Mastering Type Safety in Dart: A Comprehensive Guide
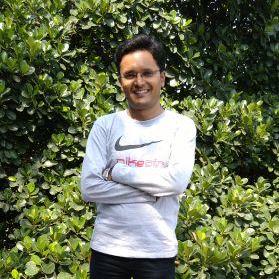

Introduction:
In this article, we will explore type safety feature of dart programming language.
What Is Type Safety?
Dart is type safe language and type safety in dart ensure that variable value always matches the variable static type, sometimes referred to as sound typing. For example, if we declare an int variable and accidentally try to assign string value to it, then we will get an error. This prevents unexpected errors in our app.
Type Safety in Dart is achieved using two different ways:
Compile Time checks (Static Type Checking)
Run time checks
Compile Time Checks (Static Type Checking):
In Dart, compile-time type safety ensures that the code we write doesn’t violate type rules before it even runs. Here’s a quick example:
void main(){
int age = 20;
age = "twenty"; // Compile time error
}
In the example above, we have an int variable named age with a value of 20. If we try to assign a string value to it, we’ll get a compile-time error. This is because Dart’s static analyzer ensures that the variable’s type matches the value we’re assigning.
Run Time Checks:
In Dart, run-time type safety ensures that values adhere to their type during execution. For instance:
void main() {
dynamic age = 'twenty-five';
print(age as int); // Throws a run-time error
}
In Dart, the dynamic keyword means type checking happens only when the program is running. If there’s a mismatch, Dart catches it and throws an error. So, even if we make a mistake, Dart’s got our back!
Type Inference:
Sometime we also declare variable like below.
var age = 20;
var name = "Manoj";
In the example above, we created two variables, age and name, and assigned them values. Dart can figure out what type these variables should be based on the values we give them. This is called Type Inference. For instance, since we gave age the value 20, Dart knows it should be an integer. Similarly, since we gave name the value “Manoj”, Dart knows it should be a string.
Benefits Of Type Safety:
Reduce Bugs: With compile time type safety, we can catch the type related bugs early in our code.
Boosts readability: With types enforced, our code is easier to understand for our teammates.
I have also created a YouTube video regarding this topic, so if you would like to watch a video tutorial, then please go to https://youtu.be/zJNnPQUO7f0
Conclusion:
In this article, we delved into the type safety feature of the Dart programming language, exploring how it ensures that variable values consistently match their declared types. We examined both compile-time and run-time checks, highlighting how they prevent type-related errors and enhance code reliability. Additionally, we discussed type inference and its role in simplifying code while maintaining type safety. The benefits of type safety, such as reducing bugs and improving code readability, were also emphasized. By understanding and leveraging Dart's type safety features, developers can write more robust and maintainable code. I hope you enjoyed reading the article.
Happy Coding!
Subscribe to my newsletter
Read articles from Manoj Kulkarni directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
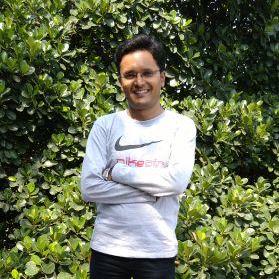
Manoj Kulkarni
Manoj Kulkarni
I am programmer, blogger and foodie. I just love computers, coding and technical things. I spend most of my free time working on building my skill set by reading technical articles about new technologies or books on software development and try to keep my self updated as much as I can.