Array method in the matrix world

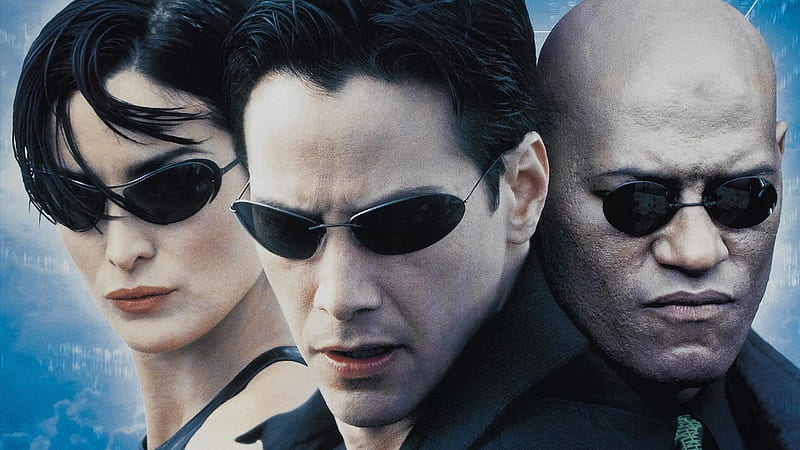
The Matrix: Code of the Arrays
Introduction
In the heart of the Matrix, a group of rebels fights against the digital overlords. Their existence depends on a mysterious force known as the Array Methods, which allow them to manipulate reality itself. Neo, Trinity, Morpheus, and others must use these powers to break free from the simulated world.
Chapter 1: Awakening the Arrays (Array length
)
Neo wakes up inside a digital construct. Morpheus stands before him and gestures toward a list of names.
let rebels = ["Neo", "Trinity", "Morpheus", "Tank", "Dozer"];
console.log(rebels.length); // 5
"There are five of us in this squad," Morpheus says. "But the system is always watching."
Neo realizes that length determines the size of their group. If one of them disappears, their numbers will shrink.
Chapter 2: Seeing the Code (Array toString()
)
Neo begins to perceive the Matrix not as reality, but as data.
console.log(rebels.toString());
// "Neo,Trinity,Morpheus,Tank,Dozer"
"The Matrix is just a sequence of data," Morpheus explains. "You can see us as mere strings within it."
Chapter 3: Finding the One (Array at()
)
The Oracle tells Neo that "The One" is among them. Morpheus looks at the rebel list and checks position 2.
console.log(rebels.at(2)); // "Morpheus"
"It's me," Morpheus smirks. "But I was only the guide. The real One is still awakening."
Neo realizes that at() allows him to select anyone at a specific index.
Chapter 4: Uniting the Team (Array join()
)
The rebels must send a message to Zion, but the transmission must be encoded into a single string.
let encodedMessage = rebels.join(" - ");
console.log(encodedMessage);
// "Neo - Trinity - Morpheus - Tank - Dozer"
"This is our message to Zion," says Trinity. "A single, unbroken string of hope."
Chapter 5: The First Loss (Array pop()
)
Suddenly, Dozer is taken out by an Agent!
rebels.pop();
console.log(rebels);
// ["Neo", "Trinity", "Morpheus", "Tank"]
"Dozer is gone," whispers Morpheus. "The team is shrinking."
Neo realizes that pop() removes the last element, just like when a rebel falls in battle.
Chapter 6: The New Recruit (Array push()
)
The rebels recruit a new member: Cypher.
rebels.push("Cypher");
console.log(rebels);
// ["Neo", "Trinity", "Morpheus", "Tank", "Cypher"]
"He may be useful," says Tank. But Neo feels uneasy…
(What they don’t know is that push() adds elements, but not all additions are good ones.)
Chapter 7: The Betrayal (Array shift()
)
Cypher betrays the team, revealing their location to the Agents!
rebels.shift();
console.log(rebels);
// ["Trinity", "Morpheus", "Tank", "Cypher"]
Neo, the first name in the list, is temporarily removed—he is captured by Agent Smith!
Chapter 8: A New Leader (Array unshift()
)
With Neo gone, Morpheus steps forward as the leader again.
rebels.unshift("Morpheus");
console.log(rebels);
// ["Morpheus", "Trinity", "Tank", "Cypher"]
"I will not let the prophecy die," Morpheus declares.
(Unshift() adds a new leader at the front of the team.)
Chapter 9: Erasing an Agent (Array delete()
)
Tank, wounded but alive, removes Cypher from their system.
delete rebels[3];
console.log(rebels);
// ["Morpheus", "Trinity", "Tank", empty]
"He's no longer one of us," says Trinity.
(The delete
method removes a value but leaves an empty hole, much like Cypher's betrayal left scars on their mission.)
Chapter 10: The Resistance Grows (Array concat()
)
The rebels meet another team from Zion: Niobe and Ghost. They unite forces.
let zionRebels = ["Niobe", "Ghost"];
let fullResistance = rebels.concat(zionRebels);
console.log(fullResistance);
// ["Morpheus", "Trinity", "Tank", empty, "Niobe", "Ghost"]
"Together, we are stronger."
(Concat() brings multiple arrays together into one resistance.)
Chapter 11: The Glitch in the Matrix (Array copyWithin()
)
Neo sees a Déjà Vu—a black cat passing twice.
fullResistance.copyWithin(1, 3, 4);
console.log(fullResistance);
// ["Morpheus", empty, "Tank", empty, "Niobe", "Ghost"]
"It's a glitch," Trinity whispers.
(The copyWithin()
method overwrites elements, like a system glitch.)
Chapter 12: The Layers of Reality (Array flat()
)
Neo finally sees the true depth of the Matrix, realizing it's built-in layers.
let matrixLayers = [[["Illusion"]], [["Control"]], [["Truth"]]];
console.log(matrixLayers.flat(2));
// ["Illusion", "Control", "Truth"]
(The flat()
method collapses layers, just as Neo collapses the illusion.)
Chapter 13: Changing Destiny (Array splice()
)
Neo hacks reality to remove the Agents from the simulation.
fullResistance.splice(1, 2, "Neo", "The Oracle");
console.log(fullResistance);
// ["Morpheus", "Neo", "The Oracle", empty, "Niobe", "Ghost"]
(The splice()
method removes elements and inserts new ones, reshaping fate.)
Chapter 14: The Final Cut (Array toSpliced()
)
In the final battle, Neo removes himself from the equation.
let newWorld = fullResistance.toSpliced(1, 1);
console.log(newWorld);
// ["Morpheus", "The Oracle", empty, "Niobe", "Ghost"]
(The toSpliced()
method creates a new version, just as Neo becomes part of the source code itself.)
Chapter 15: A New Beginning (Array slice()
)
The story continues, but only a part of it remains known.
let survivors = fullResistance.slice(0, 3);
console.log(survivors);
// ["Morpheus", "Neo", "The Oracle"]
(The slice()
method extracts a portion of the array, leaving the rest of the story untold.)
Conclusion
The Matrix was built on arrays, and those who understand them can shape reality. Now, the question is… will you code your own destiny?
Subscribe to my newsletter
Read articles from ARYAN RAJ directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
