Understanding Java User Input: BufferedReader vs Scanner Compared
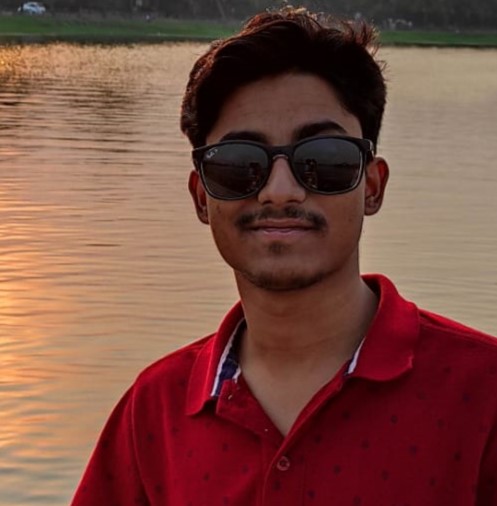

When working with Java applications, user input is important for interactive programs. Java provides several ways to handle input, but two common methods are:
1️⃣ BufferedReader – Good for large inputs but needs manual type conversion.
2️⃣ Scanner – Easy to use, supports automatic type conversion, but is a bit slower.
In this guide, we’ll compare BufferedReader and Scanner, look at their pros and cons, and talk about best practices for closing resources to avoid memory leaks.🚀
🛠️ 1. Introduction to User Input in Java
Java allows us to take input from the user using the console (keyboard) or from external sources like files. Two widely used classes for reading input are:
BufferedReader
(fromjava.io
package)Scanner
(fromjava.util
package)
Each has its own advantages, and understanding them helps in choosing the right one for different use cases.
📌 2. Using BufferedReader in Java
🔹 What is BufferedReader?
BufferedReader
is a class that efficiently reads text from an input stream (like the keyboard or files). It is commonly used with InputStreamReader
for reading user input from the console.
🔹 How to Use BufferedReader?
✅ Example: Reading User Input Using BufferedReader
import java.io.*;
public class BufferedReaderExample {
public static void main(String[] args) {
try {
BufferedReader br = new BufferedReader(new InputStreamReader(System.in));
System.out.println("Enter your name:");
String name = br.readLine(); // Reads a line of input
System.out.println("Hello, " + name);
br.close(); // Closing the resource
} catch (IOException e) {
e.printStackTrace();
}
}
}
🔹 Features of BufferedReader
✔️ Reads input line by line using readLine()
.
✔️ Uses an internal buffer, making it efficient for large input.
✔️ Requires manual type conversion (e.g., converting String
to int
).
📌 Pros & Cons of BufferedReader
✅ Pros | ❌ Cons |
Faster and efficient | Requires manual type conversion |
Suitable for large inputs | More lines of code required |
Can read input from files | Needs explicit exception handling |
📌 3. Using Scanner in Java
🔹 What is Scanner?
Scanner
is a class that provides an easy and flexible way to read user input. Unlike BufferedReader
, Scanner
can directly convert input into different data types (e.g., integers, doubles).
🔹 How to Use Scanner?
✅ Example: Reading User Input Using Scanner
import java.util.Scanner;
public class ScannerExample {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
System.out.println("Enter your age:");
int age = sc.nextInt(); // Reads an integer input
System.out.println("Your age is: " + age);
sc.close(); // Closing the resource
}
}
🔹 Features of Scanner
✔️ Reads input token by token.
✔️ Supports direct type conversion (nextInt()
, nextDouble()
, nextBoolean()
).
✔️ Can read entire lines using nextLine()
.
📌 Pros & Cons of Scanner
✅ Pros | ❌ Cons |
Easy to use | Slightly slower than BufferedReader |
Built-in type conversion | nextLine() can cause issues after nextInt() |
Works well for small input | Not suitable for large input files |
📌 5. BufferedReader vs Scanner: A Quick Comparison
Feature | BufferedReader | Scanner |
Performance | Faster | Slower for large input |
Type Conversion | Requires manual parsing (Integer.parseInt() ) | Built-in (nextInt() , nextDouble() ) |
Input Handling | Reads whole line (readLine() ) | Reads tokens (nextInt() , nextDouble() ) |
Exception Handling | Needs try-catch (IOException ) | No try-catch needed |
Best Used For | Large data, file reading | Small inputs, interactive programs |
📌 6. Practical Use Cases
🔹 When to Use BufferedReader?
✔️ When handling large input data.
✔️ When reading data from files.
✔️ When performance and efficiency matter.
🔹 When to Use Scanner?
✔️ When reading small user input from the console.
✔️ When built-in type conversion is needed.
✔️ When working with simple interactive programs.
📌 7. Conclusion
Both BufferedReader
and Scanner
are powerful tools for taking user input in Java. While BufferedReader
is more efficient for large data, Scanner
is easier to use for small, interactive applications.
Key Takeaways:
✔️ Use BufferedReader
when performance is critical (e.g., reading large files).
✔️ Use Scanner
when working with small inputs and need quick type conversion.
✔️ Always close resources properly to prevent memory leaks.
Would you like an advanced example integrating both methods? Let me know in the comments! 🚀
Subscribe to my newsletter
Read articles from Arkadipta Kundu directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
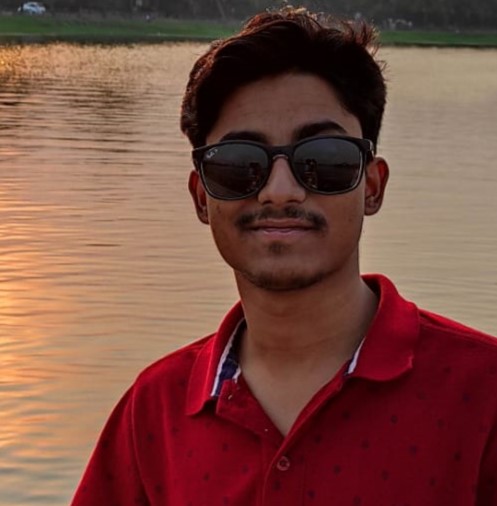
Arkadipta Kundu
Arkadipta Kundu
I’m a Computer Science undergrad from India with a passion for coding and building things that make an impact. Skilled in Java, Data Structures and Algorithms (DSA), and web development, I love diving into problem-solving challenges and constantly learning. Right now, I’m focused on sharpening my DSA skills and expanding my expertise in Java development.