Secrets of Java Inner Classes: What They Are, Why You Need Them, and How to Use Them Effectively
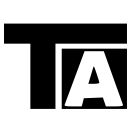
7 min read
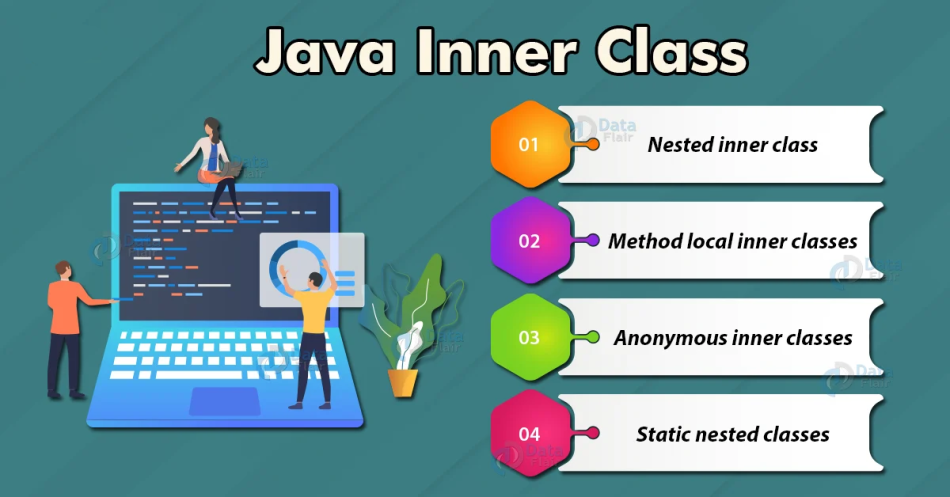
Source: Secrets of Java Inner Classes: What They Are, Why You Need Them, and How to Use Them Effectively
1. What Are Java Inner Classes?
Java Inner Classes are classes defined within another class. The defining feature of an inner class is that it has access to the members (both private and public) of its enclosing class. This makes inner classes extremely powerful for structuring code that logically belongs together. Java supports different types of inner classes, each serving a specific purpose.
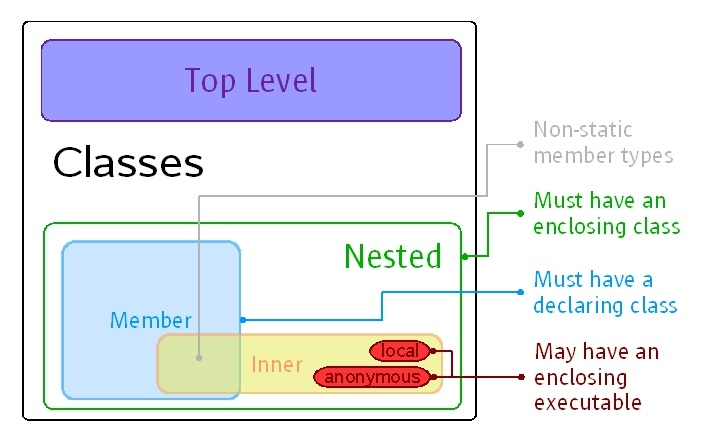
1.1 Why Use Inner Classes?
Inner Classes allow you to group classes that should be used together, leading to a cleaner and more understandable design. Here are a few reasons why you would consider using them:
- Encapsulation: By nesting classes, you can hide the internal logic of one class within another, enhancing the encapsulation.
- Logical Grouping: Classes that are tightly related in terms of functionality can be bundled together, making the code easier to maintain and follow.
- Enhanced Access: Inner classes have access to private members of the outer class, which can be useful for certain algorithms or design patterns like event handling.
Here’s an example of a basic inner class:
class Outer {
private String message = "Hello from the Outer class!";
class Inner {
void displayMessage() {
System.out.println(message); // Accessing private member of Outer class
}
}
}
public class Main {
public static void main(String[] args) {
Outer outer = new Outer();
Outer.Inner inner = outer.new Inner();
inner.displayMessage(); // Output: Hello from the Outer class!
}
}
1.2 Types of Inner Classes
There are four types of inner classes in Java:
- Regular Inner Class: This is a simple class declared inside another class. It has access to the members of the outer class.
- Static Nested Class: Unlike regular inner classes, a static nested class cannot access the outer class's non-static members. However, it can be instantiated without an instance of the outer class.
- Local Inner Class: These are classes defined within a block of code, usually inside a method. They can only be instantiated within the block where they are defined.
- Anonymous Inner Class: These are special types of local inner classes that do not have a name. They are commonly used to implement interfaces or extend classes on the fly.
Let’s explore these types in more detail with examples.
2. Regular Inner Class
A regular inner class is defined at the member level of the outer class. It can access all variables and methods of the outer class, including private members.
2.1 Example of a Regular Inner Class
class Car {
private String model = "Toyota";
class Engine {
void startEngine() {
System.out.println("Starting the engine of " + model); // Accessing the outer class’s private variable
}
}
}
public class Main {
public static void main(String[] args) {
Car car = new Car();
Car.Engine engine = car.new Engine();
engine.startEngine(); // Output: Starting the engine of Toyota
}
}
2.2 Key Points
- The regular inner class has access to both the static and non-static members of the outer class.
- You can only instantiate an inner class through an instance of the outer class, as shown in the example above.
3. Static Nested Class
Static nested classes are similar to regular inner classes, but they don’t have access to the outer class's instance variables or methods.
3.1 Example of Static Nested Class
class Computer {
static class Processor {
void display() {
System.out.println("This is a static nested class.");
}
}
}
public class Main {
public static void main(String[] args) {
Computer.Processor processor = new Computer.Processor();
processor.display(); // Output: This is a static nested class.
}
}
3.2 Benefits of Static Nested Classes
- Memory Efficiency: Since static nested classes don’t need an outer class instance, they are more memory-efficient.
- Convenience: You can instantiate a static nested class without creating an instance of the outer class.
4. Local Inner Class
Local inner classes are declared inside a block, typically within a method. They are not visible outside the block where they are defined.
4.1 Example of Local Inner Class
class Book {
void read() {
class Page {
void displayPageNumber(int number) {
System.out.println("Reading page " + number);
}
}
Page page = new Page();
page.displayPageNumber(5); // Output: Reading page 5
}
}
public class Main {
public static void main(String[] args) {
Book book = new Book();
book.read();
}
}
4.2 Key Considerations
- Local inner classes can access variables of the method where they are defined, but these variables must be final or effectively final.
- They are mainly useful when you need a class for one-time use within a method.
5. Anonymous Inner Class
Anonymous inner classes are used when you need to extend a class or implement an interface but don’t want to explicitly declare a class. They are typically used for quick event handling or callbacks.
5.1 Example of Anonymous Inner Class
interface Greeting {
void sayHello();
}
public class Main {
public static void main(String[] args) {
Greeting greeting = new Greeting() {
@Override
public void sayHello() {
System.out.println("Hello from an anonymous inner class!");
}
};
greeting.sayHello(); // Output: Hello from an anonymous inner class!
}
}
5.2 Use Cases
- Event Handling: For instance, you can use anonymous inner classes to handle button clicks in GUI applications.
- Simplifying Code: It can simplify code when you need a small class implementation without cluttering your codebase.
6. Conclusion
Java Inner Classes are a powerful tool for organizing code, especially when different components of your code are closely related. Whether it’s regular inner classes that allow you to access the outer class’s members, or anonymous inner classes that provide quick and flexible implementations, each type of inner class has its specific use case. Understanding when and how to use them will help you write more structured and maintainable code.
If you have any questions about inner classes or need further clarification, feel free to comment below!
Read more at : Secrets of Java Inner Classes: What They Are, Why You Need Them, and How to Use Them Effectively
0
Subscribe to my newsletter
Read articles from Tuanhdotnet directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
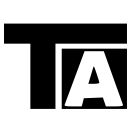
Tuanhdotnet
Tuanhdotnet
I am Tuanh.net. As of 2024, I have accumulated 8 years of experience in backend programming. I am delighted to connect and share my knowledge with everyone.