How Vector Search is Changing the Game for AI-Powered Discovery
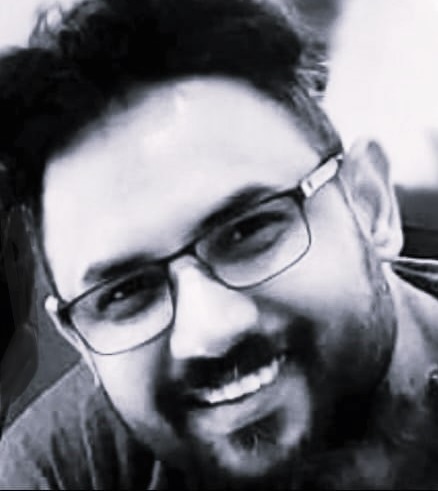
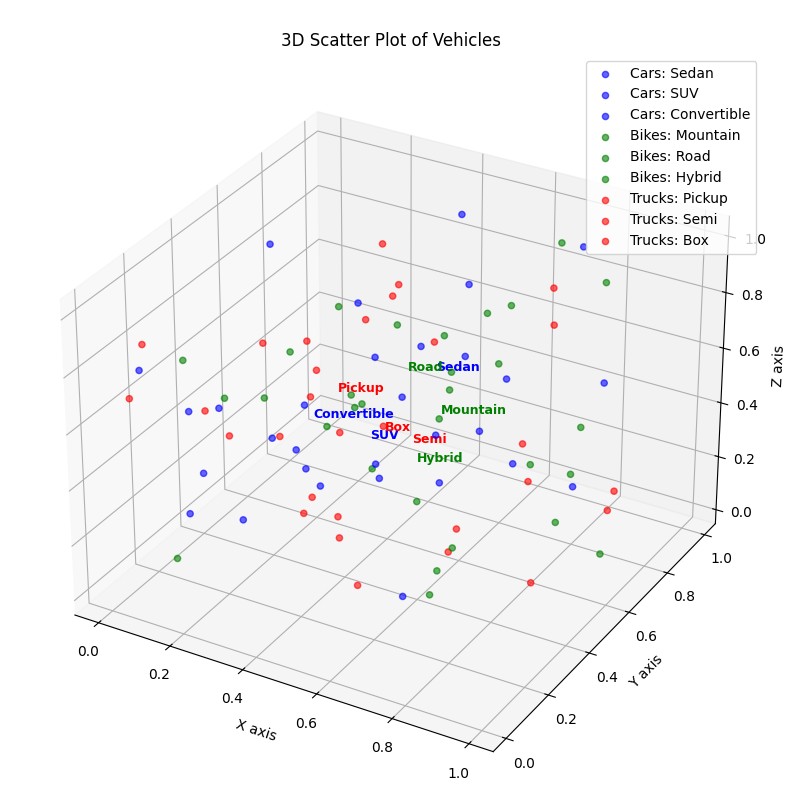
The Problem with “Dumb” Search
Early in my career, I built a recipe recommendation app that matched keywords like “chicken” to recipes containing “chicken.” It failed spectacularly. Users searching for “quick weeknight meals” didn’t care about keywords — they wanted context: meals under 30 minutes, minimal cleanup, kid-friendly. Traditional search couldn’t bridge that gap.
Vector search changes this. Instead of treating data as strings, it maps everything — text, images, user behavior — into numerical vectors that capture meaning. For example, “quick weeknight meals,” “30-minute dinners,” and “easy family recipes” cluster closely in vector space, even with zero overlapping keywords. This is how AI starts to “think” like us .
What This Article Is About
This article is my try to dives into how vector search is revolutionizing AI’s ability to discover patterns, relationships, and insights at unprecedented speed and precision. By moving beyond rigid keyword matching, vector search enables machines to understand context, infer intent, and retrieve results with human-like intuition. Through Python code examples, system design diagrams, and industry use cases (like accelerating drug discovery and personalizing content feeds), we’ll explore how this technology makes AI systems faster, more adaptable.
Why Read It?
For Developers: Build lightning-fast search systems using modern tools like FAISS and Hugging Face, with optimizations for real-world latency and scale.
For Business Leaders: Discover how vector search drives competitive advantages in customer experience, fraud detection, and dynamic pricing.
For Innovators: Learn why hybrid architectures and multimodal AI are the future of intelligent systems.
Bonus: Lessons from my own journey deploying vector search — including costly mistakes and unexpected breakthroughs.
So, What Vector Search Really is ?
Imagine you’re in a music store. Instead of searching for songs by title (like “Bohemian Rhapsody”), you hum a tune. The clerk matches your hum to songs with similar melodic patterns, even if they’re in different genres. Vector search works the same way: it finds data based on semantic patterns, not exact keywords.
Vector search maps data (text, images, etc.) into high-dimensional numerical vectors. Similarity is measured using distance metrics (e.g., cosine similarity).
Use below code to understand vector space in a very simpler way
import matplotlib.pyplot as plt
import numpy as np
# Mock embeddings: [sweetness, crunchiness]
fruits = {
"Apple": [0.9, 0.8],
"Banana": [0.95, 0.2],
"Carrot": [0.3, 0.95],
"Grapes": [0.85, 0.1]
}
# Plotting
plt.figure(figsize=(8, 6))
for fruit, vec in fruits.items():
plt.scatter(vec[0], vec[1], label=fruit)
plt.xlabel("Sweetness →"), plt.ylabel("Crunchiness →")
plt.title("Fruit Vector Space")
plt.legend()
plt.grid(True)
plt.show()
Banana and Grapes cluster near high sweetness, while Carrot stands out with crunchiness.
Can We Implement Vector Search Ourselves?
Yes! Let’s build a minimal vector search engine using pure Python:
import numpy as np
from collections import defaultdict
class VectorSearch:
def __init__(self):
self.index = defaultdict(list)
def add_vector(self, id: int, vector: list):
self.index[id] = np.array(vector)
def search(self, query_vec: list, k=3):
query = np.array(query_vec)
distances = {}
for id, vec in self.index.items():
# Euclidean distance
distances[id] = np.linalg.norm(vec - query)
# Return top K closest
return sorted(distances.items(), key=lambda x: x[1])[:k]
# Example usage
engine = VectorSearch()
engine.add_vector(1, [0.9, 0.8]) # Apple
engine.add_vector(2, [0.95, 0.2]) # Banana
engine.add_vector(3, [0.3, 0.95]) # Carrot
query = [0.88, 0.15] # Sweet, not crunchy
results = engine.search(query, k=2)
print(f"Top matches: {results}") # Output: [(2, 0.07), (1, 0.15)] → Banana, Apple
Key Limitations:
Brute-force search (O(n) time) — impractical for large datasets.
No dimensionality reduction or indexing.
Subscribe to my newsletter
Read articles from Aniket Hingane directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
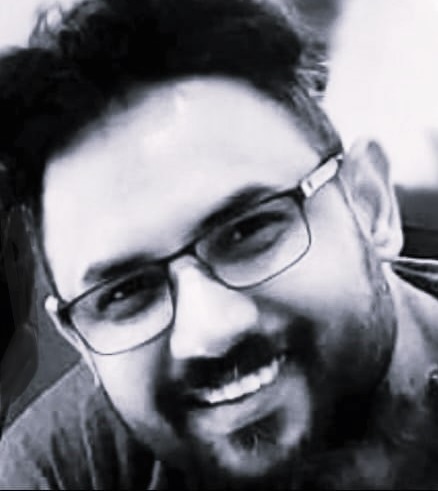