Java Security: Best Practices for Securing Java Applications
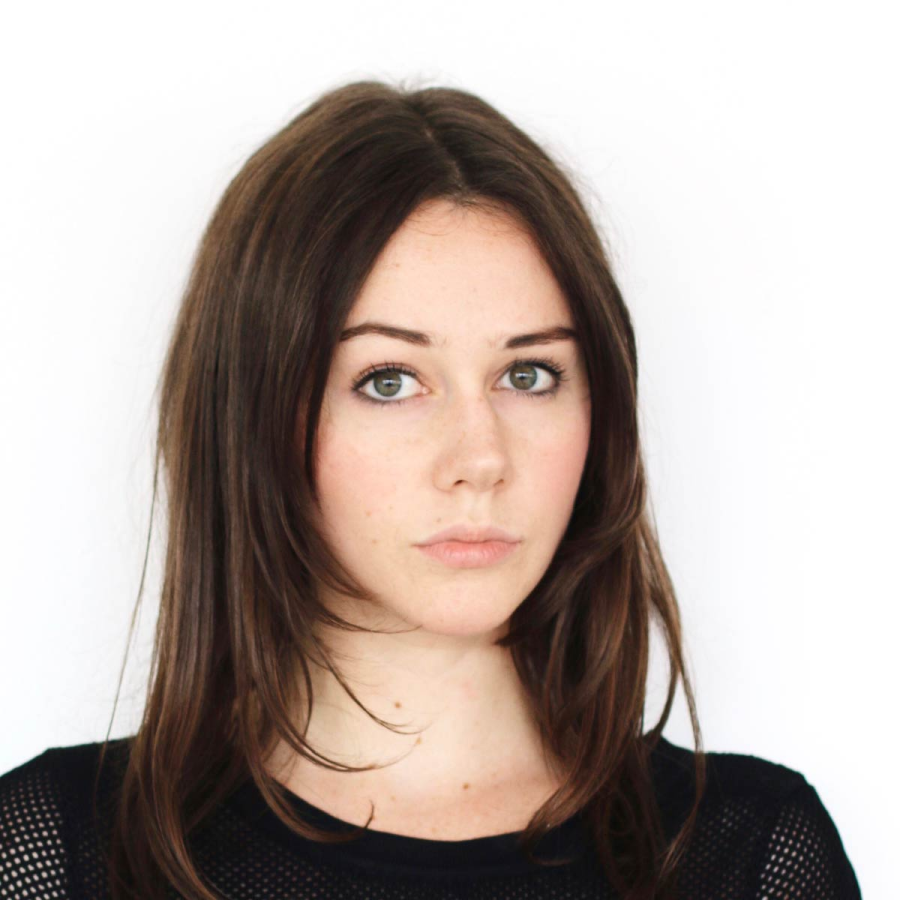

Secure Java code is the result of every Java developer adhering to code guidelines and best practices. Make sure there are no security holes in your code that may be used by hackers. Security holes in several programs have recently been found and used by hackers, affecting even large corporations such as eBay, the CIA, and the IRS.
To build secure Java code and apps, it is important to follow these rules. These measures will assist in avoiding identified malicious attacks and reduce the likelihood of security vulnerabilities introduced by Java developers.
Examples of The Potential Dangers to Java Security
1. SQL Injection Attacks
One form of cyberattack is SQL Injection, which targets the database layer of online applications. By inserting SQL code into input areas or requests, hackers may damage the program's database and gain illegal entry, steal data, or delete important records.
SQL Injection occurs when a website fails to validate user inputs, allowing attackers to insert malicious commands into the database using input boxes. It's as if you could manipulate your keystrokes to mislead the website into executing malicious code.
The three main kinds of SQL Injection attacks are:
Union-Based SQL Injection
Classic SQL Injection
Time-Based Blind SQL Injection
2. Cross-site scripting (XSS)
Hackers commit this crime when they insert malicious code into websites to damage other users.
Stored XSS: When visitors visit a specific website, malicious scripts that have been saved on a specific server are provided to them.
Reflected XSS: Without doing the necessary checks, the server sends the injected script to the user's browser through a URL or form.
3. Insecure Data Storage
When private information is not adequately or incorrectly protected during storage, we say that it is insecure. User credentials, private information, and cryptographic keys are all part of it.
Vulnerability in Java programs occurs when data is not properly protected, which can lead to sensitive information being exposed or unauthorized access occurring. Data tampering, identity theft, and unauthorized access are all possible outcomes of insufficient data storage criteria.
4. Man-in-the-Middle (MitM) Attacks
When an unethical third party subtly alters the conversation between two people, this is called a Man-in-the-Middle (MitM) attack. Security flaws in the client-server communication channel can be exploited by malicious actors in Java applications, putting sensitive data at risk during data transmission.
Gain a deeper understanding of the architecture of Java microservices. The only way to reduce the likelihood of security breaches during the development of Java applications is to implement good preventive measures.
What Are The Potential Causes Of Java Security Being Compromised?
The security of Java programs is dependent on its design, coding, and configuration, much like any other language. Some potential threats to the security of Java applications are as follows:
Problem with configuration: Inadequate password policies, incorrect permissions, or unprotected defaults are just a few examples of the security holes that may be introduced into Java by poorly set up applications or servers.
Code that is not secure: Security flaws such as SQL injection and cross-site scripting (XSS) can easily compromise Java programs if proper development standards are not followed.
Outdated software: Several security holes can be introduced into programs if third-party libraries and dependencies are not kept up to date or if older versions of Java are used.
Incorrect validation of input: Security flaws can occur when user input data is not properly validated and cleaned.
Insufficient testing for security: Vulnerabilities in security can remain undiscovered due to insufficient testing, which includes both penetration testing and code reviews.
Third-party dependencies: Security flaws in third-party libraries and frameworks pose a threat to Java programs since they depend on these components.
Awareness of Security Measures: The depth of caution and skill within managers and programmers greatly affects the security of Java applications.
There are limits to Java that developers need to be aware of and work around, even though it provides strong security. Many companies put vulnerable code into production. Deadlines, low sense of risk, and late bug discovery are the primary factors, accounting for 45% of the total. It is crucial to incorporate security testing early on in the development process since application security relies on secure coding techniques, frequent upgrades, correct configuration, and ongoing security testing. Keep in mind that there are many reasons why insecure code might be released; for example, security tests may not catch all bugs. This does not necessarily mean that the security program is poor.
Important Security Practices In Java Application Development
1. Stick to Well-Tried Library Sources
Public libraries and frameworks provide the basis for a significant portion of application code. A malicious hacker can potentially damage your application due to weaknesses within these libraries.
Ensure that you only utilize reliable libraries and maintain them updated with the newest versions since organizations trust these libraries with their company and reputation. Examine whether they need any security updates or have any known vulnerabilities.
2. Avoid Serialization
Oracle has declared its intention to eliminate Java serialization in the future due to the security risks it poses. Applications developed by Cisco and Jenkins were revealed to have serialization vulnerabilities not long ago.
It doesn't matter if a library or framework is to blame, as long as your program accepts serialized Java objects, it is susceptible. Make sure you consider all potential exposures before making an interface serializable. Another trap to watch out for is inadvertently subclassing or defining a serializable interface on a class that is subject to security.
3. Always Hash User Passwords
Keep all passwords encrypted at all times. Passwords should be hashed using a suitable hashing method, such as SHA-2, and ideally a salted hash. A password is "hashed" when it is transformed into an encrypted form. By combining the password with a specified key that is known to the application, a hashing technique is used to obtain the hash value.
4. Eliminate Exceptions That Contain Sensitive Data
To help an attacker misuse your system, exception objects might include important information. By manipulating the input parameters, an attacker can get access to the application's internal workings and structures. Never forget that the content of an error message and its kind might reveal sensitive information.
Consider the FileNotFoundException message as an example. These alerts include details on the file system's architecture and the kind of error that shows which file is missing.
Both the exception message and the exception type should be filtered for the sake of application security in Java programming.
5. Avoid Recording Private Data
Hackers should do all in their power to stop data breaches because of the enormous damage they cause to people and businesses. Criminals highly value and handle sensitive information such as passwords, bank account details, credit card numbers, and passport numbers. Avoid storing sensitive information like this in log files and take precautions to prevent it from being discovered in cleartext searches.
If you must record sensitive information, such as credit card numbers, consider recording only a portion of the number, such as the last four digits, and then encrypt it using a trusted library. You shouldn't create your encryption code.
6. Error Handling and Logging
Error messages captured in the log files and those sent by users could inadvertently expose sensitive information like account or system data.
A more secure approach would be to provide users with generic screen error warnings. In addition, when writing log error messages, make sure to exclude any information that may be beneficial to a hacker to break into your systems. This will aid support teams in their investigation of production issues.
7. Compose Basic Java Programs
In most cases, the rule of thumb is that simple Java code is secure Java code. If you want your code to be simple and safe, follow these guidelines:
Avoid making it less functional by keeping it as basic as feasible.
Make use of SonarQube and other code quality-checking tools. This program will scan your most recent code release for new vulnerabilities and do continual inspections of the code quality. Fixing a flaw or vulnerability after it has been released into production is far more difficult than preventing it.
Reduce the quantity of data exposed by your code to a minimum. To make your code secure and easy to maintain, it's best to hide implementation details.
Use the access modifiers in Java to your advantage. Class, method, and attribute access levels should be declared as restricted as feasible. Put everything that has a private setting to private.
Keep your API and interface objects as little as feasible at all times. Make components communicate in the lowest feasible scope by decoupling them. Your application will remain secure even if a breach were to affect just one part of it.
8. Validation Of Input Data
To protect sensitive information from malicious actors, such as SQL injection and Cross-Site Scripting (XSS), Java programmers should apply strong input validation. One important method of protection is query parameterization. The use of input validation frameworks or libraries, such as OWASP Enterprise Security API, is strongly advised.
9. Cryptography
To prevent monitoring of sensitive data while it is being sent or stored, Java provides strong encryption and decryption libraries. To store and authenticate passwords securely without exposing the real password, these libraries use cryptographic hashing techniques.
10. Authentication and Authorization Authentication
Secure your system from unauthorized access using strong user authentication techniques enabled by Java frameworks like Spring Security or Apache Shiro. The use of two-factor authentication (2FA) and other robust identity verification techniques should be implemented. Roles and attributes-based access control determines who has permission to do what and what data they can see. As a result, it ensures that authorized users can only access certain resources.
11. Tracking And Documenting
Security events and abnormalities can be logged by Java programs. Unusual patterns or possible security breaches can be better detected with the use of log analysis technologies. To analyze web traffic and system activity to identify cyberattacks, intrusion detection systems (IDS) may be used to build bespoke IDS solutions or interact with existing ones. Also, penetration testing frameworks and vulnerability scanners built on the Java platform may find security holes in Java apps and fix them, making them less vulnerable to attacks.
12. Ensuring The Security Of Networks
Interceptors and filters for networks: Java is a good fit for managing and setting up firewalls and filters to regulate traffic and prevent harmful requests. Additionally, it is compatible with SSL/TLS protocols, which allow for the transmission of data securely over networks and prevent snooping on encrypted communications.
13. Security Libraries And Frameworks
For typical security requirements like input/output validation and encryption, Java provides a variety of libraries and frameworks, including OWASP Java Encoder and Bouncy Castle. These solutions include safe coding standards such as masking special characters to avoid user data being executed as code and using prepared statements for database queries.
14. Vulnerability Scanning And Testing
Java application weaknesses can be found and fixed with the help of penetration testing frameworks and vulnerability examining tools like Snyk and OWASP Dependency-Check. Regular dependency scanning helps find and fix identified security holes.
15. Managing Patches And Security Updates
To fix identified holes quickly, Java programs must be updated and patched often. To find and fix security flaws in your codebase, you need to do code reviews and security audits often. Strong error handling and keeping sensitive information out of error messages are other good practices to follow.
16. Safe Session Administration
Leveraging secure cookies, appropriate session intervals, and renewing session IDs upon login, secure session management preserves user sessions.
17. Don't Use Hard-Coded Passwords
You shouldn't keep passwords, encryption keys, API keys, or other sensitive information in the code itself. It is ideal to manage sensitive data through configuration files or environment variables that have suitable access constraints.
18. Content Security Policy (CSP)
By identifying the allowed sources for loading and execution, CSP headers can help reduce the likelihood of XSS attacks. Headers such as X-Content-Type-Options, Strict Transport Security (HSTS), and Content Security Policy (CSP) can be configured in HTTP to do this.
19. Education And Training
In order to understand possible risks and the best ways to mitigate them, Java developers should acquire extensive education in knowledge of cybersecurity and secure coding standards. Organizations may improve the security of their Java applications and systems by making use of Java's features and integrating security practices into the development lifecycle. This empowers them to identify, avoid, and reduce cyberattacks with ease.
20. Prevent Injection Attacks
Injecting malicious code into a network is known as an injection attack. According to the OWASP, this attack type is the most pressing issue with online application security. An injection attack can occur in any application that permits users to input or upload data. In most cases, injection vulnerabilities are caused by inadequate validation of user input.
Summary
To summarize, while designing safe Java code, there are a few important considerations to keep in mind. During the design phase and code reviews, you should constantly keep security in mind when developing your application. Additionally, you should search for loopholes in your Java code and make use of the Java security APIs and libraries.
If you want to keep an eye on your code for security concerns, you should only utilize vendor tools that have excellent ratings. This means you need to look into all the different kinds of application attacks and make sure you take all the steps to avoid them. Protect yourself and your apps from harmful attacks and data theft by following the above-mentioned best practices for creating safe Java code applications in your company.
Subscribe to my newsletter
Read articles from Jenifer directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
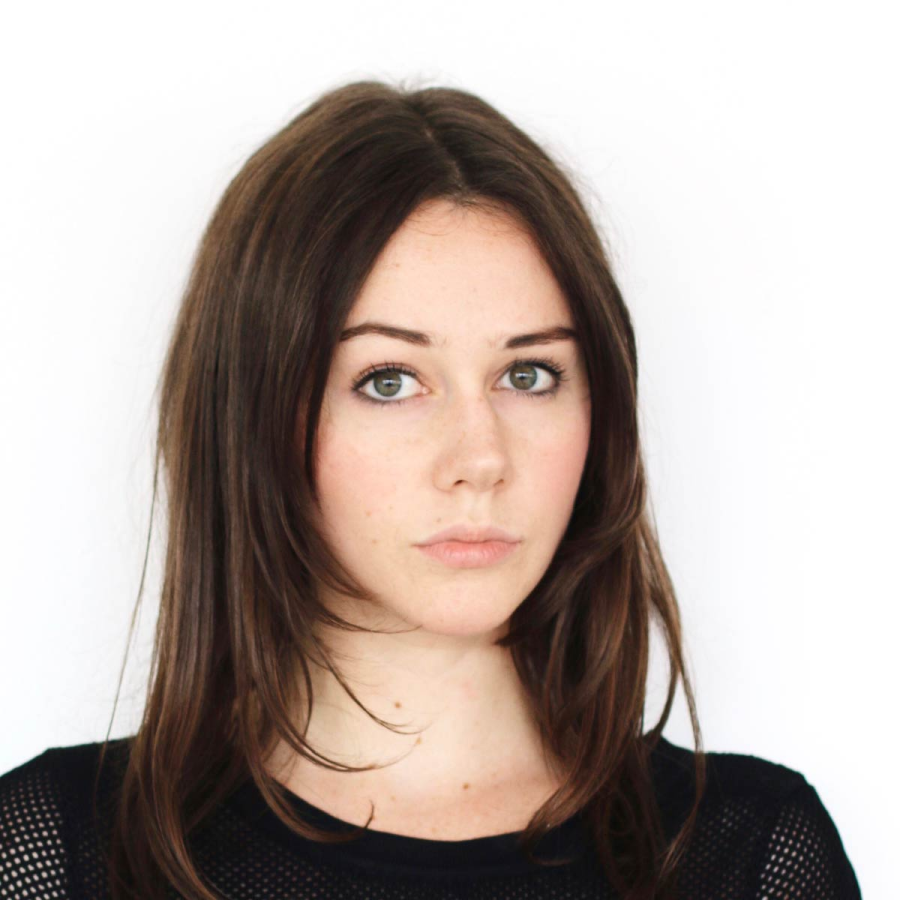