Understanding how a higher order operator works in rxjs.
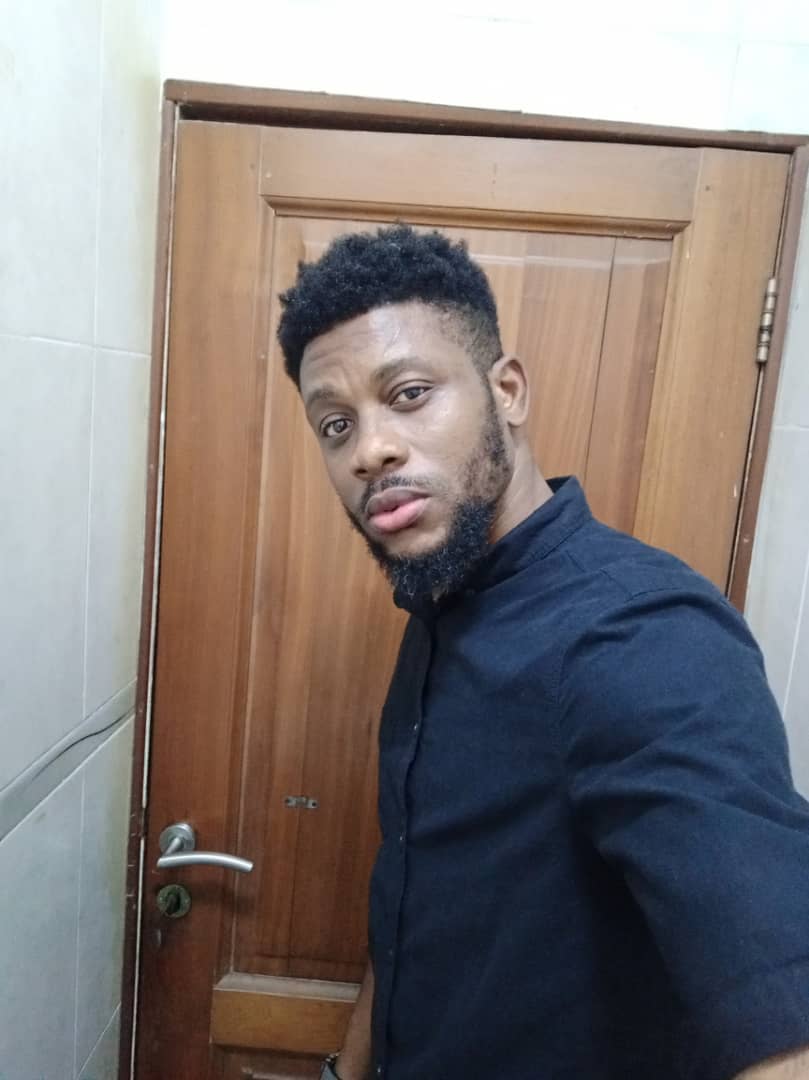
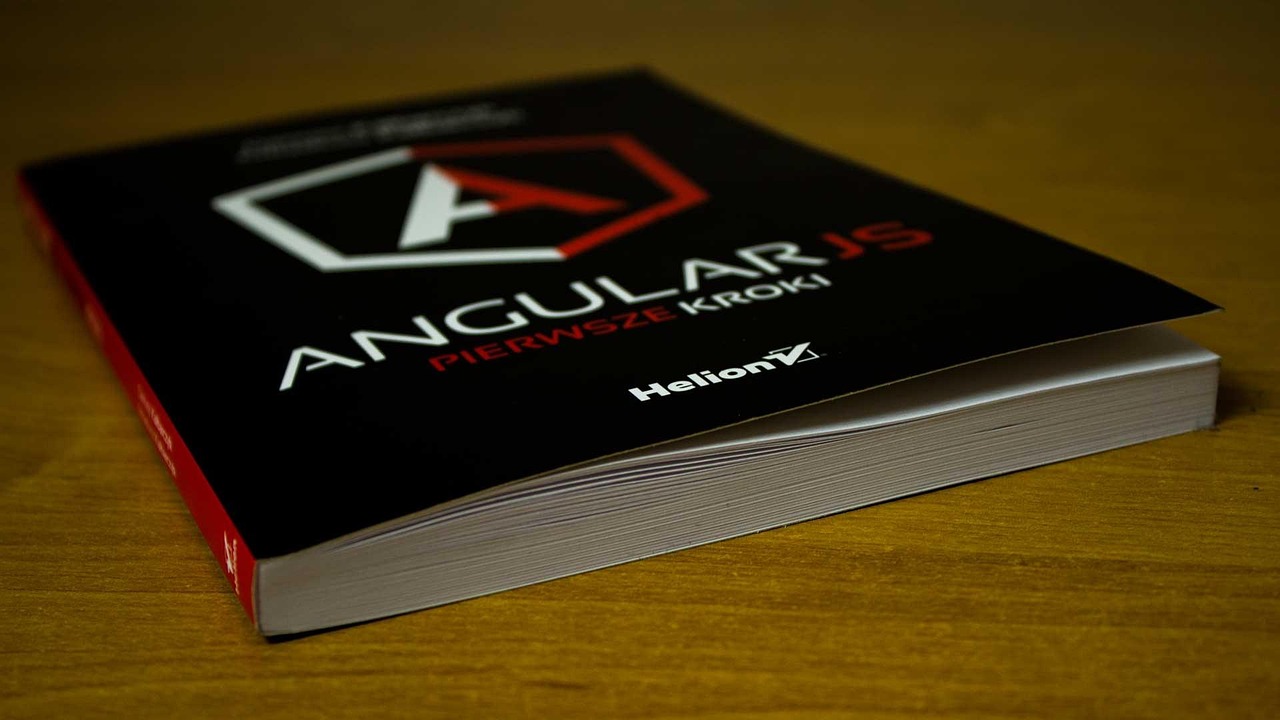
There are a handful of operators in rxjs, and you’d have most likely come across them if you are an Angular Developer.
These operators are distinguished in their functions, and their ability to perform a single task. Everything in rxjs is an observable while some are cold observables others are hot observables.
Prerequisite to follow this article
Javascript
RxJS
Angular (Optional)
Here are a few examples of the rxjs operators by their category:
Creation aka source (i.e of, from etc)
Transformation (i.e map, toArray etc)
Multicasting (i.e share, shareReplay)
Filtering (i.e distinctUntilChange, takeUntil etc)
Conditional
Error handling (i.e catchError, retry etc)
Utility (i.e delay, toPromise etc)
As I mentioned above, there’s a ton of these useful operators, but I only like to talk about the higher order operators.
Higher Order Operators
Higher order operators are simply a type operator that can automatically handle rxjs operators and resolves its value within an observable pipe.
To fully understand how this works, let’s see the problem case and show you how rxjs higher order operators can help us.
const source = from([of(1), of(2), of(3)]);
const obStream = source.pipe().subscribe((value) => {
console.log(value);
});
What you’d get within the console output will be an observable object from each number returned from the source.
With mergeMap
The mergeMap is unique from other higher order operators in its ability to subscribe to the next available emission, which evidently means it doesn’t care about the order of the data in the source observable, whichever one completes first would be returned first.
const source = from([of(1), of(2), of(3)]);
const obStream = source.pipe(
mergeMap(ob => ob)
).subscribe((value) => {
console.log(value);
});
And that’s all you need to do to resolve inner observables with rxjs.
Heads up, we have used mergeMap in this example, but you can decide to use any of the other higher order operators like concatMap(to preserve order), switchMap(to override ongoing subscription on every emission), exhaustMap(to ensure the current subscription complete before subscription to the next emission).
Subscribe to my newsletter
Read articles from Samuel Okeke directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
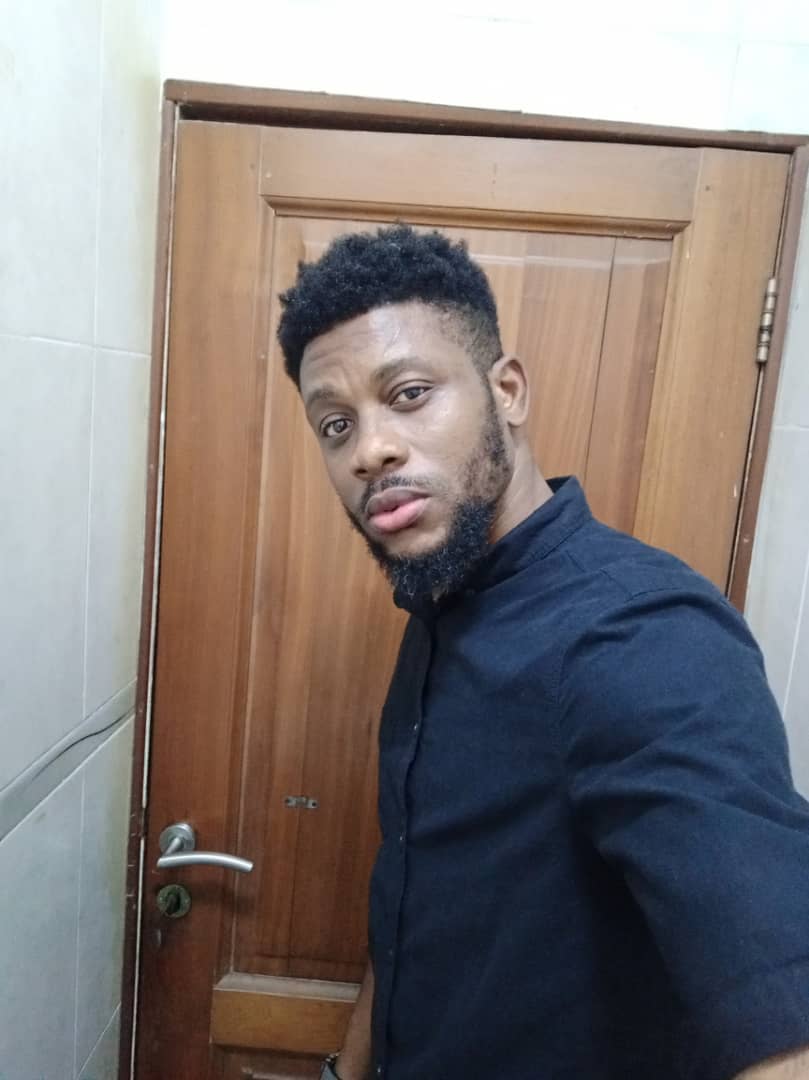
Samuel Okeke
Samuel Okeke
I'm a devout Software Developer with a pinache.