#100DaysOfCloudDevOps Challenge — Day 09 — Git
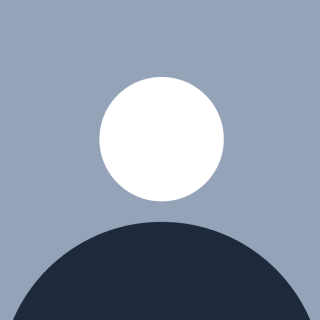

Git is a version control system, a tool that programmers use to manage and track changes to their source code over time. It allows developers to keep a history of all modifications made to their code. Git can be used through the terminal, command line, or PowerShell.
Why Use Git?
✅ Tracks code changes over time
✅ Enables team collaboration
✅ Allows branching & merging for safe development
What are GitHub and GitLab?
• Cloud-based platforms to store, collaborate, and manage repositories using Git.
Git Basics
• Distributed vs. Centralized Version Control – Enables all project versions to be stored and accessible to everyone.
• Repository – A folder (or root folder) where any type of file can be saved.
• Main/Master – The primary branch where all final modifications are merged.
• Branch – A separate version of the main/master branch where a team can safely make and test changes.
• Commit – A snapshot of changes saved in the repository.. Saves updates to the repository, overwriting the previous version.
• Push – Transfers changes from your local repository (on your computer) to a remote repository, making them visible to teammates.
• Pull – Fetches and merges changes from a remote repository (like GitHub) into your local repository.
• Merge – Combines one branch with another (usually into the main/master branch).
• Fork – Creates a personal copy of someone else’s repository, which then appears in your GitHub account.
• Clone – Copies a repository from a remote source to your local machine.
• Markdown – A lightweight formatting language designed to be easy to read, write, and edit in plain text. While HTML is for publishing, Markdown is for writing.
• WYSIWYG vs. WYSIWYM – “What You See Is What You Get” vs. “What You See Is What You Mean.”
Essential Git Commands
Command | Purpose |
git init | Initialize a new Git repository |
git clone <repo-url> | Copy a remote repository to your machine |
git status | Check the current state of your working directory |
git add <file> | Stage changes for commit |
git commit -m "message" | Save changes with a commit message |
git push origin <branch> | Upload commits to a remote repo |
git pull origin <branch> | Download the latest changes from the remote repo |
git merge <branch> | Merge a branch into the main branch |
git branch | List branches |
git checkout -b <branch> | Create and switch to a new branch |
git log --oneline | View commit history |
Hands-On
Initializing a Git Project
# Step 1: Navigate to your project folder
cd path/to/your/project
# Step 2: Initialize Git in the folder
git init
# Step 3: Link the local repository to GitHub
git remote add origin https://github.com/yourusername/repository-name.git
# Step 4: Create a file and commit changes
echo "# My First Repository" > README.md
git add README.md
git commit -m "Initial commit"
# Step 5: Push the code to GitHub
git push -u origin main
Git Advanced: Workflows, Rebasing, Merging, Resolving Conflicts
Git Workflows
• Feature Branch Workflow: Developers create feature branches and merge them into main.
• Gitflow Workflow: Uses develop, feature, release, and hotfix branches.
• Forking Workflow: Used for open-source projects where contributors fork repositories.
Rebasing vs. Merging
• Merging (git merge): Combines two branches, keeping both histories.
• Rebasing (git rebase): Moves your branch on top of another branch, rewriting history for a cleaner log.
Resolving Conflicts
• When two developers edit the same file, Git may ask for manual conflict resolution.
• Conflicts must be fixed manually before committing changes.
Hands-On
Creating and Merging Branches
1. Create a feature branch
git checkout -b feature-branch
2. Make changes and commit
echo "New Feature" >> feature.txt
git add feature.txt
git commit -m "Added new feature"
3. Merge with main branch
git checkout main
git merge feature-branch
git push origin main
Rebasing a Branch
1. Switch to the feature branch
git checkout feature-branch
2. Rebase onto main
git rebase main
3. Coding – Conflict Resolution Example
Simulating a Conflict
1. User A (Main Branch) Updates a File
echo "Hello from User A" > greetings.txt
git add greetings.txt
git commit -m "User A's change"
git push origin main
2. User B (Feature Branch) Updates the Same File
git checkout -b feature-branch
echo "Hello from User B" > greetings.txt
git add greetings.txt
git commit -m "User B's change"
git push origin feature-branch
3. Merging Causes a Conflict
git checkout main
git merge feature-branch
• Git will alert you of a conflict in greetings.txt.
Resolving the Conflict
1. Open greetings.txt and manually edit the conflicting lines.
2. Add the resolved file and commit:
git add greetings.txt
git commit -m "Resolved merge conflict"
git push origin main
Best Practices for Writing Git Commit Messages
1. Why Are Good Commit Messages Important?
• Help track changes and understand what was modified.
• Make it easier for team members to review and collaborate.
• Improve debugging by clearly describing the context of changes.
• Keep the Git history clean for future reference.
2. Best Practices for Writing Commit Messages
A. Use the Conventional Commit Format
- A structured commit message format improves readability and automation. The general structure is:
<type>(<scope>): <short description>
<optional detailed explanation>
<optional issue reference>
Example:
feat(auth): add JWT authentication for login
Implemented JWT-based authentication for user login. The token is now stored in local storage.
Closes #42
- With JIRA Ticket or Work Order Number
<JIRA-TICKET> <type>(<scope>): <short description>
<optional detailed explanation>
<optional issue reference>
Example:
PROJ-123 feat(auth): add OAuth2 login support
Implemented OAuth2 login via Google and GitHub. Users can now authenticate using third-party providers.
Closes PROJ-123.
B. Keep the Commit Message Clear and Concise
• First line: 50 characters max, written in imperative tense (e.g., “Add”, “Fix”, “Update”).
• Second line: Leave blank.
• Third line (optional): More details, reasoning, or references.
❌ Bad Example:
Updated the login system
✅ Good Example:
fix(auth): resolve login bug causing 500 error
Fixed an issue where logging in with an incorrect password would crash the server. Now, the API returns a proper error message.
C. Use Meaningful Commit Types
Use a commit type that clearly defines the purpose of the change. Some standard types include:
Type | Purpose |
feat | Introduces a new feature |
fix | Fixes a bug |
chore | Minor changes (e.g., updating dependencies) |
docs | Updates documentation |
style | Code style changes (formatting, linting) |
refactor | Improves code structure without changing functionality |
test | Adds or updates tests |
perf | Performance improvements |
✅ Examples:
feat(ui): add dark mode toggle
fix(cart): correct total price calculation
docs(readme): update installation instructions
D. When to Commit?
• Small, logical changes – Avoid committing huge chunks of code at once.
• One feature or fix per commit – This makes rollbacks and debugging easier.
• Before pushing to GitHub, review your commits using:
git log --oneline
E. Scope (Optional but Recommended)
• Defines the area of change: ui, api, auth, database, checkout, etc.
3. Hands-On Practice for Writing Better Commits
Scenario 1: Fixing a Bug
❌ Bad Commit Message:
fixed issue
✅ Good Commit Message:
fix(api): resolve undefined variable in user login
Corrected a reference error causing API requests to fail when a user logs in. The issue was due to a missing variable in authController.js.
Scenario 2: Adding a Feature
❌ Bad Commit Message:
added new feature
✅ Good Commit Message:
feat(profile): implement user profile editing
Users can now update their profile details, including name and bio. Integrated with the backend for data persistence.
Scenario 3: Updating Documentation
❌ Bad Commit Message:
updated docs
✅ Good Commit Message:
PROJ-123 docs(contributing): clarify branching strategy
Added details on how contributors should create feature branches and submit pull requests.
The Commit Message Checklist
✅ Optional: Start with a JIRA Ticket or Work Order Number (PROJ-123)
✅ Use structured commit types like feat, fix, docs, etc.
✅ Use the imperative tense (“Add” instead of “Added”)
✅ Keep the first line short and meaningful (≤50 characters)
✅ Add extra context in a second paragraph if necessary
✅ Reference issues using Closes #issue-number
✅ Commit frequently but logically – one change per commit
Learning Git is not just essential for managing code, but it’s also a fun and powerful skill that helps you stay organized and efficient as an Engineer. Mastering Git, GitHub, GitLab, and writing effective commit messages, you are ready to work collaboratively with teams and keep your projects well-organized and easy to maintain. Happy coding!
Find the GitHub repo here.
If you find this blog helpful too, give back and show your support by clicking heart or like, share this article as a conversation starter and join my newsletter so that we can continue learning together and you won’t miss any future posts.
Thanks for reading until the end! If you have any questions or feedback, feel free to leave a comment.
Subscribe to my newsletter
Read articles from anj directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by