React Fiber Works with Three.js in Web Development

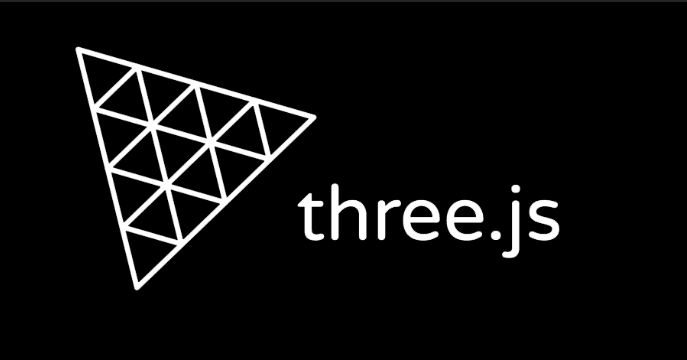
React Three Fiber (R3F) is a powerful React renderer for Three.js, enabling developers to build 3D graphics using React components. It allows for the creation of complex 3D scenes in a declarative and reusable manner, integrating seamlessly with the React ecosystem.
Key Features of React Three Fiber:
Declarative Approach: Build 3D scenes using self-contained, reusable components that respond to state changes, promoting a clean and maintainable code structure.
Full Three.js Support: R3F provides complete access to all Three.js features without limitations, ensuring that any functionality available in Three.js can be utilized within R3F.
Performance Optimization: By rendering components outside of React's reconciliation process, R3F minimizes overhead, often outperforming traditional Three.js implementations, especially in large-scale applications.
Getting Started with React Three Fiber:
To begin using R3F, install the necessary packages:
bashCopyEditnpm install three @react-three/fiber
Here's a basic example of creating a rotating cube:
jsxCopyEditimport React, { useRef } from 'react';
import { Canvas, useFrame } from '@react-three/fiber';
function RotatingBox() {
const meshRef = useRef();
useFrame(() => {
meshRef.current.rotation.x += 0.01;
meshRef.current.rotation.y += 0.01;
});
return (
<mesh ref={meshRef}>
<boxGeometry args={[1, 1, 1]} />
<meshStandardMaterial color="orange" />
</mesh>
);
}
function App() {
return (
<Canvas>
<ambientLight />
<pointLight position={[10, 10, 10]} />
<RotatingBox />
</Canvas>
);
}
export default App;
In this example, the RotatingBox
component defines a cube that rotates continuously. The Canvas
component from R3F serves as the rendering context for the scene.
Ecosystem and Extensions:
R3F boasts a vibrant ecosystem with numerous extensions to enhance development:
@react-three/drei: A collection of useful helpers and abstractions to expedite development.
@react-three/postprocessing: Integrates post-processing effects seamlessly into R3F projects.
@react-three/cannon: Provides physics-based components for realistic simulations.
For comprehensive documentation and advanced tutorials, visit the official React Three Fiber website.
By leveraging React Three Fiber, developers can create interactive and dynamic 3D web applications with the declarative power of React, streamlining the process of building complex visualizations and experiences.
Subscribe to my newsletter
Read articles from kietHT directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

kietHT
kietHT
I am a developer who is highly interested in TypeScript. My tech stack has been full-stack TS such as Angular, React with TypeScript and NodeJS.