Cryptography: Caesar Cipher
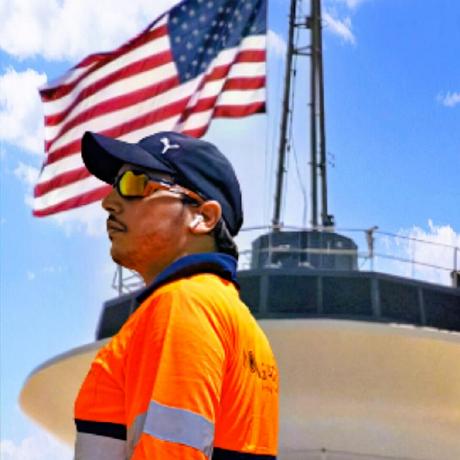

The Caesar Cipher is one of the simplest and most widely known encryption techniques. Named after Julius Caesar, who reportedly used it to protect military messages, this cipher works by shifting the letters in the plaintext by a fixed number of places in the alphabet.
Despite its simplicity, the Caesar Cipher is a fundamental concept in cryptography and serves as a good introduction to encryption techniques.
How the Caesar Cipher Works
The Caesar Cipher is a substitution cipher, meaning that each letter in the plaintext is replaced with another letter a fixed number of positions down (or up) in the alphabet.
Example:
Let’s take a shift of 3 and apply it to the word HELLO:
Plaintext: H E L L O
Shift: 3 3 3 3 3
Ciphertext: K H O O R
If the shift reaches beyond 'Z', it wraps around back to 'A'.
Mathematical Formula
For encryption, each letter is shifted using the formula:
E(x) = (x + n) mod 26
For decryption:
D(x) = (x - n) mod 26
Where:
x
is the numerical position of the letter (A=0, B=1, ..., Z=25)n
is the shift valuemod 26
ensures the result wraps around within the 26 letters of the alphabet
Implementing Caesar Cipher in C
Here’s a C# implementation of the Caesar Cipher, covering both encryption and decryption:
using System;
class CaesarCipher
{
static string Encrypt(string text, int shift)
{
char[] buffer = text.ToUpper().ToCharArray();
for (int i = 0; i < buffer.Length; i++)
{
if (char.IsLetter(buffer[i]))
{
char letter = (char)(buffer[i] + shift);
if (letter > 'Z')
letter = (char)(letter - 26);
buffer[i] = letter;
}
}
return new string(buffer);
}
static string Decrypt(string text, int shift)
{
return Encrypt(text, -shift);
}
static void Main()
{
Console.Write("Enter text: ");
string text = Console.ReadLine();
Console.Write("Enter shift value: ");
int shift = int.Parse(Console.ReadLine());
string encrypted = Encrypt(text, shift);
string decrypted = Decrypt(encrypted, shift);
Console.WriteLine($"Encrypted: {encrypted}");
Console.WriteLine($"Decrypted: {decrypted}");
}
}
Explanation:
Convert the input text to uppercase for consistency.
Iterate through each character and shift it by
n
places.If the letter exceeds
'Z'
, wrap it back to'A'
.For decryption, simply reverse the shift (
-n
).The user inputs the text and shift value, then the program encrypts and decrypts the text.
Implementing Caesar Cipher in Python
Now, let’s see the Python implementation:
def caesar_cipher(text, shift, mode='encrypt'):
result = ""
shift = shift if mode == 'encrypt' else -shift
for char in text.upper():
if char.isalpha():
shifted = ord(char) + shift
if shifted > ord('Z'):
shifted -= 26
elif shifted < ord('A'):
shifted += 26
result += chr(shifted)
else:
result += char # Preserve spaces and punctuation
return result
# Example usage
text = input("Enter text: ")
shift = int(input("Enter shift value: "))
encrypted = caesar_cipher(text, shift, 'encrypt')
decrypted = caesar_cipher(encrypted, shift, 'decrypt')
print(f"Encrypted: {encrypted}")
print(f"Decrypted: {decrypted}")
Explanation:
Convert the text to uppercase.
Iterate through each character and apply the shift.
If the new letter exceeds
'Z'
, wrap it back.Spaces and punctuation are preserved.
For decryption, we simply invert the shift (
-n
).
Strengths and Weaknesses of Caesar Cipher
Strengths:
✔ Simple to understand and implement.
✔ Provides basic security for casual or low-risk scenarios.
✔ Helps introduce concepts of cryptography.
Weaknesses:
❌ Easily breakable – A brute-force attack with 25 shifts can decrypt any message.
❌ No key complexity – The key (shift value) is predictable and small.
❌ Letter frequency analysis – Patterns in the encrypted text can be analyzed to break the cipher.
Variants and Improvements
To improve the security of the Caesar Cipher, variations have been developed:
Vigenère Cipher – Uses multiple shifts based on a keyword.
Atbash Cipher – A simple reversal cipher where A ↔ Z, B ↔ Y, etc.
ROT13 – A specific case of Caesar Cipher with a shift of 13, often used in programming.
Modern Encryption (AES, RSA, etc.) – Stronger cryptographic methods are used today.
Subscribe to my newsletter
Read articles from M B A R K directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
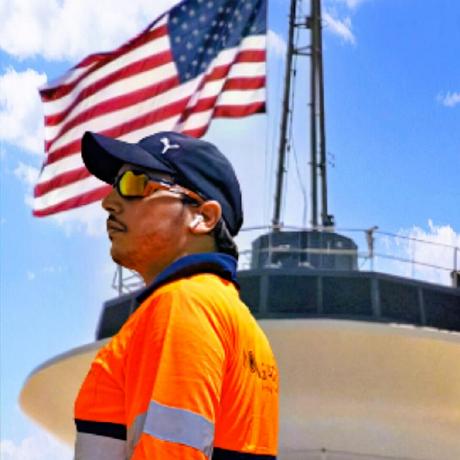
M B A R K
M B A R K
I’m a passionated .NET/C# developer, who likes to play around with C# and see how much I can do with it.