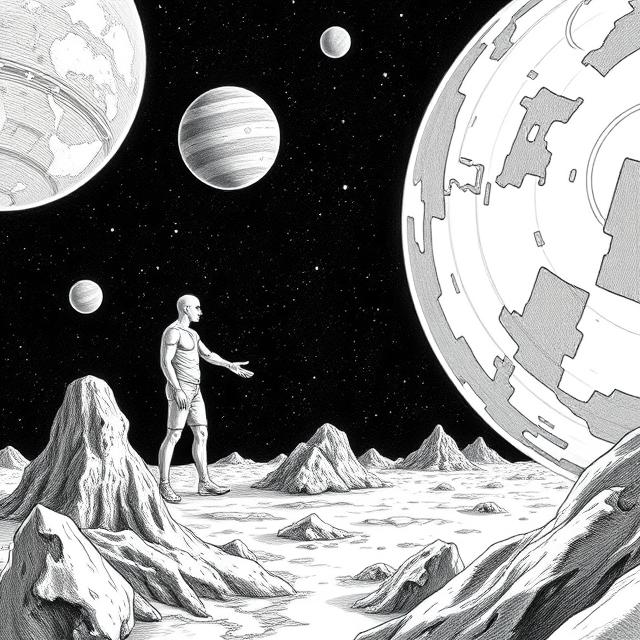
In the realm of JavaScript, serialization and deserialization play a very crucial role especially in the context of efficiently storing and transferring the data over the network. These concepts ensure that the data is seamlessly transferred over the network and is effectively received at the receiver’s end.
In this article we are going to see how the concept of serialization and deserialization can be utilized in teleporting a human from one planet to another.
First we shall look at how these concepts are used in JS.
Serialization
serialization can be defined as the process of converting data structure or object in such a form that it can be easily stored or transmitted over the network. In JavaScript the object is generally converted to a string format for the same. The most common technique for serialization of objects that’s used is JSON.stringify() method, the method converts the object format to a string format that can be easily stored or transferred.
const obj= {
name:"Sumrit",
order:"Shoes",
amount: 3490
};
// JS object for the order of shoes over an E-commerce website.That needs to be transferred for payment.
const serialize_obj= JSON.stringify(obj);
// serializing the object to a string format.
console.log(serialize_obj);
// output: '{"name":"Sumrit","order":"Shoes","amount":"3490"}',its now easy to transfer and store.
Understanding the same concept for human teleportation would involve converting the human properties unique to a particular human into a JS object notation and then converting the same to a string format to be transferred over a network onto the destination planet.
const atul_obj= {
name:"Atul",
height: "5'9",
age:28,
heartbeat_Range: "70-80 bpm",
saved_events: ["first-day of school","pet-dog's snoring","first two wheeler", "first college day","first offer-letter"],
weight:72
} ;
// Object containing unique properties of a human named atul.
const converted_obj = JSON.stringify(atul_obj);
// Converting and storing the object to a string.
console.log(converted_obj);
// '{"name":"Atul","height":"5'9","age":28,"heartbeat_Range":"70-80 bpm","saved_events":["first-day of school","pet-dog's snoring","first two wheeler","first college day","first offer-letter"],"weight":72}'
Benefits
1. Data Interchange
Serialization, particularly to JSON, provides a standardized format that can be easily understood and processed by different programming languages and systems. This makes it easier to share data between different applications, services, or platforms.
2. Storage Efficiency
Serialized data can be more compact than its in-memory representation, which can save storage space. JSON strings are often smaller than the equivalent JavaScript objects, especially when it comes to transmitting data over networks.
3. Network Transmission
When sending data over a network (e.g., via APIs), serialized data (like JSON) can be transmitted more efficiently than raw objects. This reduces the amount of data sent over the network, which can improve performance and reduce costs.
After serializing the data, next step is sending the data over a network, when the data reaches at its destination, there comes the next important step, which is deserialization. Let’s understand it.
Deserialization
Deserialization is the exact opposite of the concept of serialization. It involves converting the serialized data back to its original data structure or object, that can be used further in the program. In JavaScript, generally the serialized string is converted back to object notation so that it can be used further. Generally we use JSON.parse() method , the method converts the string back to JS object.
const obj= {
name:"Sumrit",
order:"Shoes",
amount: 3490
};
const serialize_obj= JSON.stringify(obj);
// Converting the object to string
const deserealize_obj = JSON.parse(serialize_obj);
// Converting the string back into object,and storing it.
console.log(deserealize_obj);
// output { name: 'Sumrit', order: 'Shoes', amount: 3490 }
Understanding the same concept for human teleportation would be like once we have successfully received the digital data we need to convert it back to physical form. This would include reassembling the human body and at the same time restoring the consciousness of the human, and this is most important part which’d need the properties from the object that’s derived after the deserialization.
const atul_obj= {
name:"Atul",
height: "5'9",
age:28,
heartbeat_Range: "70-80 bpm",
saved_events: ["first-day of school","pet-dog's snoring","first two wheeler", "first college day","first offer-letter"],
weight:72
} ;
const converted_obj = JSON.stringify(atul_obj);
const new_obj = JSON.parse(converted_obj);
// Converting the string back to object.
console.log(new_obj);
/*[
{
name: 'Atul',
height: "5'9",
age: 28,
heartbeat_Range: '70-80 bpm',
saved_events: [
'first-day of school',
"pet-dog's snorring",
'first two wheeler',
'first college day',
'first offer letter'
],
weight: 72
}
]*/
Benefits
1. Data Reconstruction
Deserialization allows applications to restore the state of an object or data structure from a previously serialized format. This is essential for applications as they can use the deserialized data for further processing or usage.
2. Interoperability
Deserialization enables different systems and applications to communicate effectively. For example, a web service can send serialized data to a client application, which can then deserialize it into a format that the application can work with, regardless of the programming language or platform.
3.Data Validation
During deserialization, data can be validated against expected types and structures. This can help catch errors early in the data processing pipeline, ensuring that the application receives valid and correctly formatted data.
Thanks to JavaScript that helped in understanding of Serialization and Decentralization and how these concepts can be applied for human teleportation.
Thanks for watching.
Subscribe to my newsletter
Read articles from Sumrit Gaba directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
