Jenkins Pipeline Project on AWS EC2 instance
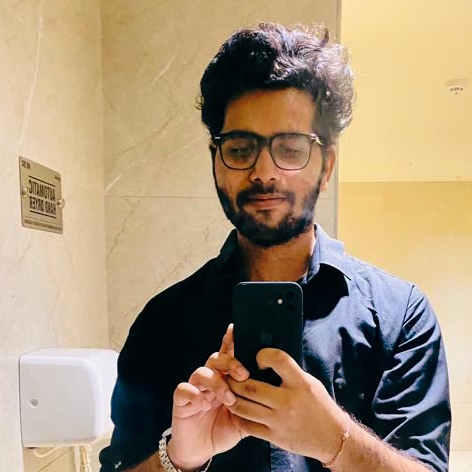

Hey techies! Welcome to another article on Jenkins, where we will create a CI/CD pipeline using Jenkins for a simple project and deploy it on an EC2 instance with an Ubuntu flavor. The project flow will look like this:
EC2—>Setup Jenkins—>Jenkins Pipeline —> Deploy
Pipeline flow would look like this:
Git Checkout —> Build and install the dependency —> Quality Check(SonarQube) —> Deployment.
We will deploying a very static Node.js and html project, you can change the project according to your need
So let’s get started
Create a EC2 instance
So, we will first spin up a EC2 instance where we use our setup our Jenkins server. The below is my configurations
Connecting to EC2 instance
Now we will connect to EC2 instance using SSH key and install the required dependency and before doing let’s enable the PORT 8080 and PORT 3000 to allow the traffic from public networks (not production practice). In production you will use nginx or load balancer , but let’s focus on Jenkins in this tutorial.
ssh command to connect will look like this :
ssh -i "login_cred.pem" ubuntu@ec2->public-ip-address.ap-south-1.compute.amazonaws.com
Make sure you have your login_cred.pem file with you.
Before running the below script run this command to get the root user access.
sudo -i
sudo apt update
sudo apt upgrade -y
#Jenkins installation
sudo apt install -y openjdk-17-jdk
sudo apt install -y wget gnupg
wget -O - https://pkg.jenkins.io/debian/jenkins.io-2023.key | sudo tee /usr/share/keyrings/jenkins-keyring.asc > /dev/null
echo "deb [signed-by=/usr/share/keyrings/jenkins-keyring.asc] https://pkg.jenkins.io/debian binary/" | sudo tee /etc/apt/sources.list.d/jenkins.list > /dev/null
sudo apt update -y
sudo apt install -y jenkins
sudo systemctl start jenkins
sudo systemctl enable jenkins
# Install NVM
curl -o- https://raw.githubusercontent.com/nvm-sh/nvm/v0.39.3/install.sh | bash
# Load nvm properly
export NVM_DIR="$HOME/.nvm"
source "$NVM_DIR/nvm.sh"
# Install Node.js & npm without sudo
nvm install v22.14.0
npm install -g npm@latest
npm install -g pm2
The above config file will install Jenkins and Nodejs for you.
do vim script.sh
copy paste the content and run
sh script.sh
Now all the required tool and dependencies would get installed.
To access the Jenkins Dashboard , use your public ip-address:8080 , install necessary plugins and Jenkins Dashboard will come up like this:
Jenkins Pipeline Setup:
Click on Create a Job
Choose Jenkins Pipeline and give name
Write a pipeline script using Groovy
Jenkins Uses Groovy language to build it’s pipeline.
Now to create any pipeline we will need an agent, we will use and in-built agent agent.
pipeline{ agent any stages{ stage('checkout'){ steps{ git branch: 'main', url: 'https://github.com/Dharansh-Neema/portfolio-dharansh' } } stage('install dependency'){ steps{ sh 'npm install' } } } }
Now we have got the code from repo, we have downloaded the dependency, it time to do the code review. For that purpose we use sonarQube.
SonarQube setup
For sonarQube we need atleast t2.micro, because it require at-least two CPU and change the in-bound rules like this :
Login to sonarQube:
Setup project keys;
Create a token for your project:
Then get the scanner:
We will use this in our pipeline to check the Code-Quality.
Now let’s setup sonarQube to Jenkins:
Pipeline after adding sonarQube:
pipeline {
agent any
environment {
SONARQUBE_URL = "http://35.154.48.121:9000"
SONAR_PROJECT_KEY = "portfolio-dharansh"
SONAR_TOKEN = "sqp_95470a48552794fe674745c0193f81cad032c06e"
}
stages {
stage('Checkout') {
steps {
git branch: 'main', url: 'https://github.com/Dharansh-Neema/portfolio-dharansh.git'
}
}
stage('Install Dependencies') {
steps {
sh 'npm install'
}
}
stage('SonarQube Scan') {
steps {
sh '''
sonar-scanner \
-Dsonar.projectKey=$SONAR_PROJECT_KEY \
-Dsonar.sources=. \
-Dsonar.host.url=$SONARQUBE_URL \
-Dsonar.login=$SONAR_TOKEN
'''
}
}
stage('Build') {
steps {
sh '''
npm run build
'''
}
}
stage('Deploy') {
steps {
sh '''
nvm use 22.14.0
pm2 stop portfolio || true # Stop old process if running
pm2 start npm --name "portfolio" -- run start
pm2 save
'''
}
}
}
}
Deployment stage of pipeline
Now after checking code-quality, we can deploy the code, now my project is based on Nodejs and simple html with tailwind css but if your project requirement and tech-stack is different make sure you have all the required dependency.
pipeline{
agent any
stages{
stage('checkout'){
steps{
git branch: 'main', url: 'https://github.com/Dharansh-Neema/portfolio-dharansh'
}
}
stage('install dependency'){
steps{
sh 'npm install'
}
}
stage('sonarQube'){
steps{
sh '''sonar-scanner \
-Dsonar.projectKey=portfolio-dharansh \
-Dsonar.sources=. \
-Dsonar.host.url=http://35.154.48.121:9000 \
-Dsonar.login=sqp_95470a48552794fe674745c0193f81cad032c06e
'''
}
}
stage('Deploy') {
steps {
sh '''
pm2 stop portfolio || true # Stop old process if running
pm2 start npm --name "portfolio-dharansh" -- run start
pm2 save
'''
}
}
}
Thank you so much for reading and if you are interested in learning about Jenkins Master Slave Architecture Click on below link.
Subscribe to my newsletter
Read articles from Dharansh Neema directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
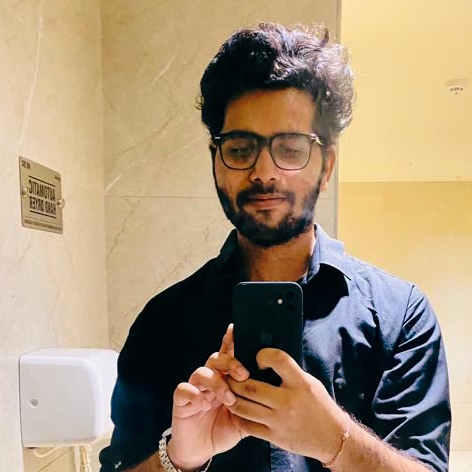