The Ultimate Guide to Getting Location in Android (GPS, Wi-Fi & More!)

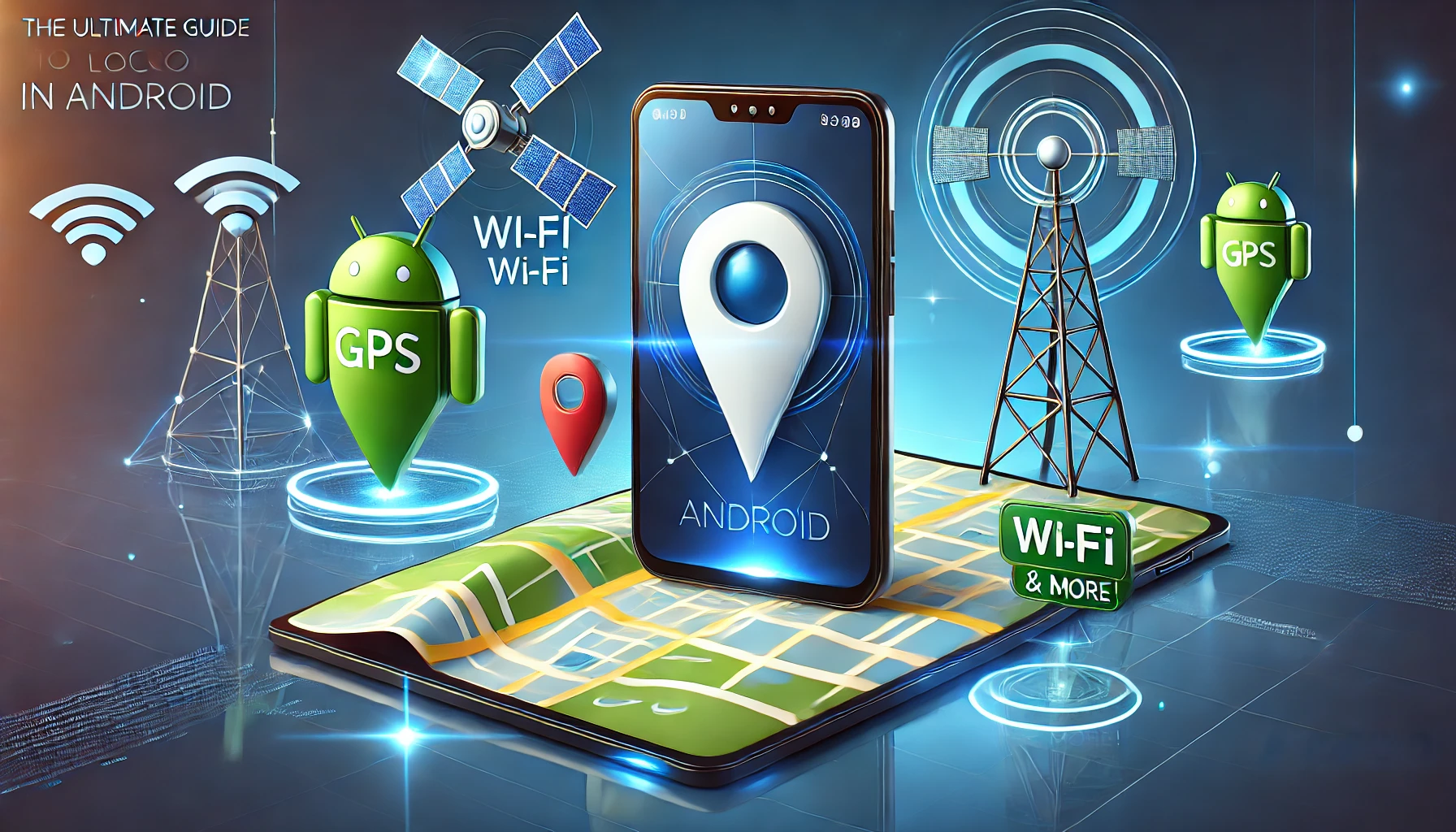
Introduction
Have you ever wondered how your Android phone knows where you are? Whether you're using Google Maps, a ride-hailing app, or a weather app, location services play a huge role in making your experience seamless.
In Android, there are different ways to get location data. You can use the traditional LocationManager (which provides GPS, Network, and Passive location), or you can use the Fused Location Provider (FLP), which is Google's smarter and more efficient solution.
In this blog, we'll break down how location services work, the difference between traditional methods and the Fused Location Provider, and how you can implement them in your Android app.
1. Different Ways to Get Location in Android
Android provides three main location providers:
1.1 GPS_PROVIDER
✅ Uses the device’s GPS hardware to get location.
✅ Provides high accuracy.
❌ Requires a clear view of the sky (doesn't work well indoors).
❌ Drains battery faster.
Code Example:
LocationManager locationManager = (LocationManager) getSystemService(Context.LOCATION_SERVICE);
if (ActivityCompat.checkSelfPermission(this, Manifest.permission.ACCESS_FINE_LOCATION) == PackageManager.PERMISSION_GRANTED) {
locationManager.requestLocationUpdates(LocationManager.GPS_PROVIDER, 5000, 10, locationListener);
}
1.2 NETWORK_PROVIDER
✅ Uses Wi-Fi and mobile networks for location.
✅ Works indoors and is power-efficient.
❌ Less accurate than GPS.
Code Example:
LocationManager locationManager = (LocationManager) getSystemService(Context.LOCATION_SERVICE);
if (ActivityCompat.checkSelfPermission(this, Manifest.permission.ACCESS_FINE_LOCATION) == PackageManager.PERMISSION_GRANTED) {
locationManager.requestLocationUpdates(LocationManager.NETWORK_PROVIDER, 5000, 10, locationListener);
}
1.3 PASSIVE_PROVIDER
✅ Listens for location updates requested by other apps.
✅ Saves battery (since it doesn’t request updates itself).
❌ No control over update frequency.
Code Example:
LocationManager locationManager = (LocationManager) getSystemService(Context.LOCATION_SERVICE);
if (ActivityCompat.checkSelfPermission(this, Manifest.permission.ACCESS_FINE_LOCATION) == PackageManager.PERMISSION_GRANTED) {
locationManager.requestLocationUpdates(LocationManager.PASSIVE_PROVIDER, 0, 0, locationListener);
}
2. The Better Way: Fused Location Provider (FLP)
2.1 What is Fused Location Provider?
Instead of using GPS, Network, or Passive location separately, Google recommends using FusedLocationProviderClient, which is part of Google Play Services. It automatically selects the best location provider based on accuracy, battery usage, and availability.
Fused Location Provider can use:
GPS (when high accuracy is needed)
Wi-Fi & Mobile Networks (for faster but less accurate location)
Device Sensors (to improve accuracy and detect movement)
2.2 How to Use FusedLocationProviderClient in Android
To get the last known location, follow these steps:
Step 1: Initialize FusedLocationProviderClient
FusedLocationProviderClient fusedLocationClient = LocationServices.getFusedLocationProviderClient(this);
Step 2: Get Last Known Location
if (ActivityCompat.checkSelfPermission(this, Manifest.permission.ACCESS_FINE_LOCATION) == PackageManager.PERMISSION_GRANTED) {
fusedLocationClient.getLastLocation()
.addOnSuccessListener(this, new OnSuccessListener<Location>() {
@Override
public void onSuccess(Location location) {
if (location != null) {
double latitude = location.getLatitude();
double longitude = location.getLongitude();
Log.d("FusedLocation", "Latitude: " + latitude + ", Longitude: " + longitude);
}
}
});
}
3. Why Use Fused Location Provider?
Feature | LocationManager | FusedLocationProviderClient |
Accuracy | High (GPS) / Medium (Network) | Automatically optimized |
Battery Consumption | High (GPS) | Low (Smart switching between GPS, Wi-Fi, and sensors) |
Ease of Use | Requires manual setup | Google-managed and optimized |
Indoor Functionality | Works poorly indoors (GPS) | Works well indoors (uses Wi-Fi & sensors) |
Conclusion
If you want high accuracy and better battery performance, the Fused Location Provider (FLP) is the best choice for getting location in Android apps. It automatically picks the best provider (GPS, Wi-Fi, or sensors), ensuring efficient location tracking with minimal battery drain.
Would you like to learn how to get real-time location updates using FLP? Stay tuned for our next blog! 🚀
Subscribe to my newsletter
Read articles from Anmol Singh Tuteja directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
