Handling events on multiple items in JavaScript

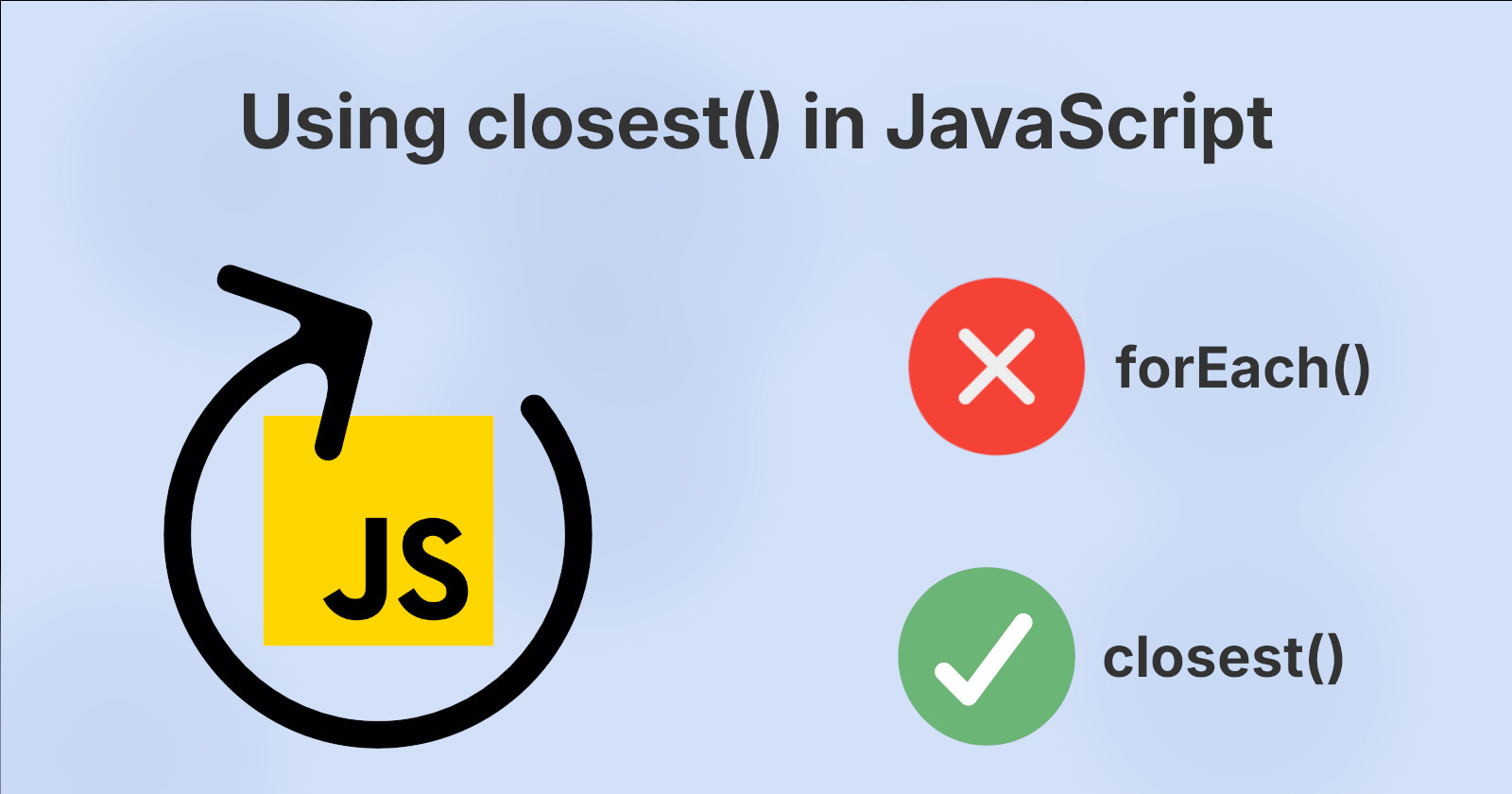
We’ve all been there. You have a list of items and each item needs to listen for an event. Maybe it’s a click event or mouseover event. Traditionally, you may have put an event listener on every item in the list, but what if I told you there was a better, more efficient way of handling this? Introducing the closest() method!
What is the closest() method?
The closest() method traverses up the DOM tree from the target element toward the document root. Imagine it similar to selecting the target element's parentNode continually until it finds what it’s looking for or reaches the document root.
Why is this helpful?
There is no way to guarantee that you will know exactly where an element is in the DOM tree. Sure, you might think that the element you’re looking for is two parentNodes away from the target element. However, what if it was sometimes 3 or 1? How would you handle it if you explicitly selected two parentNodes higher than the target.
The times where closest() comes in most handy is when you want to listen for an event on multiple elements in a list. If you were to place an event listener on every item in the list, then we would have to store that listener in memory for as many items as there are in the list. That may not be a huge deal on 10 elements, but what if it was 100, or 500, or 1000? That’s a lot of functions to store in memory! The closest() method, however, can utilize one single event listener for an indefinite number of elements.
How do I use the closest() method?
Let’s start by creating our list of elements.
<ul id="my-list">
<li class="list-item">Item 1</li>
<li class="list-item">Item 2</li>
<li class="list-item">Item 3</li>
</ul>
As you can see, we have an unordered list with an id of “my-list” and three list items with classes of “list-item”. If we wanted to listen for a click event on each of these elements without using closest(), it could look something like this.
document.querySelectorAll(".list-item").forEach((item) => {
item.addEventListener("click", function () {
if (!item.classList.contains("complete")) {
item.classList.add("complete");
return;
}
item.classList.remove("complete");
});
});
You can see how cumbersome this becomes to read, not to mention storing each of these event listeners in memory. We loop over each item in the list, add an event listener to that item, and then add or remove the “complete” class when clicked.
Now lets implement this with the closest() method.
const list = document.getElementById("my-list");
list.addEventListener("click", function(e) {
const item = e.target.closest(".list-item");
if (!item) {
console.log("Could not find an element with the class 'list-item'");
return;
}
if (!item.classList.contains("complete")) {
item.classList.add("complete");
return;
}
item.classList.remove("complete");
});
What’s happening here is that when you click anywhere in the unordered list, goes up the DOM tree in search of an element with the class “list-item”. If it gets to the document root without finding an element with the class “list-item”, then it logs a message to the console and returns out of the function. However, if it does find an element with the class “list-item”, then it checks if it’s classList contains the class “complete” and adds or removes it depending on it’s classList.
Now we have only one event listener for the whole list regardless of how many items are in this list. I hope you can see the benefit and the power behind using closest() instead of an event listener on each element in the list!
Subscribe to my newsletter
Read articles from Brad Preston directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Brad Preston
Brad Preston
I'm a software developer with a background in Golang and JS/TS