Understanding Dependency Injection in Go: A Practical Guide
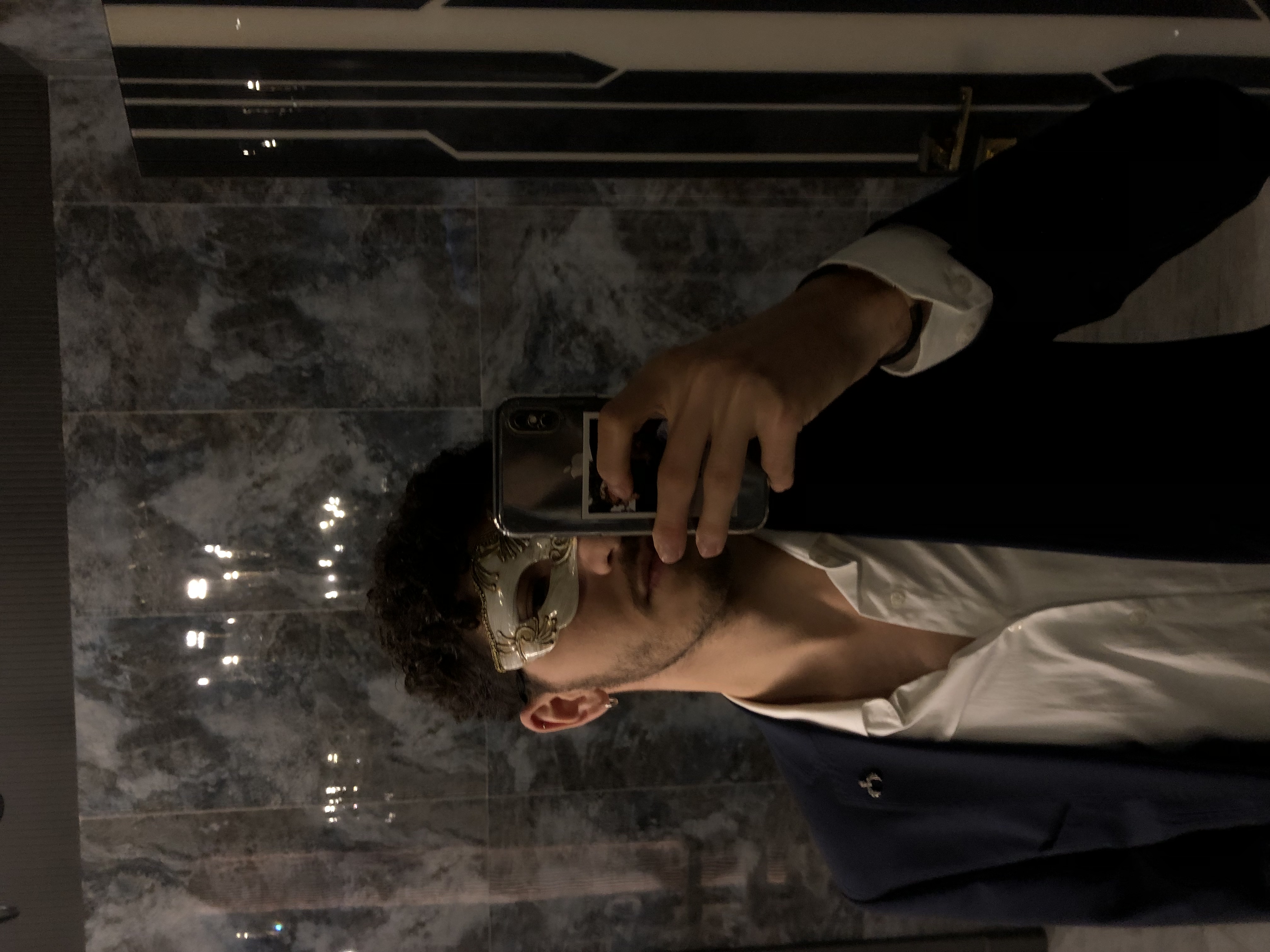
Have you ever had your code become too tightly coupled, making it hard to test and maintain? Some people certainly have. Let me share a story about how dependency injection would help you write better, more maintainable Go code.
The Problem: A Coffee Machine Gone Wrong
Let's start with a real-world analogy. Imagine you're building a coffee machine program. Here's how you might initially write it:
type CoffeeMachine struct {
grinder *Grinder
heater *Heater
}
func NewCoffeeMachine() *CoffeeMachine {
return &CoffeeMachine{
grinder: NewGrinder(),
heater: NewHeater(),
}
}
func (c *CoffeeMachine) BrewCoffee() string {
c.grinder.Grind()
c.heater.Heat()
return "Your coffee is ready!"
}
What's wrong with this code? The CoffeeMachine
is creating its own dependencies (grinder and heater). It's like buying a coffee machine where the grinder and heater are permanently welded inside. If either breaks, you'd have to replace the entire machine!
Enter Dependency Injection
Dependency injection is like making a modular coffee machine where you can easily swap out components. Here's how we can improve our code:
type Grinder interface {
Grind() string
}
type Heater interface {
Heat() string
}
type CoffeeMachine struct {
grinder Grinder
heater Heater
}
func NewCoffeeMachine(g Grinder, h Heater) *CoffeeMachine {
return &CoffeeMachine{
grinder: g,
heater: h,
}
}
Now we're passing the dependencies through the constructor. This is dependency injection in its simplest form!
Why This Matters: Testing Made Easy
Let's say we want to test our coffee machine. With the first approach, we'd need real grinder and heater components. But with dependency injection, we can create mock components:
type MockGrinder struct{}
func (m *MockGrinder) Grind() string { return "Mock grinding" }
type MockHeater struct{}
func (m *MockHeater) Heat() string { return "Mock heating" }
func TestCoffeeMachine(t *testing.T) {
mockGrinder := &MockGrinder{}
mockHeater := &MockHeater{}
machine := NewCoffeeMachine(mockGrinder, mockHeater)
result := machine.BrewCoffee()
if result != "Your coffee is ready!" {
t.Error("Expected coffee to be ready")
}
}
A More Real-World Example: User Service
Let's look at a more practical example - a user service that needs to interact with a database and send emails:
type UserService struct {
db Database
mailer EmailService
logger Logger
}
type Database interface {
SaveUser(user User) error
GetUser(id string) (User, error)
}
type EmailService interface {
SendWelcomeEmail(user User) error
}
type Logger interface {
Log(message string)
}
func NewUserService(db Database, mailer EmailService, logger Logger) *UserService {
return &UserService{
db: db,
mailer: mailer,
logger: logger,
}
}
func (s *UserService) CreateUser(user User) error {
s.logger.Log("Creating new user")
if err := s.db.SaveUser(user); err != nil {
return err
}
if err := s.mailer.SendWelcomeEmail(user); err != nil {
s.logger.Log("Failed to send welcome email")
return err
}
return nil
}
Using the Service
Here's how you might use this service in your application:
func main() {
db := postgres.NewConnection()
mailer := smtp.NewEmailService()
logger := zap.NewLogger()
userService := NewUserService(db, mailer, logger)
user := User{
ID: "1",
Email: "john@example.com",
Name: "John Doe",
}
err := userService.CreateUser(user)
if err != nil {
log.Fatal(err)
}
}
Benefits of This Approach
- Testability: You can easily create mock implementations for testing.
- Flexibility: You can swap implementations without changing the service code.
- Separation of Concerns: Each component has a single responsibility.
- Maintainability: Dependencies are explicit and visible.
Common Patterns and Best Practices
- Use Interfaces: Define interfaces for your dependencies instead of concrete types.
- Constructor Injection: Pass dependencies through constructors rather than setting them after creation.
- Keep Dependencies Minimal: Only inject what you need.
- Use Functional Options: For optional dependencies or configuration.
Here's an example of functional options:
type UserServiceOption func(*UserService)
func WithLogger(logger Logger) UserServiceOption {
return func(s *UserService) {
s.logger = logger
}
}
func NewUserService(db Database, mailer EmailService, opts ...UserServiceOption) *UserService {
s := &UserService{
db: db,
mailer: mailer,
logger: defaultLogger{}, // Default implementation
}
for _, opt := range opts {
opt(s)
}
return s
}
Conclusion
Dependency injection might seem like extra work at first, but it pays off in the long run. It makes your code more testable, maintainable, and flexible. Think of it as building with LEGO blocks instead of carving from a single piece of wood - you can always rearrange the pieces as needed.
Remember:
- Start with interfaces
- Inject dependencies through constructors
- Keep your components loosely coupled
- Think about testing from the start
Subscribe to my newsletter
Read articles from Mirkenan Kazımzade directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
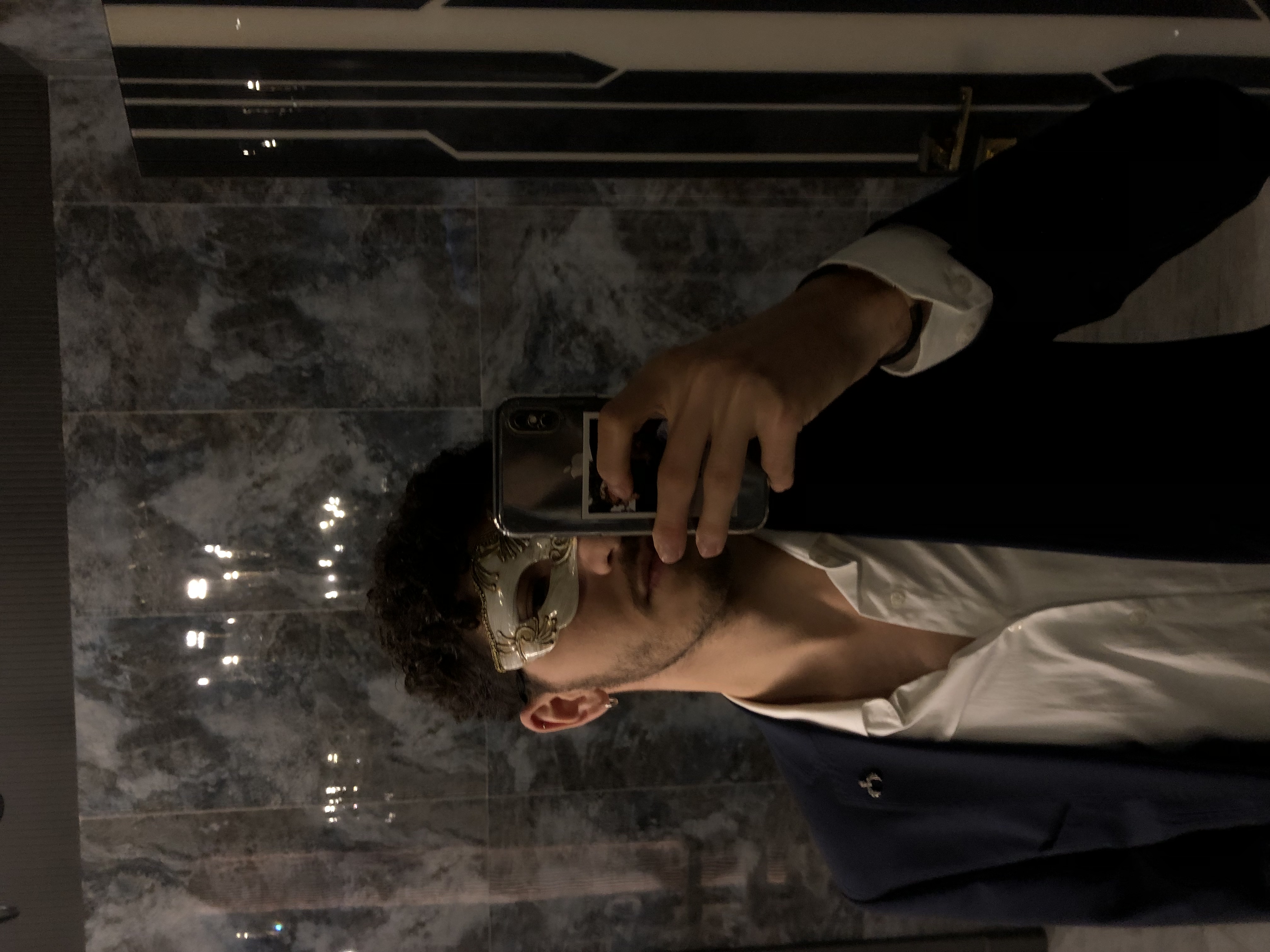