Part 8: Mastering Databases

Table of contents
- 1. Building a Full-Stack Application: Database Integration with APIs
- 2. Database DevOps: Version Control, CI/CD, and Schema Migrations
- 3. Advanced Projects: Designing a Database for a Million Users
- 4. Contributing to Open-Source Databases
- 5. Becoming a Database Expert: Certifications and Career Paths
- Conclusion
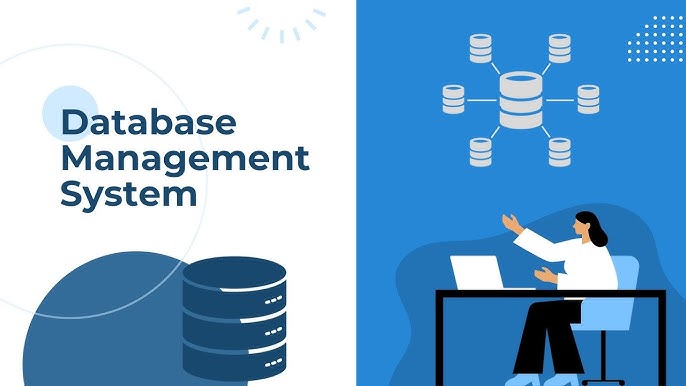
1. Building a Full-Stack Application: Database Integration with APIs
Full-Stack Architecture
Modern applications rely on seamless database integration. Here’s how to connect databases to frontend frameworks like React using REST or GraphQL APIs.
Example: Blogging Platform
Tech Stack:
Frontend: React
Backend: Node.js/Express
Database: PostgreSQL with Sequelize (ORM).
Step 1: Define Models with Sequelize
// models/Post.js
module.exports = (sequelize, DataTypes) => {
const Post = sequelize.define('Post', {
title: DataTypes.STRING,
content: DataTypes.TEXT,
authorId: DataTypes.INTEGER
});
return Post;
};
Step 2: Create API Endpoints
// routes/posts.js
router.get('/posts', async (req, res) => {
const posts = await Post.findAll();
res.json(posts);
});
router.post('/posts', async (req, res) => {
const post = await Post.create(req.body);
res.status(201).json(post);
});
Step 3: Frontend Integration
// React component
fetch('/api/posts')
.then(response => response.json())
.then(data => setPosts(data));
Challenge: Handling relational data (e.g., comments on posts).
Solution: Use eager loading with include
in Sequelize:
Post.findAll({ include: Comment });
2. Database DevOps: Version Control, CI/CD, and Schema Migrations
Version Control for Databases
Tools like Liquibase or Flyway track schema changes in code.
Example: Flyway Migration File
-- V1__create_users_table.sql
CREATE TABLE users (
id INT PRIMARY KEY,
email VARCHAR(255) UNIQUE NOT NULL
);
-- V2__add_phone_number.sql
ALTER TABLE users ADD COLUMN phone VARCHAR(20);
CI/CD Pipeline:
Commit migration files to Git.
Automate Testing: Run migrations in a staging environment.
Deploy: Apply migrations to production via Jenkins/GitHub Actions.
Challenge: Rolling back failed migrations.
Solution: Use flyway.undo scripts or backup snapshots.
3. Advanced Projects: Designing a Database for a Million Users
Scalability Strategies
Sharding: Split
Users
table by geographic region.Caching: Use Redis to store frequently accessed profiles.
Load Balancing: Distribute traffic across read replicas.
Case Study: Social Media App
Requirements:
1M daily active users.
10K posts per second.
Design:
Database: Cassandra (write scalability).
Caching: Redis for feed generation.
CDN: Cache images/videos to reduce DB load.
Query Optimization:
-- Denormalize data to avoid joins
CREATE TABLE user_feed (
user_id UUID,
post_id UUID,
content TEXT,
PRIMARY KEY (user_id, post_id)
);
4. Contributing to Open-Source Databases
How to Get Started
Choose a Project: Start with beginner-friendly issues (e.g., PostgreSQL’s “good first issue” tags).
Set Up Locally: Clone the repo, read contribution guidelines.
Fix a Bug: Improve documentation or tackle a minor bug.
Example:
Issue: “Improve PostgreSQL’s JSONB query performance.”
Contribution: Optimize index usage for JSONB paths.
Challenge: Understanding complex codebases.
Solution: Engage with community forums and mentorship programs.
5. Becoming a Database Expert: Certifications and Career Paths
Certifications
Oracle Database Administrator Certified Professional
MongoDB Certified Developer
AWS Certified Database – Specialty
Career Paths
Database Administrator (DBA): Focus on maintenance, backups, and security.
Data Engineer: Build ETL pipelines and data warehouses.
Database Architect: Design scalable systems for enterprises.
Learning Resources
Books: Designing Data-Intensive Applications by Martin Kleppmann.
Communities: Stack Overflow, Reddit’s r/Database.
Conclusion
Mastering databases is a journey of continuous learning. From building full-stack apps to optimizing distributed systems, the skills you’ve gained empower you to solve real-world challenges. Whether contributing to open-source or pursuing certifications, the database landscape offers endless opportunities for growth.
The End of the Series: You’ve progressed from foundational concepts to mastery. Now, go build something extraordinary!
FAQs
Q: How do I transition from a backend developer to a database architect?
A: Focus on scalability projects, learn distributed systems, and earn advanced certifications.
Q: Are open-source contributions necessary for career growth?
A: Not mandatory, but they showcase expertise and collaboration skills.
Q: Which certification is most valued in 2023?
A: AWS Certified Database – Specialty is highly sought-after due to cloud adoption.
Subscribe to my newsletter
Read articles from CodeXoft KE directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

CodeXoft KE
CodeXoft KE
Full-stack developer specializing in building exceptional digital experiences. Transforming ideas into elegant solutions through clean code and intuitive design.