JavaScript Top 10 Methods For Arrays

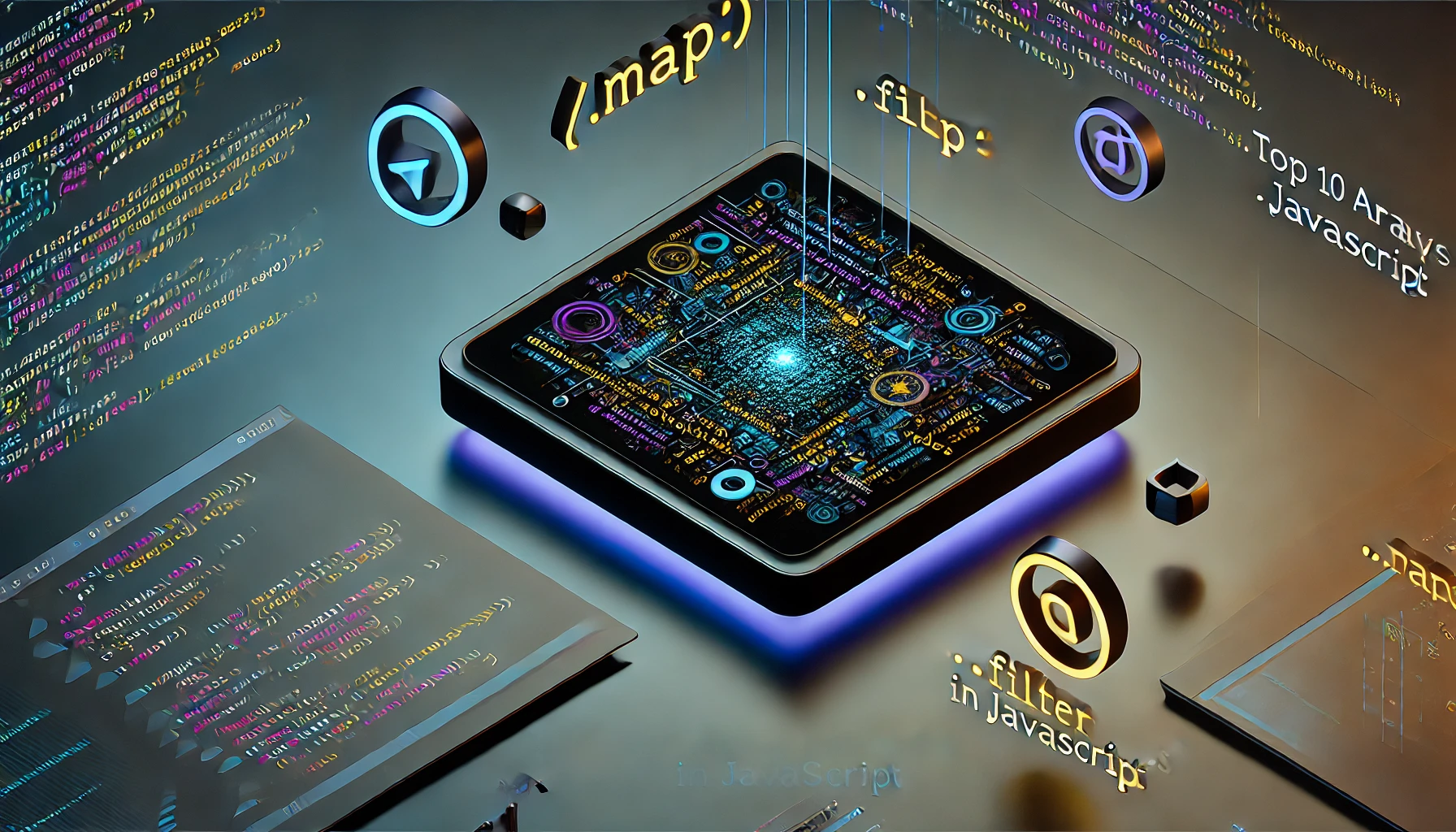
Here I will be sharing my top 10 methods for arrays in js.
push( element )
pop( )
indexOf( element, initial index )
at( index )
forEach( callbackFunction )
includes ( element )
toString ( )
flat( nestingLimits )
concat( …element, …element, etc.. )
reverse()
push ( element
)
Push method adds element in the end of the array.
const nums = [1, 3, 5];
console.log(nums); // output : [1, 3, 5]
nums.push(7);
console.log(nums); // output : [1, 3, 5, 7]
pop ()
Pop removes the element from the end of the array and also returns that removed element.
const nums = [1, 2, 3, 4, 5];
console.log(nums); // output : [1, 2, 3, 4, 5]
let removedValue = nums.pop();
console.log(removedValue); // output : 5
console.log(nums); // output : [1, 2, 3, 4]
indexOf ( element
, initial index
)
It searches the element in the array and returns the index of that element. Using this method we can also set the initial index from that the element to be searched.
// indexOf(element, initial index)
const nums = [1, 2, 3, 4, 5];
console.log(nums); // output : [1, 2, 3, 4, 5]
// Searching element without initial index
console.log(nums.indexOf(2)); // output : 1
// Searching element with initial index
console.log(nums.indexOf(5, 2)); // output : 4
console.log(nums.indexOf(1, 3)); // output : -1
at ( index
)
It returns the element present on the index if found. This method accepts both positive indexing (0 to length - 1 ) and negative indexing (-length to -1 ).
const nums = [1, 2, 3, 4, 5];
console.log(nums); // output : [1, 2, 3, 4, 5]
// Positive indexing => 0, .....(Array.length - 1)
console.log(nums.at(2)); // output : 3
console.log(nums.at(6)); // output : undefined
// Negative indexing => -(Array.length), ........ -1,
console.log(nums.at(-5)); // output : 1
console.log(nums.at(-6)); // output : undefined
forEach( callbackfun(value, index, Array)
)
With this method, we can iterate through the arrays and access each element seprately.
const nums = [1, 2, 3, 4, 5];
console.log(nums); // output : [1, 2, 3, 4, 5]
/* In this iteration we are only accessing values
so we don't have to write index and Array. */
nums.forEach((element) => {
console.log(element);
})
/* output :
1
2
3
4
5
*/
includes ( element
, initial index
)
It searches the element in the array and returns true if found otherwise false. It doesn't return the index like indexOf()
method.
const nums = [1, 2, 3, 4, 5];
console.log(nums); // output : [1, 2, 3, 4, 5]
// Searching element without initial index
console.log(nums.includes(2)); // output : true
// Searching element with initial index
console.log(nums.includes(5, 2)); // output : true
console.log(nums.includes(1, 3)); /*// output : false => after index 3
not a single 1 is present. */
toString ( )
This method creates a new string from the Array and return the string. It doesn't manipulates the original Array.
const nums = [1, 2, 3, 4, 5];
console.log(nums); // output : [1, 2, 3, 4, 5]
// take temp to store the converted string
const temp = nums.toString();
console.log(temp); // output : 1,2,3,4,5
console.log(typeof temp); // output : string
// Checking original array
console.log(nums); // output : [1, 2, 3, 4, 5]
flat ( depth
)
This method removes the depth in the array and creates a new array. It doesn't manipulate the original array.
const nums = [1, [2, 3, [4, 5]]]; // Highest depth : 2
console.log(nums); // output : [ 1, [ 2, 3, [ 4, 5 ] ] ]
console.log(nums.flat(1)); // output : [ 1, 2, 3, [ 4, 5 ] ]
console.log(nums.flat(2)); // output : [ 1, 2, 3, 4, 5 ]
// Checking original Array
console.log(nums); // output : [ 1, [ 2, 3, [ 4, 5 ] ] ]
concat ( …value1, …value2, …etc
)
This method joins all arrays and returns a new array. It doesn't manipulate the original array.
const nums1 = [1, 2, 3];
const nums2 = [4, 5, 6];
const nums3 = [7, 8, 9];
console.log(nums1, nums2, nums3); //output : [ 1, 2, 3 ] [ 4, 5, 6 ] [ 7, 8, 9 ]
// Joining all arrays in single array
const temp = nums1.concat(...nums2, ...nums3);
console.log(temp); // output : [1, 2, 3, 4, 5, 6, 7, 8, 9]
reverse ( )
This method changes the order of the order of the element in the array and also changes the original array.
const nums = [1, 2, 3, 4, 5, 6, 7, 8, 9];
console.log(nums); //outptut : [1, 2, 3, 4, 5, 6, 7, 8, 9]
nums.reverse();
console.log(nums); // output : [9, 8, 7, 6, 5, 4, 3, 2, 1]
So this was my top 10 methods for array in JavaScript. I know that I haven’t added map, filter and reduce for my personal reasons.
Subscribe to my newsletter
Read articles from Aquib Jawed directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
