Array and Objects In JavaScript

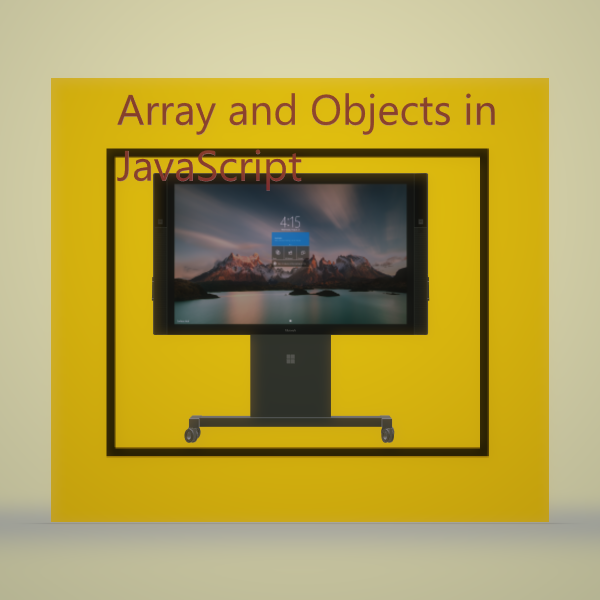
You might be thinking about storing a large chunk of data in one variable. Here I am to fulfill your desired queries. In this article we will learn about the Array and Object and see it’s application with examples.
What is Array ?
Arrays are a non-primitive data type, which help to store multiple values in a single variable. You can say that an array is a special type of object that allows storing a list of items in an array of different data type variables like numbers, strings, or even other arrays and objects.
Creating Array : To create an array ,we use square brackets []
let array = [];
Now let's see an example: Let's assume you want to store a list of fruit names in a single variable. In this situation, we prefer to use an array.
let fruits = ["Apple", "Banana", "Cherry"];
console.log(fruits[0]) // outout : Apple
console.log(fruits[1]) // output : Banana
Accessing Array Elements:
You are wondering about why I am using fruits[0] to access array elements instead of directly using fruits to access all the elements of the array at once like we did earlier.
In an array, each element has zero-based indexing, meaning the first element is at index 0, the second element is at index 1, and so on. why zero based indexing we will look it in next blog.
Modifying Array :
One of the reasons to use an array is that we can modify our array according to our needs. This means the array is mutable.
You can modify an element in an array by using its index.
fruits[1] = "Blueberry"; // Changing "Banana" to "Blueberry"
console.log(fruits); // Output: ["Apple", "Blueberry", "Cherry"]
Array Methods:
JavaScript arrays come with built-in methods that allow you to manipulate the data. Here are some commonly used methods:
.length method: It gives you the size of the array, meaning the total number of elements present in the array.
let fruits = ["Apple", "Blueberry", "Cherry"] console.log(fruits); // Output: ["Apple", "Blueberry", "Cherry"] console.log(fruits.length) // Optput : 3
push() – Adds an element to the end of the array.
pop() – Removes the last element of the array.
shift() – Removes the first element of the array.
unshift() – Adds an element to the beginning of the array.
Similarly, we have a number of built-in methods for arrays. If you want to dive deeper into array methods, you can refer to Array and its method documents.
Objects in JavaScript:
An object in JavaScript is a collection of key-value pairs. A key is also called a property and is always of string type, whereas a value can be of any type like a string, array, number, or even another object.
Creating Object : To create object we use curly braces {}.
Example :
let tea = {
name: "black-tea",
price: 100,
origin: "Mongolia"
};
In the above example:
Tea is a object with its three properties: name, price and origin.
The keys are name, price and city and there values are “black-tea”, 100 and “Mongolia”.
Accessing Object Properties
You can access an object's properties using either dot notation or bracket notation.
Dot Notation
console.log(tea.name); // Output: balck-tea
console.log(tea.price); // Output: 100
Bracket Notation
Bracket notation allows you to access properties using a string or variable.
console.log(tea["name"]); // Output: "black-tea"
Modifying Object Properties
You can modify an object's properties by directly assigning a new value to them.
tea.price = 200; // Changing age from 100 to 200
console.log(tea.price); // Output: 200
Object method to be continued in next blog stay tune
Subscribe to my newsletter
Read articles from ANKESH KUMAR SINGH directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
