# Introduction to Full Stack Development: A Beginner's Guide

Table of contents
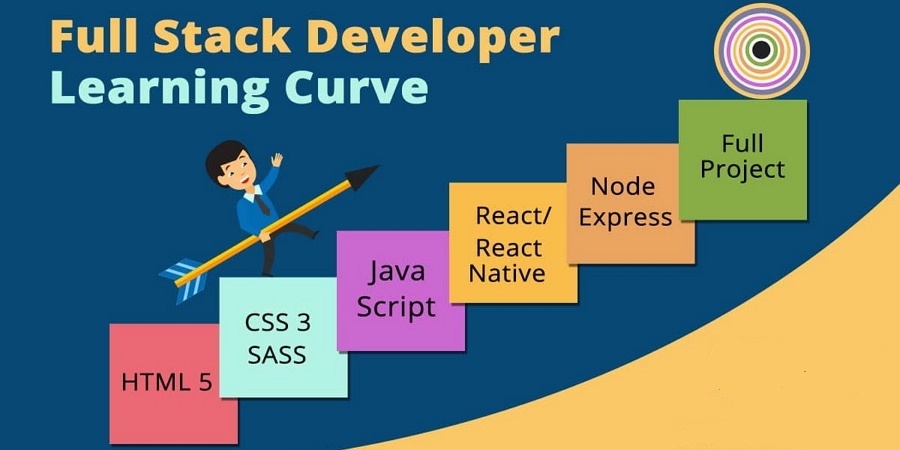
Introduction to Full Stack Development: A Beginner's Guide
In today’s digital age, web development is one of the most sought-after skills. Full Stack Development, particularly the MERN Stack (MongoDB, Express.js, React.js, and Node.js), plays a crucial role in building dynamic web applications. This article will cover the fundamentals of MERN Stack, guide you on building a responsive website using HTML, CSS, and JavaScript, explain RESTful APIs, and share best practices for writing clean, maintainable code.
1. Introduction to MERN Stack: A Beginner’s Guide
The MERN Stack is a powerful JavaScript-based technology stack used to build modern web applications. Let’s break it down:
MongoDB – A NoSQL database that stores data in JSON-like documents.
Express.js – A lightweight backend framework for Node.js to handle server-side logic.
React.js – A JavaScript library for building interactive user interfaces.
Node.js – A runtime environment that allows JavaScript to run on the server.
Why Choose MERN Stack?
✔ JavaScript throughout the stack (Frontend & Backend).
✔ Open-source and actively maintained by the developer community.
✔ Efficient for building Single Page Applications (SPA).
2. How to Build a Responsive Website Using HTML, CSS, and JavaScript
A responsive website ensures a seamless user experience across different devices. Here’s how you can build one:
Step 1: Structure with HTML
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Responsive Website</title>
</head>
<body>
<header>
<h1>Welcome to My Website</h1>
</header>
<main>
<p>This is a responsive webpage.</p>
</main>
</body>
</html>
Step 2: Style with CSS
body {
font-family: Arial, sans-serif;
margin: 0;
padding: 0;
text-align: center;
}
@media (max-width: 600px) {
body {
background-color: lightgray;
}
}
Step 3: Add Interactivity with JavaScript
document.addEventListener("DOMContentLoaded", function() {
alert("Welcome to my responsive website!");
});
By combining these elements, you can create a responsive and interactive website.
3. Understanding RESTful APIs and How They Work
A RESTful API (Representational State Transfer API) is a web service that allows communication between client and server applications.
Key Features of REST APIs:
Uses standard HTTP methods (GET, POST, PUT, DELETE).
Follows a stateless architecture.
Uses JSON for data exchange.
Example: Creating a Simple REST API with Node.js and Express.js
const express = require('express');
const app = express();
app.use(express.json());
app.get('/api/message', (req, res) => {
res.json({ message: "Hello, World!" });
});
app.listen(3000, () => console.log('Server running on port 3000'));
This simple API responds with a JSON message when accessed via a GET request.
4. Best Practices for Writing Clean and Maintainable Code
To ensure your code is efficient, readable, and easy to maintain, follow these best practices:
1. Follow Proper Naming Conventions
Use meaningful variable and function names:
// Bad Naming
let x = 10;
function fn() {
return x * 2;
}
// Good Naming
let price = 10;
function calculateTotalPrice(price) {
return price * 2;
}
2. Keep Functions Small and Focused
A function should perform a single task:
// Avoid long functions
function processUserData(user) {
validateUser(user);
saveUser(user);
sendWelcomeEmail(user);
}
// Better Approach
function validateUser(user) { /* validation logic */ }
function saveUser(user) { /* save logic */ }
function sendWelcomeEmail(user) { /* email logic */ }
3. Use Comments Wisely
Write meaningful comments to explain why, not what:
// Bad Comment
let a = 10; // Declaring a variable
// Good Comment
let maxUsers = 10; // Maximum number of allowed users in the session
4. Maintain Code Formatting and Indentation
Use consistent indentation and spacing:
if (user.isLoggedIn) {
console.log("Welcome, " + user.name);
}
By following these principles, you can ensure your code remains clean and maintainable over time.
Conclusion
Full Stack Development with MERN Stack offers endless possibilities for building scalable web applications. By understanding the MERN Stack, creating responsive websites, utilizing RESTful APIs, and following clean coding practices, you can become a proficient web developer. Start coding today and explore the world of Full Stack Development!
Do you have any questions or feedback? Let’s discuss in the comments!
Subscribe to my newsletter
Read articles from geetha sree directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
