Serialization and Deserialization: Teleporting a Human from Toronto to New York

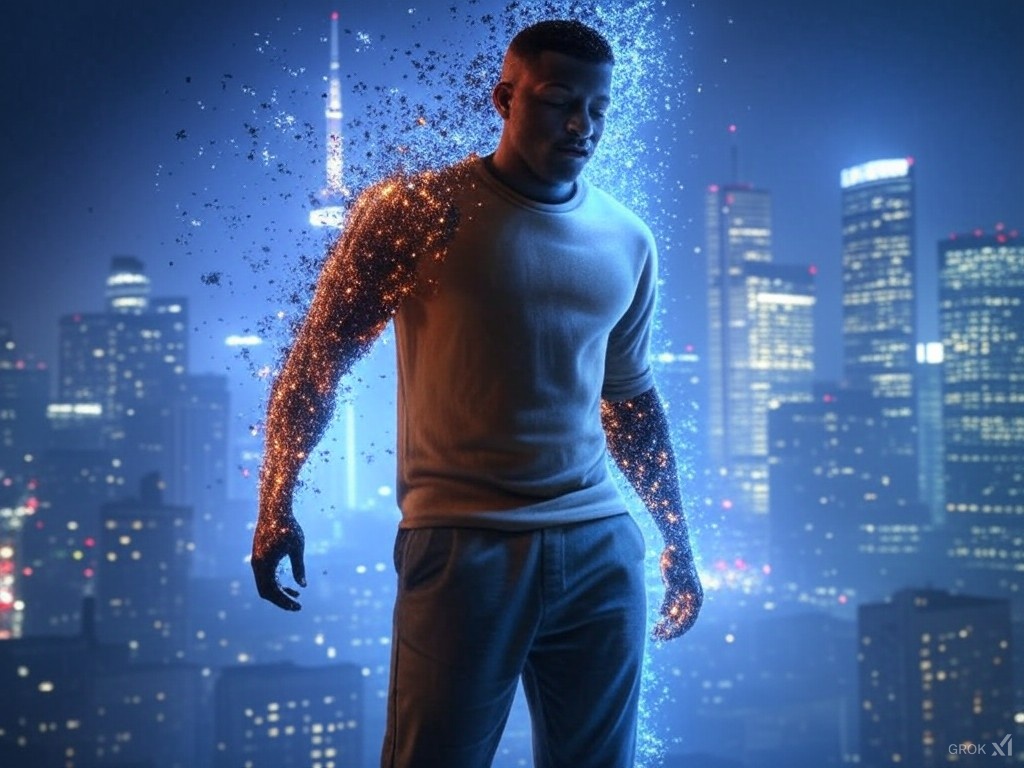
Ever since I was a kid, I’ve been fascinated by futuristic sci-fi movies where teleportation is as common as WiFi. Imagine a world where you could instantly zap yourself from Toronto to New York! ⚡️
While human teleportation remains a mystery (at least in 2025), we do have a somewhat similar concept in the programming world: serialization and deserialization. Just like breaking down a person into data and reconstructing them elsewhere (theoretically, of course), JavaScript allows us to convert complex data structures into a transmittable format (serialization) and later rebuild them back into their original form (deserialization).
We may not be able to teleport people (yet!), but understanding serialization in JavaScript gets us one step closer to making data travel seamlessly across systems. So, let’s dive into how this works and why it’s such a powerful concept!
Serialization (with JSON)
As we touched on earlier, serialization is the process of converting complex data structures—such as objects or arrays—into a format that can be easily stored or transmitted. The most commonly used format for this in JavaScript is JSON (JavaScript Object Notation).
Now, let’s bring back our teleportation analogy. If I wanted to teleport myself from Toronto to New York, I couldn’t just walk into a computer. Instead, I’d need to convert myself into a data-friendly format—essentially breaking myself down into structured information that a system can process. Once in this format, I could be sent across the internet and reconstructed at my destination.
In JavaScript, we serialize objects into JSON using the JSON.stringify()
method. Here’s how it works:
const user = {
name: "Sartaj",
age: 25,
height: 180
};
const serializedString = JSON.stringify(user);
console.log(serializedString); // Output: {"name":"Sartaj","age":25,"height":180}
Now, Sartaj is no longer just a person—I’m a JSON string, ready to be transmitted anywhere!
Serialization is crucial for sending data between systems, saving it in files, or storing it in databases. But teleporting data is only half the journey. Once it reaches the other side, we need a way to bring it back to its original form—and that’s where deserialization comes in.
Deserialization (with JSON)
Deserialization is the process of converting serialized data back into its original structure, allowing programs to interact with it as native JavaScript objects.
Let’s revisit our teleportation analogy. We already converted ourselves into a data format and transmitted it across the network from Toronto to New York. But what good is a string of data if it can’t be reconstructed? To bring me back to my physical form, someone in New York would need to parse my data and reconstruct me properly.
In JavaScript, this is done using JSON.parse()
:
const jsonString = '{"name":"Sartaj","age":25,"height":180}';
const deserializedString = JSON.parse(jsonString);
console.log(deserializedString.name); // Output: Sartaj
Now, I’m no longer just a string—I’m back as a fully structured object!
Deserialization is essential whenever data is received in JSON format, such as when fetching data from an API, reading from a file, or receiving messages from a server. Just like in teleportation, if we can’t reconstruct the data correctly, it’s useless!
Alternative Serialization Formats
While JSON is the go-to choice for many developers due to its simplicity and readability, it's not the only serialization format out there. Depending on the use case, other formats might offer better performance, more flexibility, or additional features. Let’s explore some popular alternatives:
BSON (Binary JSON): A binary-encoded extension of JSON that supports additional data types like dates and raw binary data. It's commonly used in MongoDB to store documents efficiently.
XML (eXtensible Markup Language): A structured, human-readable format used to encode documents with hierarchical data. It’s widely used in web services, configuration files, and legacy systems.
YAML (YAML Ain't Markup Language): A human-friendly, indentation-based data format that’s great for configuration files (e.g., Kubernetes, Docker, and CI/CD pipelines). It's often preferred over JSON when readability is a priority.
FlatBuffers: Developed by Google, this ultra-fast serialization library allows reading and writing data without unpacking or parsing. It’s perfect for high-performance applications like game engines, real-time applications, and embedded systems.
Each of these formats shines in different scenarios—choosing the right one depends on your project’s needs, performance constraints, and readability requirements.
Challenges in Serialization and Deserialization
While serialization makes it easy to transmit and store data, it's not always smooth sailing. Here are some common challenges that developers face:
Data Loss: Not all data types are natively supported by JSON. For example, functions,
undefined
, andSymbol
values are omitted during serialization, potentially leading to lost information. If you need to store functions or special values, you might need custom serialization techniques.Data Corruption: Improper handling during serialization or deserialization can lead to corrupted data, especially with complex or large datasets. This can happen due to network issues, incorrect parsing, or incompatible data formats.
Format Incompatibility: Different systems may use different serialization formats (e.g., JSON vs. XML). If a system expects JSON but receives XML, it might fail to parse the data correctly, leading to communication issues between applications.
Understanding these challenges helps developers choose the right serialization format and apply techniques like data validation, error handling and format consistency to ensure data integrity.
Best Practices to Mitigate Serialization Challenges
To ensure smooth and error-free serialization and deserialization, follow these best practices:
Data Validation: Before deserializing, always validate incoming data to ensure it meets the expected format and structure. This helps prevent data corruption, security vulnerabilities, and unexpected crashes.
Error Handling: Implement robust try-catch mechanisms to handle serialization and deserialization errors gracefully. Unexpected data formats or corrupted payloads can cause failures, so having proper error management is crucial.
Format Consistency: Ensure both the sender and receiver agree on the data format to avoid incompatibility issues. Sticking to widely accepted formats like JSON, BSON, or Protocol Buffers improves data exchange across different systems.
By following these best practices, you can minimize risks, improve reliability, and enhance data integrity in your applications!
Wrapping It Up
Serialization and deserialization are the unsung heroes of modern data exchange, allowing information to travel seamlessly across systems, databases, and networks. Whether you're sending data between applications, storing structured information, or optimizing performance, understanding these concepts is essential for every developer.
While JSON is the most popular choice, exploring alternative formats like BSON, XML, YAML, and FlatBuffers can help you pick the right tool for the job. At the same time, being aware of common challenges and following best practices—such as data validation, error handling, and format consistency—ensures your serialized data remains reliable and secure.
So next time you’re dealing with data transmission, just think of it as a mini teleportation experiment—breaking things down, sending them across, and reconstructing them on the other side. Master this process, and you’ll be well on your way to building efficient, scalable, and error-free applications!
Subscribe to my newsletter
Read articles from Sartaj Singh directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
