Understanding prototype vs __proto__ in JavaScript

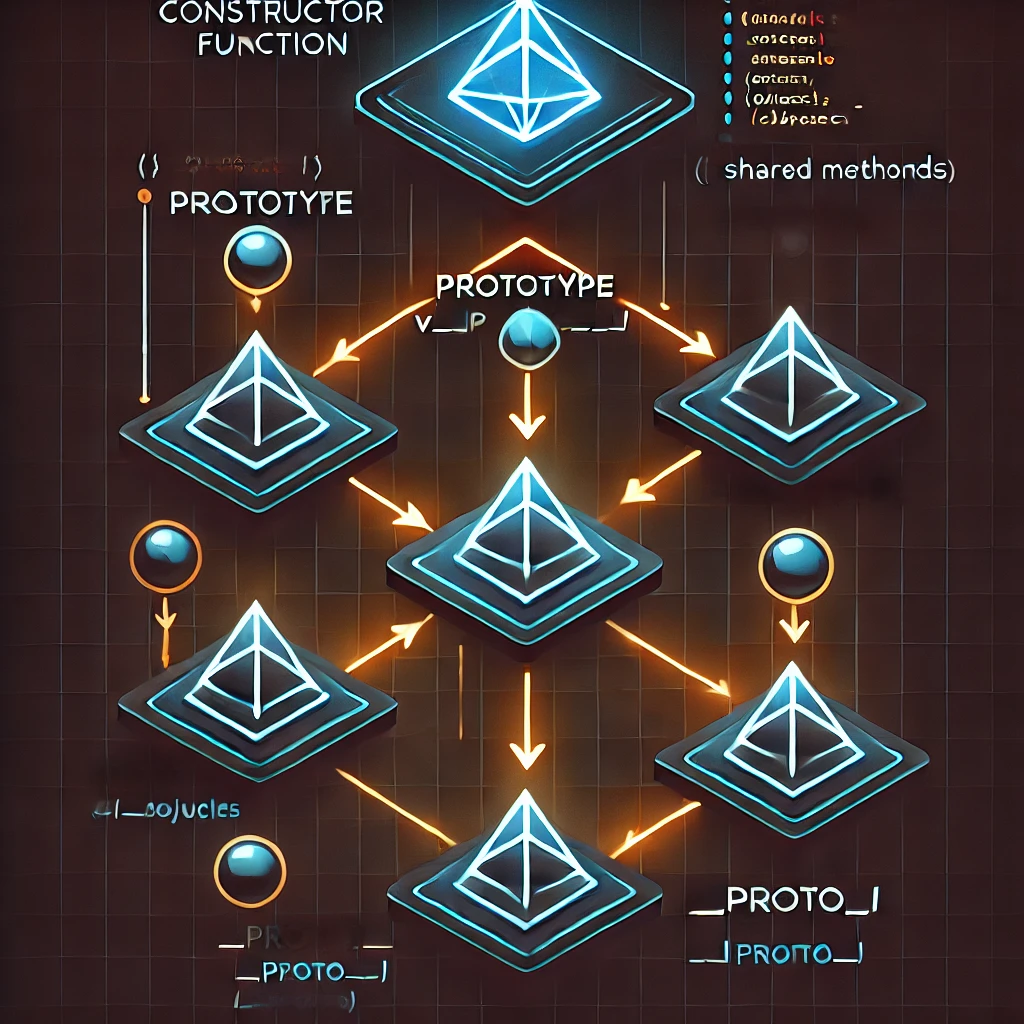
When working with JavaScript, understanding prototype
and __proto__
is crucial for mastering object-oriented programming. In this article, we’ll explore these concepts with examples and learn how they impact inheritance.
What is prototype
?
The prototype
property is used in constructor functions to add shared properties and methods to objects created from that constructor. This helps in memory optimization since all instances share the same prototype methods instead of duplicating them.
Example:
function Person(name, age) {
this.name = name;
this.age = age;
}
// Adding a method to the prototype
Person.prototype.greet = function() {
console.log(`Hello, my name is ${this.name}`);
};
const person1 = new Person("Alice", 25);
person1.greet(); // Output: Hello, my name is Alice
In the example above, greet
is defined in Person.prototype
, meaning all instances of Person
will share this method.
What is __proto__
?
__proto__
is an internal property of an instance that points to its constructor’s prototype. This establishes a prototype chain, allowing objects to inherit properties and methods.
Example:
const person1 = new Person("Batman", 28);
console.log(person1.__proto__ === Person.prototype); // true
Since person1
is an instance of Person
, its __proto__
points to Person.prototype
, allowing it to access methods like greet()
even though they are not defined directly on person1
.
What is the role of the new
keyword?
In JavaScript, the new
keyword plays an important role when creating objects using constructor functions:
It creates a new empty object.
It sets the object’s
__proto__
to the constructor’sprototype
.It binds
this
inside the constructor to the new object.It returns the new object.
Example:
const person1 = new Person("Bruce Wayne", 30);
Here, person1.__proto__
is set to Person.prototype
, enabling inheritance.
How to Inherit Properties Without extends
?
In JavaScript, inheritance can be achieved without using the extends
keyword by directly modifying the prototype.
Example:
class Info {
swim() {
return "Swimming";
}
}
class Animal {
bark() {
return "Barking";
}
}
Animal.prototype.swim = function() {
return "Swimming";
};
const obj1 = new Animal();
console.log(obj1.swim()); // Output: Swimming
console.log(obj1.bark()); // Output: Barking
Here, even though swim()
is not defined inside the Animal
class, we added it manually to Animal.prototype
, making it available to all Animal
instances.
Conclusion
In this article, we explored how prototype
and __proto__
work in JavaScript. We learned that:
The
prototype
property is used to define shared methods for all instances of a constructor.The
__proto__
property is an instance property that links an object to its prototype.The
new
keyword plays a vital role in setting up the prototype chain.We can achieve inheritance without
extends
by manually adding properties to a constructor’s prototype.
Subscribe to my newsletter
Read articles from rohit directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
