Understanding Objects in JavaScript
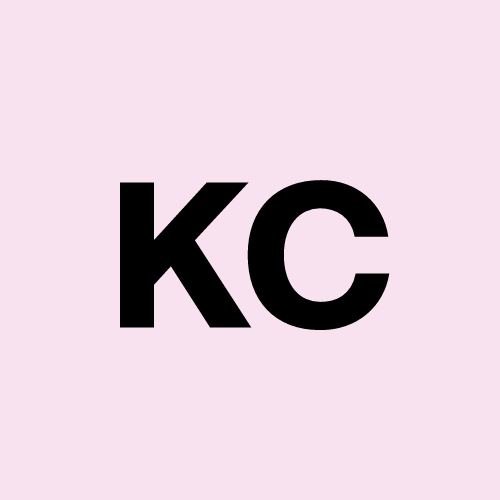
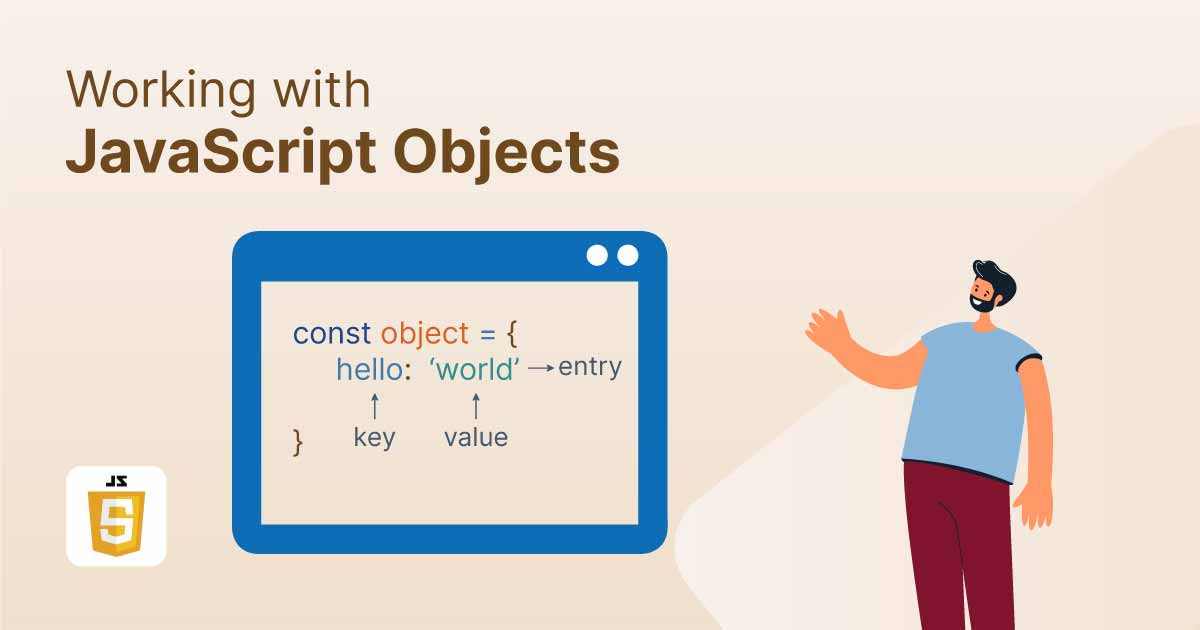
Let's take a step forward in JavaScript by learning about objects. A fun fact about JavaScript is that almost everything in it is an object.
Letβs get started π
Objects
Objects in JavaScript are an important data structure. They consist of key-value pairs. In objects, you can have properties and various methods related to that object. A method is simply a behavior. Objects are a non-primitive data type, so they are dynamic. We can easily add, modify, or delete elements or methods from objects because of their mutable nature. Objects are mainly stored in heap memory, and their reference is stored in a variable in stack memory.
Various ways to create objects
Object literal
{ }
This is one of the simplest ways to create objects. It performs well and is very easy to use because its syntax is straightforward.
let obj={
name:'karan'
}
console.log('Obj1:',obj); //Output: Obj1: { name: 'karan' }
By using
new
keywordOne another way to create objects is using new keyword.
new
keyword is basically used to create an instance.
let obj=new Object()
obj.name="Karan"
console.log(obj) //Output: { name: 'Karan' }
Performing CRUD Operations
Object literal
{ }
This is one of the simplest ways to create objects. It performs well and is very easy to use because its syntax is straightforward.
let obj={
name:'karan'
}
console.log('Obj1:',obj); //Output: Obj1: { name: 'karan' }
Adding properties
We can easily add properties in object by just using dot operator
obj_name.poperty_name=value
let obj={
name:'karan'
}
obj.age=19
console.log(obj) // Output: { name: 'karan', age: 19 }
Modifying properties
Just like adding properties we can modify them also by using the same syntax.
obj_name.poperty_name=value
let obj={
name:'karan'
}
obj.name='Sahil'
console.log(obj) // Output: { name: 'Sahil' }
Delete Properties
The syntax for deleting properties from an object is slightly different.
delete object_name.property_name
let obj={
name:'karan',
age:19
}
console.log(obj) // Output: { name: 'Sahil',age: 19 }
delete obj.age
console.log(obj) // Output: { name: 'Sahil' }
Checking Existence of Property in Object
One can easily check wheter the property is available in object or not. Objects propvide one method that is object.hasOwnProperty(β property_name β)
. It give output in terms of true and false only just like your boolean primitive data type.
let obj={
name:'karan',
age:19
}
console.log(obj) // Output: { name: 'Sahil',age: 19 }
console.log(obj.hasOwnProperty('age')) // Output: true
Memory Allocation
Objects are stored in heap memory, which is known as dynamic memory. There are two types of memory: stack and heap. Primitive data types are stored in the stack, while non-primitive types like objects and arrays are stored in the heap, while their references (memory addresses) are stored in the stack.
Conclusion
JavaScript objects are a fundamental data structure that allows us to store key-value pairs dynamically. They are mutable, making it easy to add, modify, and delete properties. Objects are stored in heap memory, with references in stack memory. Understanding objects is crucial for mastering JavaScript as almost everything in JavaScript revolves around them. π
Subscribe to my newsletter
Read articles from Karanraj Chauhan directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
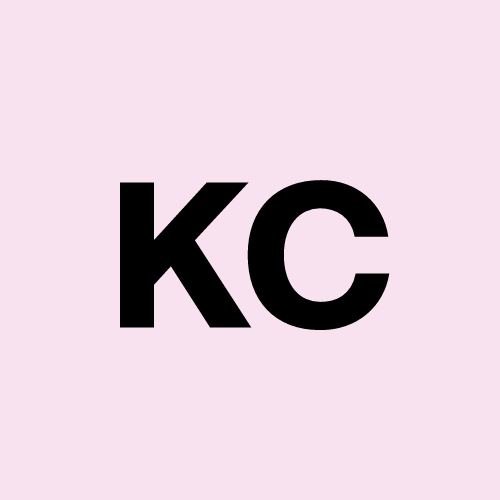