Java Generics 101: Your Complete Resource
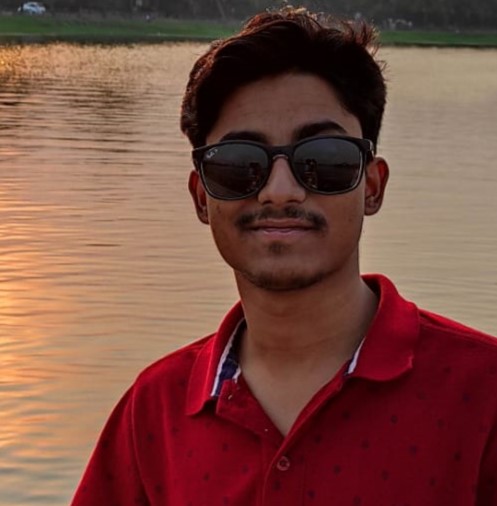
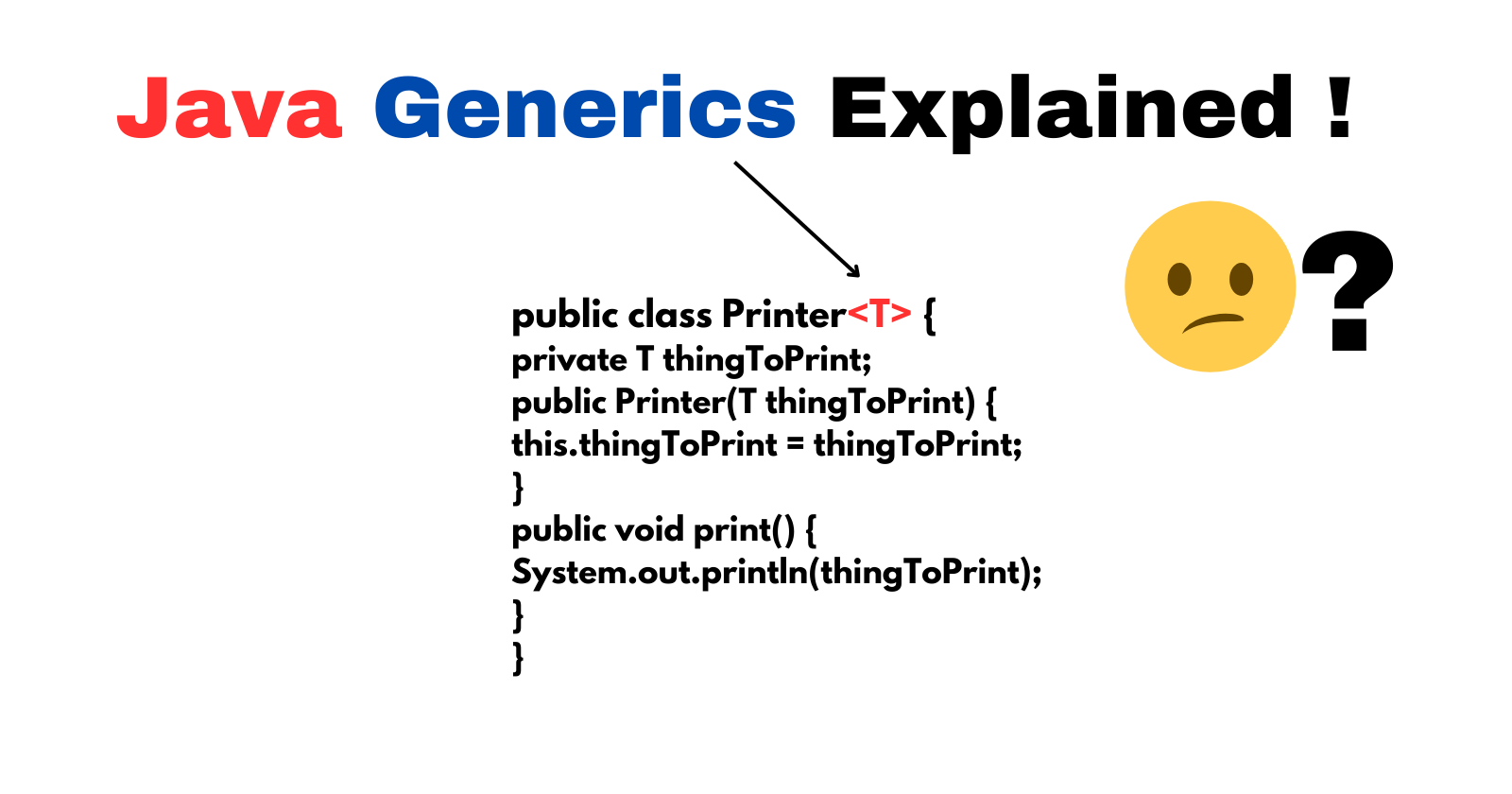
Java Generics might look confusing at first with their angle brackets (<>
), T
, ?
, K
, V
, but once you get the hang of them, they become a key tool for writing flexible, reusable, and type-safe code. In this guide, you'll learn what Generics are and why they are used, how Generics help reduce code duplication, how to create Generic Classes, Methods, and Interfaces, and what Wildcard (?
) and Bounded Generics are and when to use them. By the end of this article, you'll feel confident using Generics in your own Java programs!๐
1๏ธโฃ Why Do We Need Generics? (The Problem Before Generics)
Before Generics, Java developers often faced issues like code duplication and type safety problems.
๐ Example: Without Generics
Let's say we want a class that stores an integer value and prints it:
public class IntegerPrinter {
private Integer thingToPrint;
public IntegerPrinter(Integer thingToPrint) {
this.thingToPrint = thingToPrint;
}
public void print() {
System.out.println(thingToPrint);
}
}
โ Usage:
IntegerPrinter printer = new IntegerPrinter(23);
printer.print(); // Output: 23
But what if we want to print a Double
instead of an Integer
?
We can't use this class because it only works with Integer
.
So, we create another class:
public class DoublePrinter {
private Double thingToPrint;
public DoublePrinter(Double thingToPrint) {
this.thingToPrint = thingToPrint;
}
public void print() {
System.out.println(thingToPrint);
}
}
Now, we need another class for String
:
public class StringPrinter {
private String thingToPrint;
public StringPrinter(String thingToPrint) {
this.thingToPrint = thingToPrint;
}
public void print() {
System.out.println(thingToPrint);
}
}
๐ฅ Problem:
We are repeating code for every different type (Integer
, Double
, String
, etc.).
๐ Solution? Generics!
2๏ธโฃ Introduction to Java Generics
Instead of writing separate classes for each type (IntegerPrinter
, DoublePrinter
, StringPrinter
), we create one generic class that works for all types.
๐ Example: Generic Printer Class
public class Printer<T> { // <T> defines a generic type
private T thingToPrint;
public Printer(T thingToPrint) {
this.thingToPrint = thingToPrint;
}
public void print() {
System.out.println(thingToPrint);
}
}
โ Usage of Generic Class
Printer<Integer> intPrinter = new Printer<>(23);
intPrinter.print(); // Output: 23
Printer<Double> doublePrinter = new Printer<>(33.5);
doublePrinter.print(); // Output: 33.5
Printer<String> stringPrinter = new Printer<>("Hello Generics");
stringPrinter.print(); // Output: Hello Generics
๐น How Generics Solve the Problem?
โ๏ธ One class for multiple data types.
โ๏ธ No need to create separate classes for each type.
โ๏ธ Type Safety: Ensures we donโt pass incorrect types.
3๏ธโฃ Important Generic Concepts
๐น 1. Type Parameters (T
, E
, K
, V
)
T
(Type), E
(Element), K
(Key), V
(Value) are just conventions. You can name them anything, but these are widely used:
T
โ Type parameter (used in generic classes/methods).E
โ Element (commonly used in collections).K, V
โ Key, Value (commonly used in maps).
๐น 2. Generic Methods
Just like generic classes, we can create generic methods inside regular or generic classes.
โ Example: A Generic Method to Print Any Type
public class GenericMethodExample {
public static <T> void shout(T thingToShout) {
System.out.println(thingToShout + "!!!");
}
public static void main(String[] args) {
shout("Hello"); // Output: Hello!!!
shout(42); // Output: 42!!!
shout(3.14); // Output: 3.14!!!
}
}
๐น The <T>
before void
tells Java that T
is a generic type.
๐น 3. Generics in Java Collections
One place you've already used Generics is in Java Collections!
โ
Example: Using Generics in ArrayList
ArrayList<String> names = new ArrayList<>();
names.add("Alice");
names.add("Bob");
// names.add(100); โ ERROR: Type safety ensures only Strings are allowed
๐น Without Generics, Java Collections could store any object, leading to type safety issues.
๐น With Generics, Java prevents type mismatches at compile time instead of runtime.
4๏ธโฃ Advanced Generics: Bounded Types and Wildcards
๐น 1. Bounded Type Parameters (extends
)
We can restrict a generic type to a certain superclass or interface using extends
.
โ Example: Restricting Generics to Animal Subclasses
class Animal {
void eat() {
System.out.println("Animal is eating");
}
}
class Dog extends Animal { }
class Cat extends Animal { }
class Printer<T extends Animal> { // T must be an Animal
private T thingToPrint;
public Printer(T thingToPrint) {
this.thingToPrint = thingToPrint;
}
public void print() {
thingToPrint.eat(); // Safe because T is guaranteed to be Animal
}
}
public class Main {
public static void main(String[] args) {
Printer<Dog> dogPrinter = new Printer<>(new Dog());
dogPrinter.print(); // Output: Animal is eating
// Printer<String> stringPrinter = new Printer<>("Hello"); โ ERROR
}
}
๐น T extends Animal
ensures only Animal
subclasses can be used.
๐น 2. Wildcards (?
)
What if we want a method that can accept a list of any type?
This is where wildcards (?
) help!
โ
Example: Using ?
for Wildcard Generics
public static void printList(List<?> myList) {
System.out.println(myList);
}
public static void main(String[] args) {
List<Integer> numbers = List.of(1, 2, 3);
List<String> words = List.of("Hello", "World");
printList(numbers); // Works โ
printList(words); // Works โ
}
๐น List<?>
means a list of any type.
โ
Bounded Wildcards (? extends Type
)
public static void printAnimals(List<? extends Animal> animals) {
for (Animal a : animals) {
a.eat();
}
}
๐น ? extends Animal
means list elements must be subclasses of Animal
.
5๏ธโฃ Conclusion
Java Generics provide a powerful way to write reusable and type-safe code.
โ
Key Takeaways:
โ๏ธ Generics prevent code duplication by allowing a single class to handle multiple types.
โ๏ธ Type safety ensures compile-time type checking, reducing runtime errors.
โ๏ธ Bounded Generics (T extends Animal
) allow restrictions on generic types.
โ๏ธ Wildcard Generics (?
) allow flexible method parameters.
Now that you understand Generics, go ahead and use them in your Java projects! ๐
๐ก Whatโs next?
๐ Learn about Generic Interfaces and Type Erasure in Java!
Did you find this guide helpful? Let me know in the comments! ๐
Subscribe to my newsletter
Read articles from Arkadipta Kundu directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
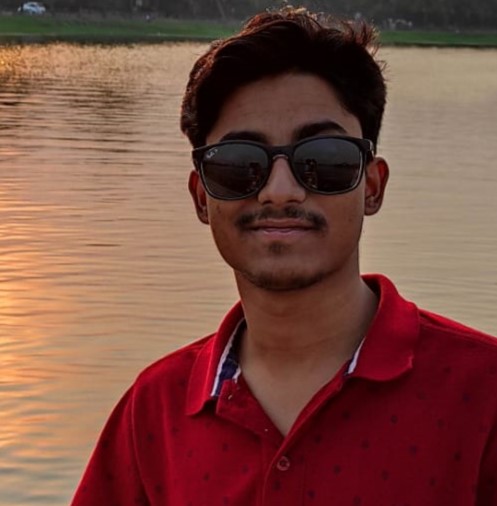
Arkadipta Kundu
Arkadipta Kundu
Iโm a Computer Science undergrad from India with a passion for coding and building things that make an impact. Skilled in Java, Data Structures and Algorithms (DSA), and web development, I love diving into problem-solving challenges and constantly learning. Right now, Iโm focused on sharpening my DSA skills and expanding my expertise in Java development.