#100DaysOfCloudDevOps Challenge — Day 07 — Powershell
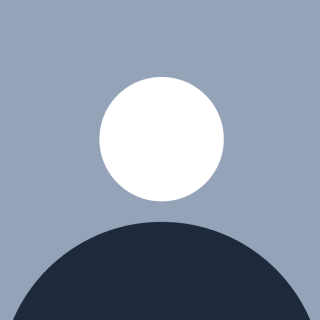
Table of contents

What is Powershell?
PowerShell is a tool that helps automate tasks and manage systems. It includes a command-line interface (where you type commands), a scripting language (to write custom tasks), and a framework to manage configurations. PowerShell works on Windows, Linux, and macOS.
Install PowerShell on Windows, Linux, and macOS: https://learn.microsoft.com/en-us/powershell/scripting/install/installing-powershell?view=powershell-7.5
The three core cmdlets in PowerShell
Get-Help
Get-Command
Get-Member
People often ask me, “How do you determine the commands in PowerShell?” Two essential tools for exploring and understanding PowerShell commands are Get-Help and Get-Command.
Key Aspects of PowerShell
Cmdlets - Basic System Commands
PowerShell cmdlets help manage processes, files, and system information.
📌 Example: Search for a module (e.g., Azure)
# This will search the PowerShell Gallery for any modules that match Az, which is commonly used for managing Azure resources.
Find-Module -Name "Az"
📌 Example: Search for modules related to AWS
# This will return the AWS module to manage AWS resources in PowerShell.
Find-Module -Name "AWSPowerShell"
📌 Example: Check running processes
Get-Process | Select-Object -First 10 # Show first 10 running processes
📌 Example: Find a specific process (e.g., Safari)
Get-Process -Name "Safari"
📌 Example: Kill an unresponsive app (e.g., Safari)
Stop-Process -Name "Safari" -Force
📌 Example: Get the current system date and time
Get-Date
📌 Example: Get the user current timezone
Get-TimeZone
Pipelines - Data Filtering & Sorting
Pipelines allow passing output from one command to another.
📌 Example: Find large files in a folder
Get-ChildItem -Path ~/Documents -Recurse | Where-Object { $_.Length -gt 10MB }
📌 Example: List the top 5 CPU-consuming processes
Get-Process | Sort-Object CPU -Descending | Select-Object -First 5
📌 Example: Find all .log files and delete them
Get-ChildItem -Path ~/Documents -Filter "*.log" | Remove-Item -Force
Objects - Structured Data Handling
PowerShell deals with objects, not just text.
📌 Example: Get system information as an object
$systemInfo = system_profiler SPHardwareDataType
Write-Output $systemInfo
📌 Example: Extract specific details from objects
(system_profiler SPHardwareDataType | ConvertFrom-Csv).ModelName
📌 Example: Display disk space usage
Get-PSDrive -PSProvider FileSystem
Scripting - Automate Repetitive Tasks
📌 Example: Create a script to clean temporary files
- Open Terminal and create a new PowerShell script:
code ~/cleanup.ps1
- Add this code inside the script:
$folder = "~/Downloads"
Get-ChildItem -Path $folder -Recurse | Where-Object { $_.LastWriteTime -lt (Get-Date).AddDays(-30) } | Remove-Item -Force
Write-Output "Old files deleted!"
- Save and run:
pwsh ~/cleanup.ps1
📌 Example: Backup files to an external drive
Copy-Item -Path ~/Documents/* -Destination /Volumes/BackupDrive -Recurse
📌 Example: Script to check disk space and send an alert
$freeSpace = (Get-PSDrive -Name C).Free
if ($freeSpace -lt 10GB) {
Write-Host "Warning: Low disk space!"
} else {
Write-Host "Disk space is sufficient."
}
Remoting - Connect to Remote Systems
PowerShell can manage remote machines.
📌 Example: SSH into a Linux Server
Enter-PSSession -HostName "user@yourserver.com" -UseSSH
📌 Example: Run a command on a remote machine
Invoke-Command -HostName "user@yourserver.com" -UseSSH -ScriptBlock { Get-Date }
Modules - Extend PowerShell Features
Modules add new commands.
📌 Example: Install and use a module
Install-Module -Name PowerShellGet -Scope CurrentUser -Force
Import-Module PowerShellGet
📌 Example: Get a list of installed modules
Get-Module -ListAvailable
Automation - Schedule and Automate Tasks
PowerShell automates system administration, cloud, and DevOps tasks.
📌 Example: Automatically restart a service if it’s stopped
$service = Get-Service -Name "Spooler"
if ($service.Status -ne 'Running') {
Start-Service -Name "Spooler"
Write-Host "Service restarted."
}
📌 Example: Backup files every day using Task Scheduler
$Action = New-ScheduledTaskAction -Execute "PowerShell.exe" -Argument "-File C:\Scripts\Backup.ps1"
$Trigger = New-ScheduledTaskTrigger -Daily -At 3am
Register-ScheduledTask -TaskName "DailyBackup" -Action $Action -Trigger $Trigger
Some PowerShell Commands
The basics
✅ Goal: Get comfortable with basic cmdlets.
1. Open PowerShell and run the following commands:
Get-Process # List running processes
Get-Service # List system services
Get-Date # Display current date and time
Get-Command # List available commands
Get-Help Get-Process # Get help on a command
2. Challenge: Use PowerShell to find your system’s hostname and IP address.
hostname
Get-NetIPAddress
Work with Files & Folders
✅ Goal: Learn how to create, modify, and delete files.
1. Create a new folder and a text file:
New-Item -ItemType Directory -Path "C:\PowerShellPractice"
New-Item -ItemType File -Path "C:\PowerShellPractice\log.txt"
2. Write text into the file:
Set-Content -Path "C:\PowerShellPractice\log.txt" -Value "This is a test log."
3. Append more text:
Add-Content -Path "C:\PowerShellPractice\log.txt" -Value "Appending new log entry."
4. Read the file:
Get-Content -Path "C:\PowerShellPractice\log.txt"
5. Challenge: List all .txt files in C:\PowerShellPractice and delete them.
Get-ChildItem -Path "C:\PowerShellPractice" -Filter "*.txt" | Remove-Item
Automate User & Process Management
✅ Goal: Manage processes and system users.
1. Find a running process (e.g., Notepad) and stop it:
Get-Process -Name notepad
Stop-Process -Name notepad -Force
2. List all currently logged-in users:
Get-WmiObject -Class Win32_ComputerSystem | Select-Object UserName
3. Challenge: Check if “notepad.exe” is running every 10 seconds, and stop it if found.
while ($true) {
if (Get-Process -Name notepad -ErrorAction SilentlyContinue) {
Stop-Process -Name notepad -Force
Write-Output "Notepad was running and has been stopped."
}
Start-Sleep -Seconds 10
}
Automate System Tasks with Schedules
✅ Goal: Schedule a script to run automatically.
1. Create a script that logs the system uptime every hour.
(Get-Date).ToString() + " Uptime: " + (Get-Uptime) | Out-File -Append "C:\PowerShellPractice\uptime.log"
2. Schedule the script to run every hour using Task Scheduler:
$Action = New-ScheduledTaskAction -Execute "PowerShell.exe" -Argument "-File C:\PowerShellPractice\uptime.ps1"
$Trigger = New-ScheduledTaskTrigger -Hourly -At (Get-Date).AddHours(1)
Register-ScheduledTask -TaskName "Log System Uptime" -Action $Action -Trigger $Trigger
Manage Cloud Resources (Azure)
✅ Goal: Use PowerShell to manage cloud resources.
📌 Prerequisite: Install Az PowerShell module.
Install-Module -Name Az -Scope CurrentUser -AllowClobber -Force
1. Login to Azure:
Connect-AzAccount
2. Create a new Azure resource group:
New-AzResourceGroup -Name "MyResourceGroup" -Location "EastUS"
3. Challenge: List all VMs in your Azure subscription.
Get-AzVM
Write a Simple PowerShell Script
✅ Goal: Automate a repetitive task.
1. Open Notepad and save the following script as C:\PowerShellPractice\backup.ps1:
$source = "C:\Users\Public\Documents"
$destination = "D:\Backup"
if (-Not (Test-Path $destination)) {
New-Item -ItemType Directory -Path $destination
}
Copy-Item -Path "$source\*" -Destination $destination -Recurse
Write-Output "Backup completed at $(Get-Date)" | Out-File -Append "$destination\backup.log"
2. Run the script in PowerShell:
PowerShell -File "C:\PowerShellPractice\backup.ps1"
3. Challenge: Modify the script to zip the backup folder after copying.
✅ Goal: For macOS and Linux, create a user and set permissions using shell commands in PowerShell.
# Define user details
$userName = "newuser"
$password = "P@ssw0rd"
$group = "staff" # For macOS/Linux, you can use groups like 'staff'
$folderPath = "/Users/Public/Documents"
$permission = "rw" # read/write permissions
# Create new user (Linux/macOS)
sudo dscl . -create /Users/$userName
sudo dscl . -create /Users/$userName UserShell /bin/bash
sudo dscl . -create /Users/$userName RealName "New User"
sudo dscl . -create /Users/$userName Password $password
sudo dscl . -append /Groups/$group GroupMembership $userName
# Set folder permissions
sudo chmod -R u+rw $folderPath # Give user read/write permission
sudo chown -R $userName:$group $folderPath # Change folder ownership
# Output success message
Write-Host "User $userName created and permissions set!"
DevOps - Run PowerShell in CI/CD Pipeline
✅ Goal: Run PowerShell in a DevOps pipeline.
1. In Azure DevOps, GitHub Actions, or GitLab CI/CD, add a PowerShell script step.
Example (Azure DevOps YAML):
steps:
- task: PowerShell@2
inputs:
targetType: 'inline'
script: |
Write-Host "Starting deployment..."
Get-Date
3. Challenge: Modify the pipeline to deploy an Azure VM using PowerShell.
Whether you’re a beginner or an expert, PowerShell provides tools to make system administration and cloud management a breeze. Start with simple commands like Get-TimeZone or create automated tasks such as backing up files daily.
Ready to dive in? Explore the full capabilities of PowerShell with a simple installation on your machine, and try out the practical examples provided. Let PowerShell simplify and automate your workflow today!
Start your PowerShell journey here.
Find the GitHub repo here.
If you find this blog helpful too, give back and show your support by clicking heart or like, share this article as a conversation starter and join my newsletter so that we can continue learning together and you won’t miss any future posts.
Thanks for reading until the end! If you have any questions or feedback, feel free to leave a comment.
Subscribe to my newsletter
Read articles from anj directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by