Building an Automated Anime News Feed with AWS EventBridge and Slack

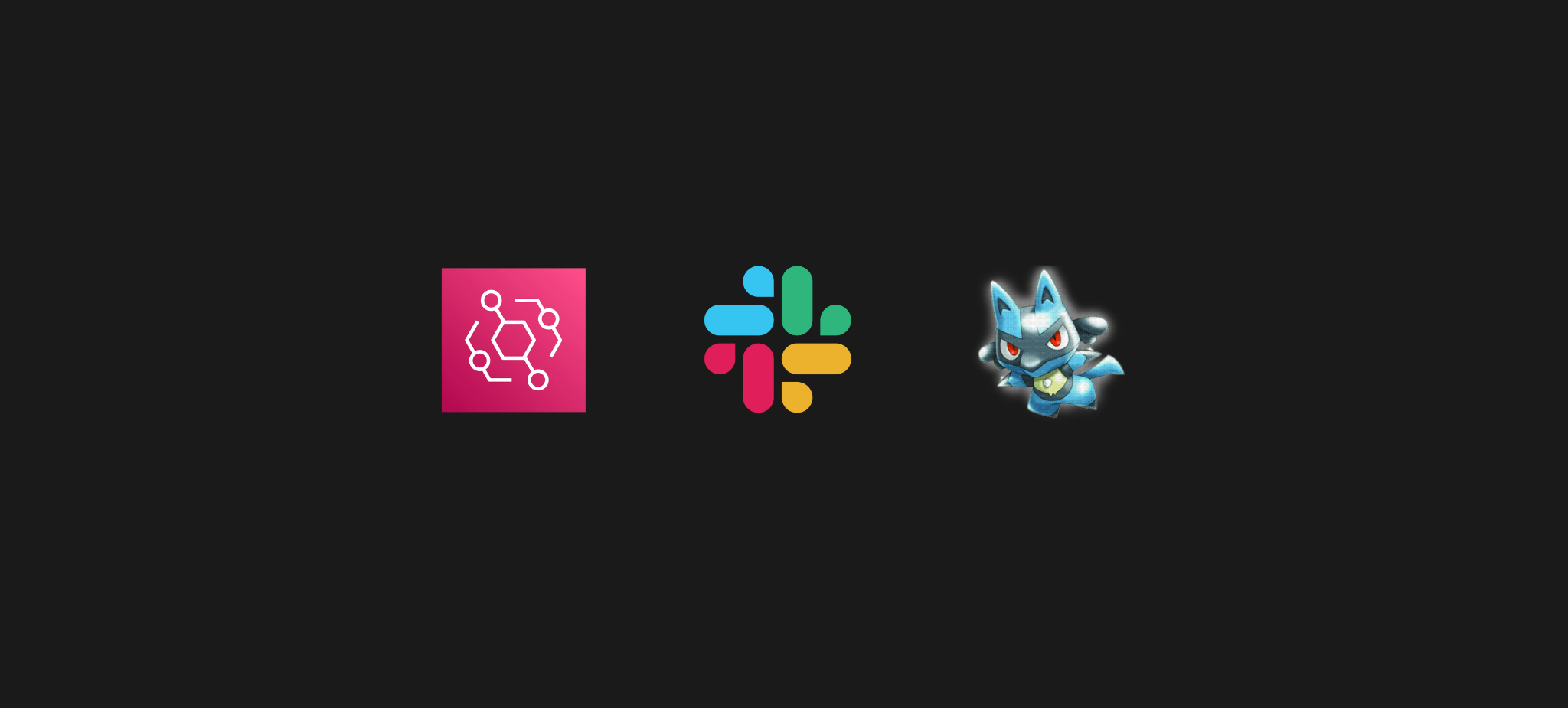
Do you like anime? Well, I love it, and like many other topics, there are usually interesting news worth reading with a cup of coffee to start the morning. However, since there are many news items per day, I was thinking that instead of going to look for them on a trusted blog, I could receive them as a Slack notification daily (although it could also be Discord or others).
Join me to see how I spent some time doing what we like most: automating something we can do daily in 1 minute.
For this we need:
An Amazon AWS account
A Slack account
A reliable source of anime news (in my case I'll use Crunchyroll)
Solution Architecture
Before starting to write code, let me tell you how I thought about this solution. The idea is quite simple: we need something that periodically checks the news and sends it to Slack. But why did I choose these specific AWS services?
The Data Flow
Here's how a news item flows through the system:
EventBridge acts as our alarm clock, triggering every morning at 10 AM
This "alarm clock" triggers our Lambda function
Lambda does the heavy lifting:
Connects to Crunchyroll's RSS to get the latest news
Processes the information and formats it nicely
Sends everything to Slack through a webhook
What is AWS Lambda?
Lambda is like having a small program that sleeps until you need it. The great thing is:
You only pay when it runs (and the free tier is super generous)
No need to worry about servers or infrastructure
It can handle everything from simple tasks to complex processes
It integrates perfectly with other AWS services
In our case, Lambda is perfect because:
The task is relatively simple and quick
We don't need a server running 24/7
RSS processing and sending to Slack takes seconds
What is AWS SAM?
SAM is like having a personal assistant that handles all the tedious parts of configuring AWS services. Instead of clicking around in the AWS console:
We write everything in a YAML file
SAM takes care of creating and configuring everything needed
Makes development and deployment much simpler
If something goes wrong, it's easy to destroy everything and start over
What is AWS EventBridge and why use it?
EventBridge is the service that makes all of this automatic. It's like having a cron job in the cloud, but on steroids:
Can schedule tasks with second-level precision
Natively integrates with Lambda
Highly reliable (you won't miss news because the server went down)
Allows schedule modifications without touching the code
In our case, we configure it to run every day at 10 AM, but we could easily change it to run every hour or even every 5 minutes if we wanted to stay super updated.
Why this architecture?
You might ask yourself, why not use a simple script on a server? Well, this architecture has several advantages:
Zero-maintenance: No need to worry about operating system updates or dependencies
Scalable: If we wanted to add more news sources or Slack channels, it's trivial
Cost-efficient: With the free tier, this will probably be free for a good while
Reliable: AWS ensures everything works, you only worry about the code
Getting Started
1. Project Structure
Our project will have this structure:
eventbridge-with-sam/
├── src/
│ ├── helpers/
│ │ ├── slack.helper.ts
│ │ ├── xml.helper.ts
│ ├── services/
│ │ └── news.service.ts
│ ├── tasks/
│ │ └── fetch-news.ts
│ ├── interfaces/
│ │ └── news.ts
├── template.yml
└── package.json
2. Code Implementation
Let's go step by step, explaining each part of the code and why we structured it this way. First, our Slack helper (slack.helper.ts
). This will handle all communication with Slack:
import axios from 'axios';
export class SlackHelper {
// Sends formatted messages to Slack using webhook
static async sendMessage(message: string) {
try {
await axios.post(process.env.SLACK_WEBHOOK_URL!, { text: message });
console.log('Message sent to Slack:', message);
} catch (error) {
console.error('Error sending message to Slack:', error);
throw error;
}
}
}
Now, we need a helper to handle the RSS XML (xml.helper.ts):
import { parseStringPromise } from 'xml2js';
export class XMLHelper {
// Parses XML data into a structured format
static async parseXML(xml: string) {
try {
return await parseStringPromise(xml);
} catch (error) {
console.error('Error parsing XML:', error);
throw error;
}
}
}
To keep our code well-typed, we define the interface for our news:
export interface AnimeNewsItem {
title: string;
link: string;
}
The main service (news.service.ts) is where the magic happens. This is where we fetch the news and process it:
import axios from 'axios';
import { SlackHelper } from '../helper/slack.helper';
import { XMLHelper } from '../helper/xml.helper';
import { AnimeNewsItem } from '../interfaces/news';
export class NewsService {
// Main function to fetch and process news
async fetchAndSendNews() {
try {
// Fetch RSS feed
const response = await axios.get(process.env.CRUNCHYROLL_RSS_URL!);
const xmlData = response.data;
// Parse XML to JSON
const newsJson = await XMLHelper.parseXML(xmlData);
const rawItems = newsJson.rss.channel[0].item;
if (!rawItems || rawItems.length === 0) {
console.log('No news found for today.');
return;
}
// Transform raw items into our NewsItem format
const items: AnimeNewsItem[] = rawItems.map((news: any) => ({
title: news.title[0],
link: news.link[0],
}));
// Format message for Slack
let message = `📰 *Latest News Update:*\n\n`;
items.forEach((news) => {
message += `🔹 *${news.title}*\n🔗 ${news.link}\n\n`;
});
await SlackHelper.sendMessage(message);
} catch (error) {
console.error('Error in news processing:', error);
throw error;
}
}
}
Also, we add our Lambda task (fetch-news.ts
) which will be the entry point when EventBridge invokes it:
import { EventBridgeEvent } from 'aws-lambda';
import { NewsService } from '../services/news.service';
export const fetchAndSendNews = async (event: EventBridgeEvent<'Scheduled Event', {}>) => {
console.log('[Scheduled Task] Fetching news...');
await new NewsService().fetchAndSendNews();
console.log('[Scheduled Task] News sent.');
};
Finally, our configuration file (template.yml) that defines all the infrastructure:
AWSTemplateFormatVersion: 2010-09-09
Description: >-
news-automation
Transform:
- AWS::Serverless-2016-10-31
Globals:
Api:
Cors:
AllowMethods: "'*'"
AllowHeaders: "'*'"
AllowOrigin: "'*'"
Function:
CodeUri: ./dist
Runtime: nodejs18.x
MemorySize: 128
Timeout: 10
AutoPublishAlias: Live
Environment:
Variables:
CRUNCHYROLL_RSS_URL: !Sub '{{resolve:ssm:/services/shared/CRUNCHYROLL_RSS_URL}}'
SLACK_WEBHOOK_URL: !Sub '{{resolve:ssm:/services/shared/SLACK_WEBHOOK_URL}}'
Resources:
fetchAndSendNews:
Type: AWS::Serverless::Function
Properties:
Handler: tasks/fetch-news.fetchAndSendNews
Description: Fetch news from RSS feed and send to Slack
MemorySize: 300
Timeout: 900
Events:
DailyTrigger:
Type: Schedule
Properties:
Schedule: cron(0 10 * * ? *)
Enabled: true
Description: Runs every day at 10:00 AM UTC
The cron expression 0 10 * * ? *
will run our function every day at 10:00 AM UTC. Here's the breakdown of the expression:
Minute | Hour | Day of Month | Month | Day of Week | Year (optional) |
0 | 10 | * | * | ? | * |
Additionally, for the template to work, we need to configure some additional resources. First, we'll create the parameters in AWS Systems Manager Parameter Store (SSM):
# Create parameters in SSM
aws ssm put-parameter \
--name "/services/shared/CRUNCHYROLL_RSS_URL" \
--value "https://www.crunchyroll.com/newsrss" \
--type "String"
aws ssm put-parameter \
--name "/services/shared/SLACK_WEBHOOK_URL" \
--value "your-slack-webhook-url" \
--type "SecureString"
We also need a samconfig.toml
file for deployment configuration:
version = 0.1
[dev]
[dev.deploy]
[dev.deploy.parameters]
stack_name = "eventbridge-with-sam"
s3_bucket = "your-deployment-bucket"
s3_prefix = "eventbridge-with-sam"
region = "us-west-2"
confirm_changeset = true
capabilities = "CAPABILITY_IAM"
parameter_overrides = "Env=dev"
image_repositories = []
3. Slack Configuration
Now comes the fun part - we'll configure Slack to receive our news. Whether you're familiar with creating Slack apps or not, here's a step-by-step guide to get you started:
- First, go to api.slack.com/apps and click on "Create New App":
- See that green "Create New App" button? Click it and select "From Scratch":
- Now you need to give your app a name - I called mine "AnimeNewsBot" and added it to my "Codeanding" workspace:
Once the app is created, go to "Incoming Webhooks" in the side menu and activate the option:
Click on "Add New Webhook to Workspace"
Select the channel where you want to receive the news
Save the webhook URL that Slack provides, we'll need it later!
Your configured webhook should look something like this:
4. Deployment
Now comes the exciting part. Let's deploy our application step by step:
- First, make sure you have all dependencies installed:
yarn install
- Build the project (this transpiles TypeScript to JavaScript):
yarn build
- Deploy the application:
yarn deploy
During deployment, SAM will:
Create an S3 bucket if it doesn't exist
Package your code
Create/update resources in AWS
Show you a summary of changes
If everything goes well, you should see something like this in the terminal:
Successfully created/updated stack - eventbridge-with-sam
To verify that everything works, wait until the next scheduled time (10:00 AM UTC), and you should see the news appear in your Slack channel:
5. Resource Cleanup
When you want to remove everything created (to avoid charges or just clean up), run these commands:
# Delete the CloudFormation stack
sam delete --stack-name eventbridge-with-sam
# Delete SSM parameters
aws ssm delete-parameter --name "/services/shared/CRUNCHYROLL_RSS_URL"
aws ssm delete-parameter --name "/services/shared/SLACK_WEBHOOK_URL"
Ideas for Improvement
Liked the project? Great! But there's always room for improvement. Some ideas if you want to keep practicing:
More messaging platforms: Why stick with just Slack? You could add support for Discord or Telegram. Each platform has its own API and would be an excellent abstraction exercise.
Better messages: Current messages are functional, but you could make them more attractive. How about adding news preview images or using Slack blocks for a more elaborate format?
Custom filters: Interested in only certain types of news? You could add filters by keyword or categories. You could even allow each user to configure their own filters.
On-demand commands: Sometimes you don't want to wait for the daily update. How about adding a
/news
command to get the latest news whenever you want?
If you implement any of these improvements or come up with another interesting idea, don't hesitate to make a PR to the repo! It would be interesting to see what happens.
Source Code
Want to see the complete code, or contribute? Find it on GitHub! EventBridge with SAM
Common Troubleshooting
Error: "Cannot find webhook URL"
Verify that you've correctly configured the SLACK_WEBHOOK_URL environment variable
Make sure the webhook is active in Slack
Not receiving messages in Slack
Verify that the webhook has permissions to post in the channel
Check CloudWatch logs for errors
I hope you found this guide helpful! If you have any questions or suggestions, please leave your comments below - I'd love to hear your thoughts and experiences.
Until the next post, let's keep coding and learning together!
Subscribe to my newsletter
Read articles from Julissa Rodriguez directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
