Make Users Smile: Fix Memory Leaks in Your Flutter App
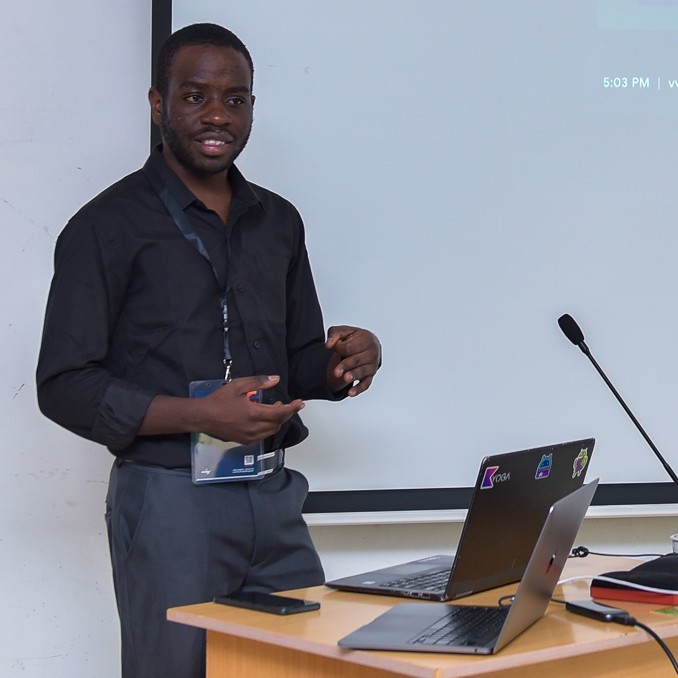

INTRODUCTION
Memory leaks occur when your app retains memory that is no longer needed, leading to performance issues, crashes, and excessive resource usage.
I know that most of us in Flutter might have been thinking that memory leaks only impact app size, but they quietly degrade performance. Beyond consuming storage, memory leaks slow down your app, cause crashes, and harm user experience. By implementing the right strategies, you can keep your Flutter apps fast, responsive, and reliable across all platforms.
Common Causes Memory Leaks in Flutter
Efficient memory management is crucial for maintaining a smooth and responsive Flutter app. By identifying common causes, applying platform-specific optimizations, and following best practices, you can prevent memory leaks and enhance app performance.
Unreleased Streams & Listeners π
Active stream subscriptions and event listeners can persist in memory if not properly disposed of, leading to memory leaks. Always cancel stream subscriptions and remove listeners in the dispose()
method of a StatefulWidget
:
@override
void dispose() {
myStreamSubscription.cancel();
myController.dispose();
super.dispose();
}
Mismanaged Async Operations β‘
Async tasks such as HTTP requests and database queries can consume memory if not handled correctly. Use CancelToken
to abort HTTP requests when no longer needed:
CancelToken cancelToken = CancelToken();
void fetchData() {
Dio().get('https://api.example.com/data', cancelToken: cancelToken);
}
@override
void dispose() {
cancelToken.cancel();
super.dispose();
}
For streams, use takeWhile
to automatically cancel subscriptions when the widget is disposed:
stream.takeWhile((_) => mounted).listen((data) {
// Process data
});
Overuse of
setState()
π
Calling setState()
excessively can cause unnecessary widget rebuilds, leading to performance issues. Optimize state updates by:
Updating only when necessary.
Using
const
widgets to prevent unnecessary re-renders.Leveraging state management solutions like Provider, Riverpod, or BLoC.
Retaining Large Data Objects π
Large assets such as images, files, or cached data can lead to excessive memory usage if not properly managed:
Release large assets when no longer needed.
Optimize images by setting
cacheWidth
andcacheHeight
inImage.asset
orImage.file
to reduce memory consumption:
Image.asset('assets/image.png', cacheWidth: 200, cacheHeight: 200);
Singletons & Global Variables π
Singletons and global variables persist throughout the appβs lifecycle, potentially leading to memory leaks. Properly manage their lifetimes by implementing disposal mechanisms:
class MySingleton {
static final MySingleton _instance = MySingleton._internal();
factory MySingleton() => _instance;
MySingleton._internal();
void dispose() {
// Cleanup resources
}
}
Dependency injection frameworks like get_it
or riverpod
can also help manage object lifetimes more effectively.
Web-Specific Memory Leaks π
Web-based Flutter applications can suffer from memory leaks if DOM elements and event listeners are not properly removed:
Dispose event listeners when widgets are destroyed.
Optimize UI for web: Some mobile-specific animations may cause performance issues in browsers. Use simpler animations or conditionally disable them on web:
if (kIsWeb) {
// Use simpler animations for web
}
- Platform-Specific Optimizations π±
Efficient List Handling
For large datasets, prefer
ListView.builder
overListView
to render only visible items, conserving memory:
ListView.builder(
itemCount: items.length,
itemBuilder: (context, index) {
return ListTile(title: Text(items[index]));
},
);
Reducing Animation Complexity
On lower-end devices or web applications, simplify complex animations to improve performance:
if (!kIsWeb && Platform.isAndroid) {
// Apply full animations
} else {
// Use lightweight animations for web and older devices
}
How to Detect Memory Leaks in Flutter
Identifying memory leaks in your Flutter app can be challenging, but with the right tools and techniques, it's achievable. Hereβs how you can detect and fix memory leaks effectively:
1. Use Flutter DevTools
Flutter DevTools is a powerful debugging and performance monitoring tool for Dart and Flutter applications. It allows you to analyze your appβs memory usage. To access it, run:
flutter pub global run devtools
2. Capture Heap Snapshots
Taking heap snapshots at different points in time helps monitor memory usage and detect leaks. These snapshots can reveal objects that arenβt being garbage collected as expected, indicating a possible memory leak.
3. Review Your Code
Carefully analyze your code to ensure objects are properly disposed of when no longer needed. Pay special attention to controllers, as they are a common cause of memory leaks in Flutter. Always dispose of them correctly to prevent unnecessary memory retention.
By leveraging these techniques, you can keep your Flutter app efficient and free of memory leaks.
Best Practices & Tools
Always override
dispose()
inStatefulWidget
to clean up controllers, streams, and listeners.Use Flutter DevTools to monitor memory and detect leaks early.
Manage object retention: Avoid caching objects indefinitely; use weak references where applicable.
Optimize async execution: Properly handle
async
/await
and ensure tasks are canceled when no longer needed.
Letβs create Flutter apps that are not just beautiful but also high-performing! ππ‘
Subscribe to my newsletter
Read articles from Siro Daves directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
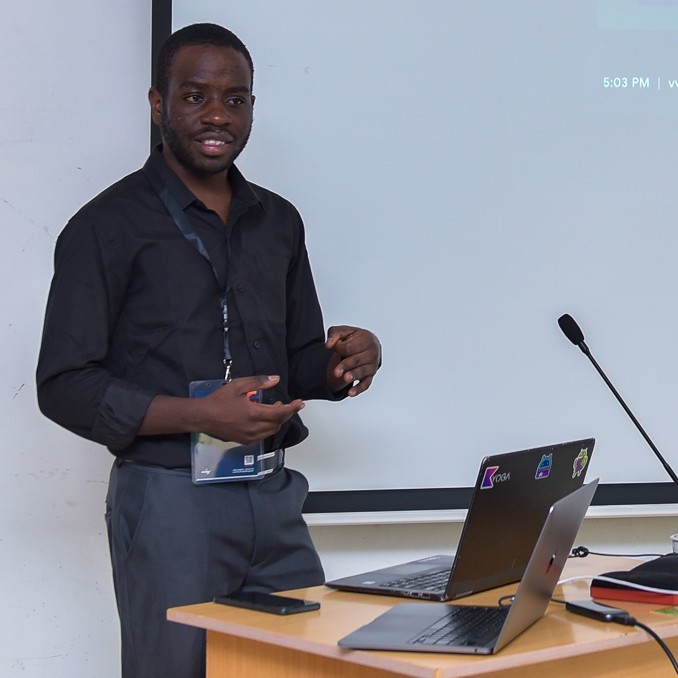
Siro Daves
Siro Daves
Software engineer and a Technical Writer, Best at Flutter mobile app development, full stack development with Mern. Other areas are like Android, Kotlin, .Net and Qt