Java Exception Handling Explained: A Complete How-To Guide
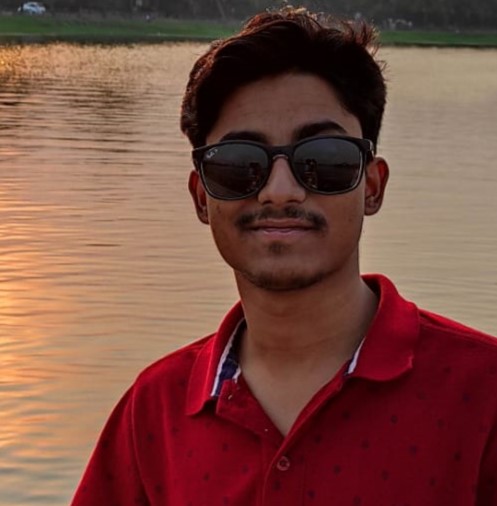

Hey everyone! 👋 I’ve been learning Java recently, and one of the most interesting (and sometimes confusing!) topics I came across was exception handling. At first, it felt overwhelming, but after some trial and error, I finally started to understand how exceptions work and why they are so important.
In this blog, I’ll share what I’ve learned about exception handling in Java, including the different types of errors, how to use try-catch
, and the difference between throw
and throws
. If you're also trying to wrap your head around this topic, I hope this guide helps you as much as it helped me! 😊
🔹 1. Understanding Errors in Java
Before diving into exceptions, I had to first understand that not all errors are the same. Java programs can run into three main types of errors:
1️⃣ Compile-Time Errors (Syntax Mistakes)
These are errors that the Java compiler catches before running the program.
If there’s a missing semicolon or a typo, Java won’t let the program run until you fix it.
✅ Example (Syntax Error):
System.out.println("Hello); // ❌ Missing closing quote causes an error
🔹 How I fixed it: The compiler showed an error, so I added the missing "
at the end.
2️⃣ Logical Errors (Bugs in Code)
These are mistakes in logic where the program runs fine but gives the wrong result.
The compiler won’t catch these errors, so you have to debug your code carefully.
✅ Example (Logic Error):
int result = 2 + 2; // Expecting 4, but mistakenly coded as 5
🔹 How I fixed it: Double-checked my calculations and corrected the code.
3️⃣ Runtime Errors (Exceptions!)
These errors happen while the program is running, causing it to crash.
If we don’t handle them, Java will terminate the program immediately.
✅ Example (Division by Zero - ArithmeticException):
int a = 10 / 0; // ❌ ArithmeticException: Division by zero
🔹 How I fixed it: I used exception handling (which I’ll explain below!).
🔹 2. What Are Exceptions and Why Should We Handle Them?
At first, I thought exceptions were just another type of error, but I learned that exceptions are actually Java’s way of signaling a problem. If an exception occurs, Java stops executing the program unless we handle it properly.
Why Handle Exceptions?
✔️ Prevents program crashes (important for apps that shouldn’t suddenly stop!).
✔️ Helps users understand what went wrong (instead of showing confusing error messages).
✔️ Ensures smooth execution by handling errors gracefully.
🔹 3. My First Try-Catch Block in Java
Java provides the try-catch mechanism to handle exceptions, and this was my first step in writing error-proof code.
✅ Syntax of Try-Catch
try {
// Code that may cause an exception
} catch (ExceptionType e) {
// Code to handle the exception
}
✅ Example: Handling Division by Zero
try {
int result = 10 / 0; // 🚨 ArithmeticException occurs here
} catch (ArithmeticException e) {
System.out.println("Cannot divide by zero!");
}
🔹 How It Works:
The code inside
try
executes first.If an exception occurs, Java jumps to the
catch
block and handles the error.If there’s no exception, Java skips the
catch
block and continues execution.
🔹 4. Handling Multiple Exceptions
While practicing, I realized that a program can throw different exceptions, and I needed to handle them separately.
✅ Example: Catching Multiple Exceptions
try {
int[] arr = new int[5];
arr[10] = 50; // 🚨 ArrayIndexOutOfBoundsException
} catch (ArithmeticException e) {
System.out.println("Arithmetic Error");
} catch (ArrayIndexOutOfBoundsException e) {
System.out.println("Array Index Error");
} catch (Exception e) {
System.out.println("Some other error occurred");
}
🔹 What I learned:
Specific exceptions should be caught first (
ArrayIndexOutOfBoundsException
beforeException
).The most general exception (
Exception
) should be caught last.
🔹 5. throw
vs throws
: What’s the Difference?
This part confused me at first! But after experimenting, here’s what I understood:
Feature | throw | throws |
Definition | Used inside a method to manually trigger an exception | Used in method declaration to indicate potential exceptions |
Where It’s Used | Inside a method or block | In the method signature |
Example Usage | throw new IOException("Error"); | public void readFile() throws IOException {} |
✅ Example of throw
(Manually Throwing an Exception)
public void validateAge(int age) {
if (age < 18) {
throw new IllegalArgumentException("Age must be 18 or above");
}
}
✅ Example of throws
(Declaring an Exception in a Method)
public void readFile() throws IOException {
FileReader file = new FileReader("data.txt");
}
🔹 Key Learning: throw
is used inside a method, while throws
is used in the method signature to tell Java that an exception might occur.
🔹 6. My Takeaways & Best Practices for Exception Handling
✔️ Use specific exceptions instead of Exception
(it helps pinpoint the error).
✔️ Log exceptions instead of just printing them (e.printStackTrace()
).
✔️ Use finally
to clean up resources (closing files, database connections).
✔️ Avoid empty catch blocks (catch (Exception e) { }
does nothing useful!).
✔️ Don’t use exceptions for normal logic flow (exceptions should be used for errors, not for controlling the program).
In a nutshell 🥜
When I first started learning Java exception handling, I thought it was complex and unnecessary. But after experimenting with try-catch, throw, and throws, I realized how important and useful it is for writing reliable code.
If you’re also learning Java, I’d suggest practicing different exceptions, writing custom exceptions, and experimenting with try-catch blocks. It really helps to see how Java handles errors in real-time!
💡 What’s Next? In my next blogs, I’ll explore checked vs unchecked exceptions and custom exceptions in more detail. Stay tuned, and let’s keep learning together! 🚀
Subscribe to my newsletter
Read articles from Arkadipta Kundu directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
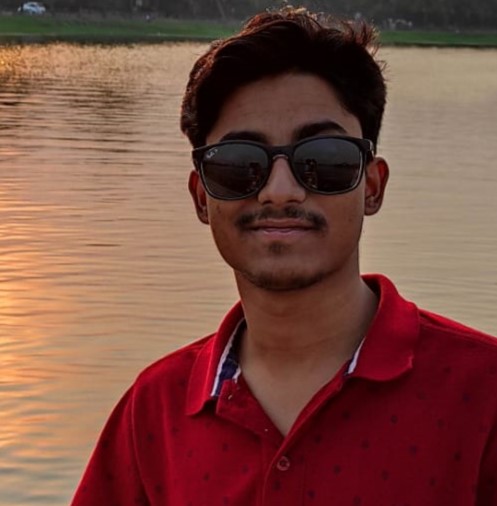
Arkadipta Kundu
Arkadipta Kundu
I’m a Computer Science undergrad from India with a passion for coding and building things that make an impact. Skilled in Java, Data Structures and Algorithms (DSA), and web development, I love diving into problem-solving challenges and constantly learning. Right now, I’m focused on sharpening my DSA skills and expanding my expertise in Java development.