Decoding Django ORM: Mastering aggregate() and annotate()

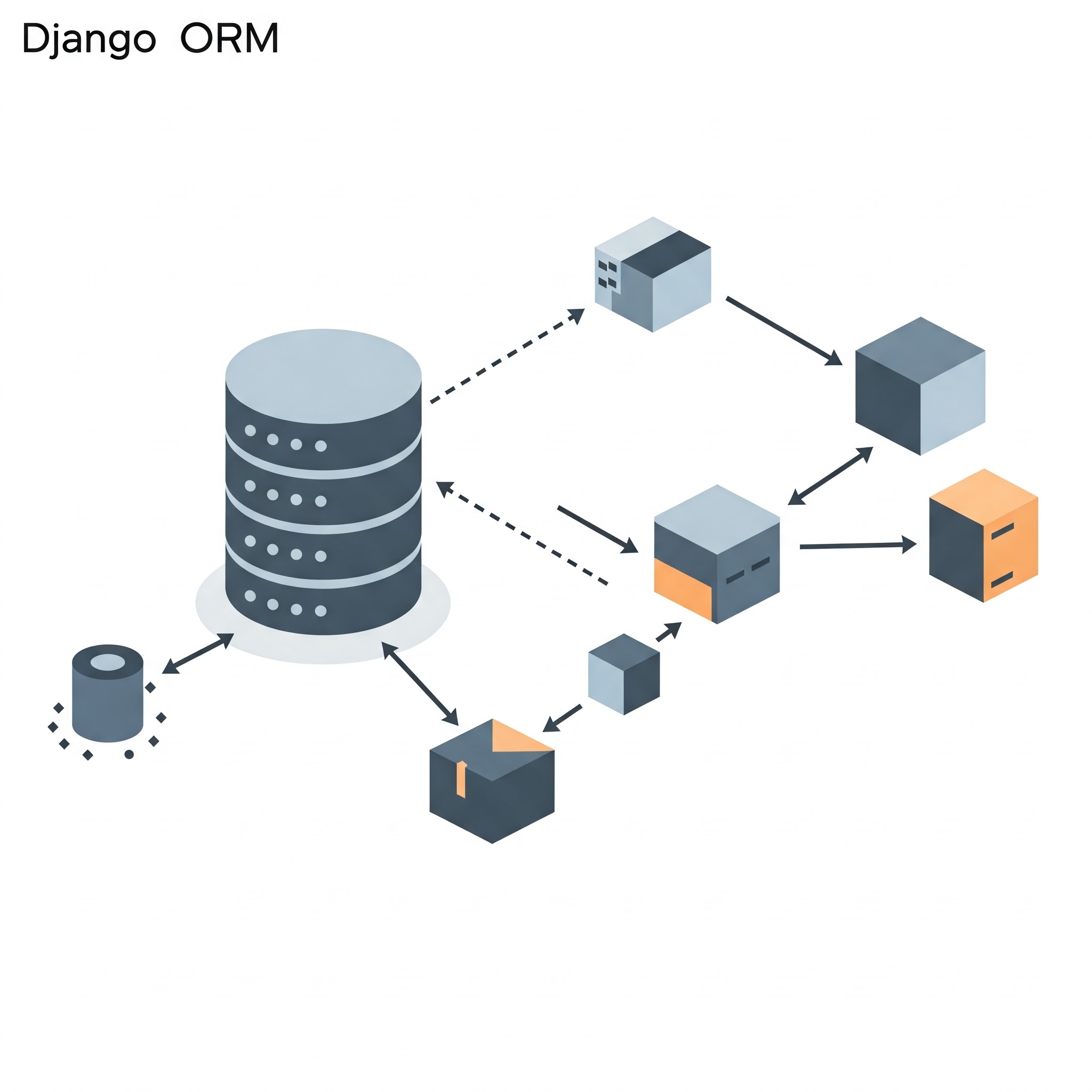
Django's ORM (Object-Relational Mapper) is a powerful tool for interacting with your database. Two particularly useful, but sometimes confusing, methods are aggregate()
and annotate()
. Both allow you to perform calculations on your data, but they differ significantly in their approach and output. Understanding the nuances of each is key to writing efficient and effective Django queries.
This post will demystify aggregate()
and annotate()
with clear explanations and practical code examples.
The Core Difference: Summarization vs. Augmentation
At their heart, the difference between aggregate()
and annotate()
lies in what they do with your data:
aggregate()
: Think ofaggregate()
as your data summarizer. It performs calculations across your entire QuerySet and returns a single dictionary containing the aggregate values. It boils down your data into key metrics.annotate()
:annotate()
is your data augmentor. It performs calculations on each individual object in your QuerySet and adds the result as a new field to that object. It enriches your objects with calculated information.
Deep Dive with Examples
Let's illustrate with a scenario. Imagine you have an e-commerce platform with Author
and Book
models:
Python
from django.db import models
class Author(models.Model):
name = models.CharField(max_length=200)
class Book(models.Model):
title = models.CharField(max_length=200)
author = models.ForeignKey(Author, on_delete=models.CASCADE)
price = models.DecimalField(max_digits=5, decimal_places=2)
aggregate()
: The Data Summarizer
Let's say you want to find the average price of all books in your store:
Python
from django.db.models import Avg
average_price = Book.objects.aggregate(Avg('price'))
print(average_price) # Output: {'price__avg': Decimal('25.50')} (example)
aggregate()
returns a dictionary. The key (price__avg
) is automatically generated, but you can customize it:
Python
summary = Book.objects.aggregate(average_book_price=Avg('price'))
print(summary) # Output: {'average_book_price': Decimal('25.50')} (example)
You can also perform multiple aggregations at once:
Python
from django.db.models import Count, Min, Max
summary = Book.objects.aggregate(
book_count=Count('*'),
min_price=Min('price'),
max_price=Max('price')
)
print(summary) # Example Output: {'book_count': 10, 'min_price': Decimal('10.00'), 'max_price': Decimal('50.00')}
annotate()
: The Data Augmentor
Now, let's say you want to know how many books each author has written:
Python
from django.db.models import Count
authors_with_book_count = Author.objects.annotate(book_count=Count('book'))
for author in authors_with_book_count:
print(f"{author.name}: {author.book_count}")
annotate()
returns a QuerySet. Each Author
object in this QuerySet now has a new attribute, book_count
, representing the number of books they've written.
Here's another example where we annotate each book with its author's name:
Python
books_with_author_name = Book.objects.annotate(author_name=models.F('author__name'))
for book in books_with_author_name:
print(f"{book.title} by {book.author_name}")
We use models.F('author__name')
to access related fields in the annotation.
Key Differences Summarized
Feature | aggregate() | annotate() |
Scope | Entire QuerySet | Each object in the QuerySet |
Return Value | Dictionary | QuerySet |
Purpose | Summarize data | Add calculated fields to objects |
When to Use Which
aggregate()
: Use when you need summary statistics (averages, sums, counts) for your data. Think dashboards, reports, and overall metrics.annotate()
: Use when you need to add calculated information to individual objects in your QuerySet. This is useful for displaying related data, creating lists with counts, or preparing data for more complex processing.
Subscribe to my newsletter
Read articles from Rohit Ranjan directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Rohit Ranjan
Rohit Ranjan
I am a software Developer, interested in topics like Python, Django, Backend Development, Data Analysis, Cloud and AI. I learn and write about these topics when i find something interesting