Convert Excel to High-Resolution Images in Python [Pro Guide]

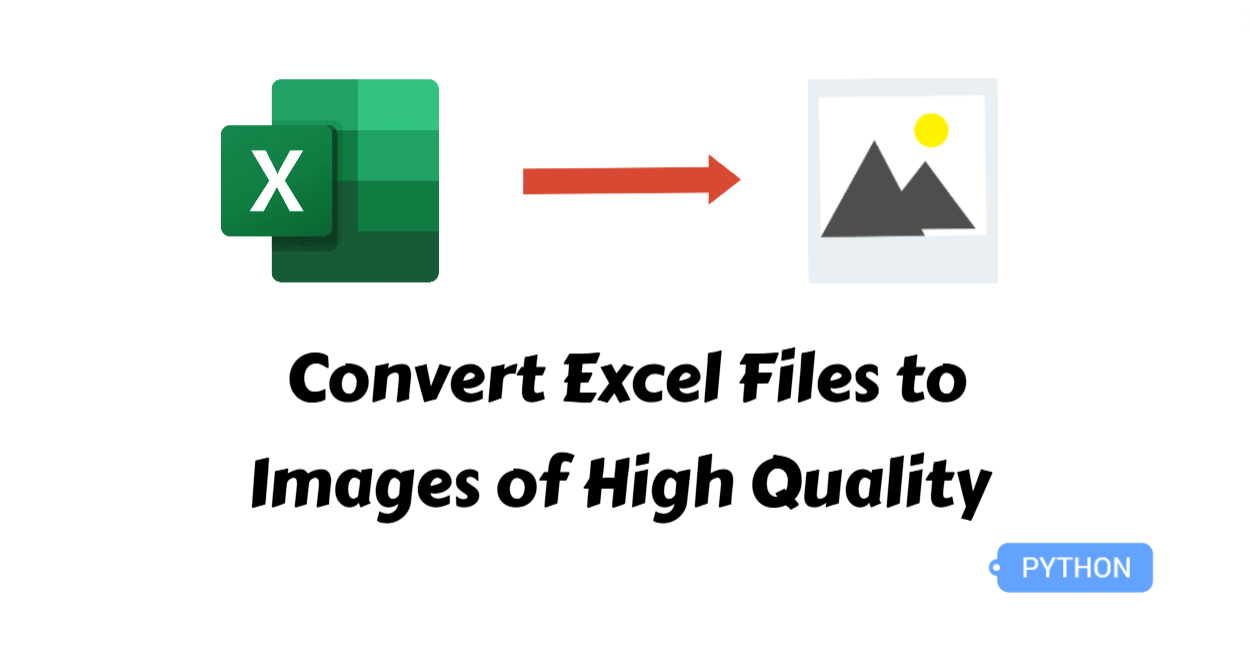
When presenting data in a report or PowerPoint presentation, taking a direct screenshot of an Excel document can result in blurry images, while hyperlinks or embedded files may not be intuitive enough. This makes converting Excel data into high-quality images a crucial task. With Python, you can automate this process by exporting an entire worksheet or a specific cell range as a high-resolution PNG. In this article, we’ll walk you through how to convert Excel to images using Python, providing step-by-step instructions and code examples to help you master this technique efficiently.
Python Library to Convert Excel to Images
In this guide, third-party Excel libraries are recommended to make the task easier. You might first consider open-source libraries like pandas or openpyxl, but they are not well-suited for this task. Pandas may struggle with maintaining Excel's formatting, while openpyxl only supports chart exports, not full-cell content.
That’s why this article recommends using Spire.XLS for Python. As a standalone library, it doesn’t rely on Microsoft Excel or external tools, ensuring accurate and complete Excel-to-image conversion. Additionally, it supports various other tasks, such as converting Excel to HTML or PDF, as well as encrypting and decrypting Excel files. You can install it using the pip command: pip install Spire.XLS
.
Convert Excel Worksheets to Images of High Quality
The most common requirement is to convert an entire Excel worksheet into an image. Visualizing data in image format makes it more intuitive, enhances the clarity of reports, and strengthens the credibility of analysis and conclusions. Spire.XLS for Python offers the Worksheet.SaveToImage() method, which allows you to quickly convert any Excel worksheet into a high-quality image. Let's explore the specific steps to use this method.
Steps to convert Excel worksheet to images:
Create an object of the Workbook class.
Read an Excel spreadsheet from files using the Workbook.LoadFromFile() method.
Get a worksheet through the Workbook.Worksheets[] property.
Convert the Excel worksheet to images with the Worksheet.ToImage() method.
Save the image as a PNG file or other common image formats.
Here’s the Python example of converting the first worksheet to an image:
from spire.xls import *
from spire.xls.common import *
# Create a Workbook object
workbook = Workbook()
# Load an Excel file
workbook.LoadFromFile("/Population.xlsx")
# Get the first worksheet
sheet = workbook.Worksheets[0]
# Save the worksheet to an image
image = sheet.ToImage(sheet.FirstRow, sheet.FirstColumn, sheet.LastRow, sheet.LastColumn)
# Save the image to a PNG file
image.Save("/SheetToImage.png")
workbook.Dispose()
Convert Excel Worksheets to Images without White Margins
Sometimes, when converting an Excel worksheet to an image, you may notice unwanted white margins around the cells. To eliminate these margins and obtain a clean, well-cropped image, you can set the margins to zero before conversion. Let’s go through the specific steps to achieve this.
Steps to convert Excel worksheets to images without white margins:
Create an instance of the Workbook class.
Load an Excel file through the Workbook.LoadFromFile() method.
Get a specific worksheet through the Workbook.Worksheets[] property.
Remove all the margins of the worksheet by setting them as zero.
Convert the Excel worksheet to images using the Worksheet.ToImage() method.
Save the image as a PNG file.
Below is the code example of exporting the first worksheet of an Excel file without white margins:
from spire.xls import *
from spire.xls.common import *
# Create a Workbook object
workbook = Workbook()
# Load an Excel file
workbook.LoadFromFile("/Population.xlsx")
# Get the first worksheet
sheet = workbook.Worksheets[0]
# Set all margins of the worksheet to zero
sheet.PageSetup.LeftMargin = 0
sheet.PageSetup.BottomMargin = 0
sheet.PageSetup.TopMargin = 0
sheet.PageSetup.RightMargin = 0
# Convert the worksheet to an image
image = sheet.ToImage(sheet.FirstRow, sheet.FirstColumn, sheet.LastRow, sheet.LastColumn)
# Save the image to a PNG file
image.Save("/SheetToImageWithoutMargins.png")
workbook.Dispose()
Export Excel Cell Ranges as Images in Python
In some cases, you may only need to display a specific portion of the data instead of the entire Excel worksheet. You can achieve this using the Worksheet.ToImage() method by modifying its parameters to specify the desired range. The following steps will show you how to do it.
Steps to convert cell ranges to images:
Create a Workbook object.
Specify the file path to load Excel files with the Workbook.LoadFromFile() method.
Retrieve a worksheet through the Workbook.Worksheets[] property.
Specify the cell range and convert it to an image using the Worksheet.ToImage() method.
Save the image as a PNG.
from spire.xls import *
from spire.xls.common import *
# Create a Workbook object
workbook = Workbook()
# Load an Excel file
workbook.LoadFromFile("/Population.xlsx")
# Get the first worksheet
sheet = workbook.Worksheets[0]
# Convert a specific cell range of the worksheet to an image
image = sheet.ToImage(5, 1, 10, 4)
# Save the image to a PNG file
image.Save("/CellRangeToImage.png")
workbook.Dispose()
The Conclusion
In this article, we’ve walked through the steps of converting an Excel worksheet into a high-quality image using Python. We’ve also covered how to remove unwanted white margins from the resulting images and how to export specific cell ranges to an image. With these techniques, you can easily and efficiently present your Excel data in a visually appealing way, making it perfect for reports, presentations, and more!
Subscribe to my newsletter
Read articles from Casie Liu directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
