Understanding the Difference: Laravel Lazy Loading vs Eager Loading
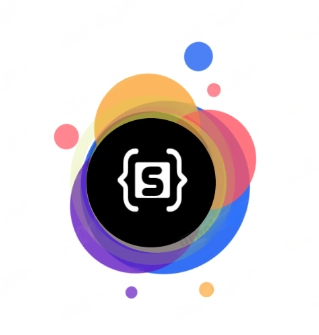
Table of contents

Lazy and Eager loading are different strategies for loading data from the database. They are used to optimize the performance of the Laravel application.
Lazy Loading
Lazy Loading is a technique in which related data is loaded from the database only when it is accessed. This means that the related Eloquent model data is not retrieved until the relationship is explicitly accessed in the code. Eloquent Relationships must be defined in the models for lazy loading to work. Eloquent relationships are defined as methods in your Eloquent model classes.
How it works…
When you query the main model, Laravel retrieves only the main data from the database. If you need related data, a separate query is executed when you access the relationship.
$author = Author::find(10); // This fetches the specific author data.
At this point, only the author's data is fetched. If you want to fetch the books related to that author, you need to access the relationship which is defined in the model
Eloquent relationship example in Author.php - Model
// Define the relationship an author has many books
public function books()
{
return $this->hasMany(Book::class);
}
// Access the relationship
$books = $author->books; // new query to fetch the books for that author.
When you retrieve a model, the related models are not loaded by default, only when the related relationship in accessed, the query is executed to fetch the related data.
The N+1 Query Problem
Lazy loading can lead to the N+1 query problem when you loop through multiple parent models and access their relationships.
Lazy loading is most suitable in scenarios where the related data is not always needed. Otherwise, it can lead to performance issues.
Eager Loading
Eager loading is a technique where related data models are loaded simultaneously with the main model. This means that when user data is fetched, the related user data is also retrieved in a single query.
How it works…
$user = User::with(‘post’)->find(10);
Laravel provides eager loading using the with()
method. This method loads related model data efficiently. This can reduce the number of executed queries. It helps improve performance and prevents the N+1 query problem.
This can increase the initial load time if too much related data is loaded at once. It also consumes more memory.
However, both methods are necessary. When choosing between eager loading and lazy loading, consider the specific use case and the performance requirements of the web application.
Subscribe to my newsletter
Read articles from Code With Shashika directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
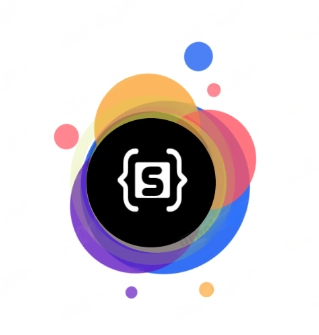
Code With Shashika
Code With Shashika
I am a Senior Web Developer | Software Engineer (Laravel, WordPress) who is experienced for more than 5 years.