Techniques for Using Repeat Annotation in Java: Unlocking Its Power with Code Examples and Demos
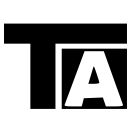
5 min read
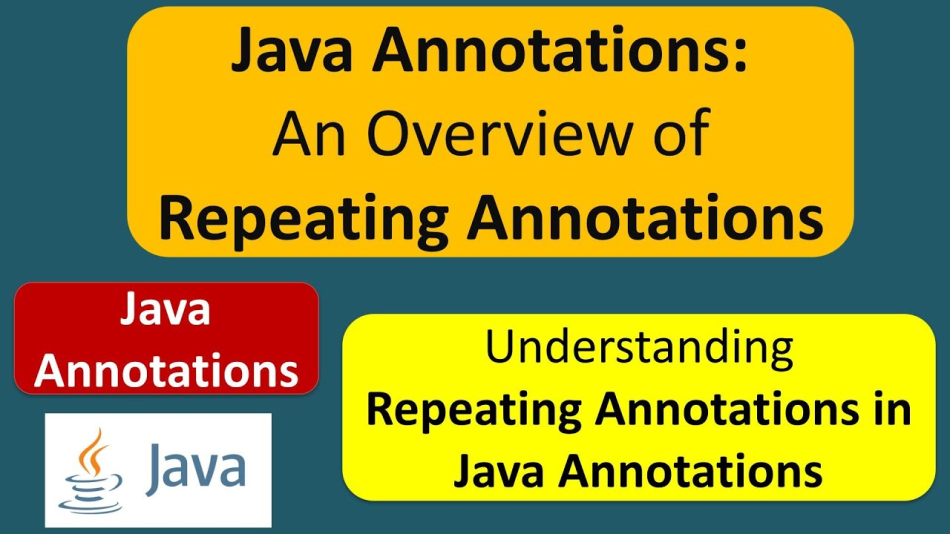
Source: Techniques for Using Repeat Annotation in Java: Unlocking Its Power with Code Examples and Demos
1. Understanding Repeatable Annotations in Java
Repeat annotations offer a way to apply the same annotation to a target multiple times. Before Java 8, this was not possible, which often resulted in developers using workaround methods like arrays to achieve similar results. Java 8 fixed this limitation by introducing the @Repeatable meta-annotation.
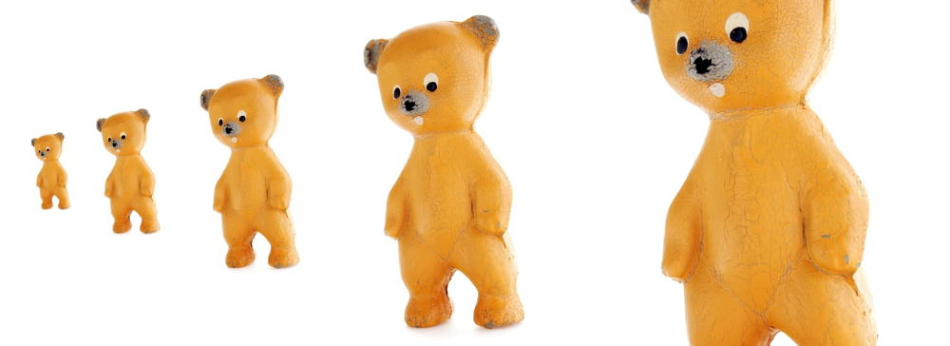
1.1 What Are Repeatable Annotations?
Repeatable annotations allow you to annotate a particular class, method, or other element multiple times with the same annotation. These annotations are wrapped in a container annotation behind the scenes, which makes them manageable.
For example, let’s say you have an annotation called @Role that you want to apply multiple times to the same class. Prior to Java 8, you couldn’t directly annotate a class with @Role more than once. Now, with repeatable annotations, you can achieve this easily.
import java.lang.annotation.ElementType;
import java.lang.annotation.Repeatable;
import java.lang.annotation.Retention;
import java.lang.annotation.RetentionPolicy;
import java.lang.annotation.Target;
@Target(ElementType.TYPE)
@Retention(RetentionPolicy.RUNTIME)
@Repeatable(Roles.class)
@interface Role {
String value();
}
@Target(ElementType.TYPE)
@Retention(RetentionPolicy.RUNTIME)
@interface Roles {
Role[] value();
}
In the example above, the @Role annotation is repeatable, and the @Roles annotation acts as a container for multiple instances of @Role.
1.2 Why Use Repeatable Annotations?
The key benefit of repeatable annotations lies in simplifying your code when multiple annotations are required. Without this feature, developers often had to manage arrays of annotations, which were less intuitive and more prone to errors.
In scenarios such as specifying multiple roles for users, managing configurations, or handling specific constraints, repeatable annotations can clean up the code by avoiding clunky arrays.
1.3 A Basic Example
Let’s walk through a basic example where we apply repeatable annotations to a class representing a user with multiple roles.
@Role("Admin")
@Role("User")
public class User {
}
In this example, the User class has two roles: "Admin" and "User." Behind the scenes, Java aggregates these annotations using the container annotation @Roles.
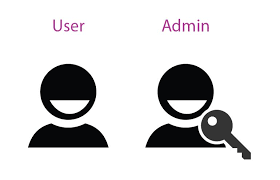
1.4 Accessing Repeat Annotations
At runtime, you may want to retrieve all instances of a repeatable annotation. Java provides the getAnnotationsByType() method for this purpose.
import java.util.Arrays;
public class RepeatableExample {
public static void main(String[] args) {
Role[] roles = User.class.getAnnotationsByType(Role.class);
Arrays.stream(roles).forEach(role -> System.out.println("Role: " + role.value()));
}
}
When you run this example, it will print:
Role: Admin
Role: User
2. Real-World Applications of Repeat Annotations
Now that we understand the basics of repeatable annotations, let’s look at some practical applications where this feature can be particularly useful.
2.1 Applying Multiple Constraints
In enterprise applications, you often need to apply multiple constraints to a method or class. For example, you might want to annotate a method with multiple validation constraints such as @Size, @Min, or @Max. With repeatable annotations, this is now much simpler to manage.
@Size(min = 5)
@Size(max = 10)
public String validateName(String name) {
return name;
}
2.2 Managing Security Roles
One of the most common real-world scenarios for repeatable annotations is managing security roles in applications. When different parts of an application require multiple roles, you can use repeatable annotations to streamline role assignments.
@Role("Admin")
@Role("Manager")
public class AdminPanel {
// Business logic here
}
Here, the AdminPanel requires both the "Admin" and "Manager" roles. This approach improves code readability and ensures that roles are clearly documented in the code.
2.3 Simplifying Configurations
Another useful scenario is when managing configurations. Suppose you need to apply multiple configuration settings to a class or method. Instead of creating complex configuration objects, you can use repeatable annotations to keep things clean and readable.
@Config(setting = "debug", value = "true")
@Config(setting = "timeout", value = "5000")
public class ConfigManager {
// Configuration logic
}
This makes it easy to manage configurations by simply annotating the class or method multiple times with different settings.
3. Demo: Putting Repeat Annotations into Practice
Let’s run a complete demo using repeatable annotations. We will create a system where users are assigned multiple roles, and we’ll access those roles at runtime.
3.1 Defining the Annotations
import java.lang.annotation.ElementType;
import java.lang.annotation.Repeatable;
import java.lang.annotation.Retention;
import java.lang.annotation.RetentionPolicy;
import java.lang.annotation.Target;
@Target(ElementType.TYPE)
@Retention(RetentionPolicy.RUNTIME)
@Repeatable(Roles.class)
@interface Role {
String value();
}
@Target(ElementType.TYPE)
@Retention(RetentionPolicy.RUNTIME)
@interface Roles {
Role[] value();
}
3.2 Applying Repeatable Annotations
@Role("Admin")
@Role("User")
@Role("Manager")
public class User {
}
3.3 Accessing the Annotations at Runtime
import java.util.Arrays;
public class RepeatableDemo {
public static void main(String[] args) {
Role[] roles = User.class.getAnnotationsByType(Role.class);
System.out.println("Assigned Roles:");
Arrays.stream(roles).forEach(role -> System.out.println(role.value()));
}
}
3.4 Demo Output
When running the above code, the output will be:
Assigned Roles:
Admin
User
Manager
As demonstrated, repeatable annotations simplify the process of applying multiple roles and improve code readability.
4. Conclusion
Repeatable annotations in Java are an essential tool for developers looking to streamline their code when multiple instances of the same annotation are required. Whether you're working with roles, constraints, or configurations, using repeatable annotations makes your code more intuitive and less error-prone.
Now that you've seen the power of repeat annotations in action, you can start implementing them in your own projects. If you have any questions or would like to share your experience with repeatable annotations, feel free to leave a comment below!
Read more at : Techniques for Using Repeat Annotation in Java: Unlocking Its Power with Code Examples and Demos
0
Subscribe to my newsletter
Read articles from Tuanhdotnet directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
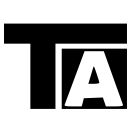
Tuanhdotnet
Tuanhdotnet
I am Tuanh.net. As of 2024, I have accumulated 8 years of experience in backend programming. I am delighted to connect and share my knowledge with everyone.