How I Made My Next.js Blog Serve Markdown Files for Agent Experience SEO (AX-SEO)
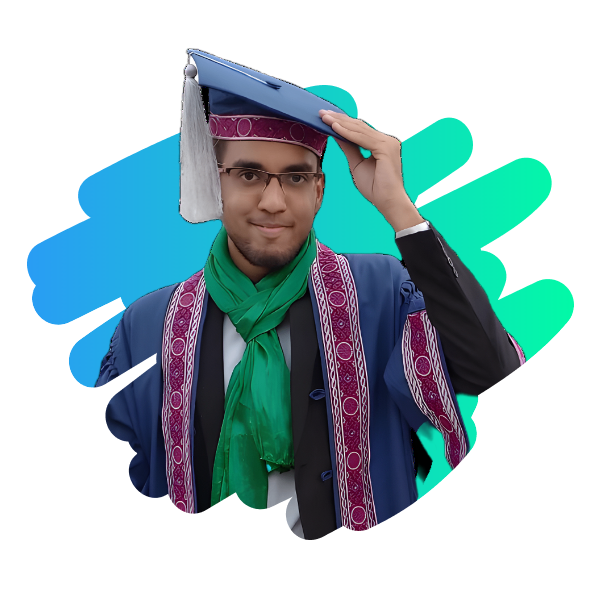

I’ll show you how to serve raw Markdown files in a Next.js blog using middleware and API routes, enabling Agent Experience SEO (AX-SEO).
AX-SEO focuses on optimizing content for AI agents, LLM-powered search engines, and automated crawlers that now play a major role in content discovery.
By the end of this post, you’ll be able to:
✅ Implement Next.js middleware for .md
URL rewriting.
✅ Set up API routes to fetch and serve Markdown content.
✅ Optimize your blog for Agent Experience SEO (AI-driven search).
✅ Provide clean, structured data for AI-powered search engines.
Traditional SEO is no longer enough. AI search engines, LLM-powered assistants (ChatGPT, Perplexity, Google’s AI Overviews, etc.), and autonomous agents are now indexing and ranking content differently.
Why does AX-SEO matter?
AI agents prefer structured content – Raw Markdown is easier to parse than HTML.
LLMs use Markdown for training & retrieval – AI search engines extract structured text more efficiently.
AX-SEO improves discoverability – AI-driven platforms rank content based on how well agents can process it.
If you want your blog optimized for AI-driven search, this guide is for you!
Most blogs serve only HTML, making extracting structured information hard for AI tools. Common mistakes include:
❌ Not offering raw text formats – AI agents struggle with unnecessary markup.
❌ Ignoring structured data for LLMs – AI search engines prioritize clean content.
This guide avoids these mistakes and ensures a future-proof content strategy for AI indexing.
How I Did It
Step 1: Middleware for .md
Routing
I used Next.js Middleware to detect .md
URLs and redirect them to an API route:
import type { NextRequest } from "next/server";
import { NextResponse } from "next/server";
export function middleware(req: NextRequest) {
const url = req.nextUrl.clone();
if (url.pathname.endsWith(".md")) {
url.pathname = `/api${url.pathname}`;
return NextResponse.rewrite(url);
}
return NextResponse.next();
}
export const config = {
matcher: ["/blog/:path*.md", "/projects/:path*.md"],
};
Step 2: API Route to Serve Markdown
Next, I created API endpoints to fetch Markdown content:
import { readMarkdown } from "@/server/read-markdown-file";
import { NextRequest } from "next/server";
export async function GET(_req: NextRequest, { params: { slug } }: { params: { slug: string } }) {
return readMarkdown(slug);
}
Step 3: Fetching Markdown Content from CMS
The readMarkdown
the function retrieves the raw Markdown content:
import useBlogSlug from "@/features/posts/hooks/useBlogSlug";
import { NextResponse } from "next/server";
export async function readMarkdown(slug: string) {
try {
const {
post: {
content: { markdown },
},
} = await useBlogSlug({ params: { slug: slug.replace(".md", "") } });
return new NextResponse(markdown, {
headers: {
"Content-Type": "text/plain",
},
});
} catch (error) {
console.error("Error reading markdown file:", error);
return new NextResponse(JSON.stringify({ message: "Markdown file not found" }), { status: 404 });
}
}
Step 4: Adding a Markdown Link to the Blog
To make the Markdown file accessible, I added a link on each blog page:
<Link href={`/api/${params.type}/${params.slug}.md`} passHref>
View Markdown
</Link>
Now, every post has a “View Markdown” button that links to the raw .md
version.
How This Boosts Agent Experience SEO (AX-SEO)
✅ AI-Optimized Content – Markdown is easier for AI bots to read than HTML.
✅ AX-SEO Ready – AI-powered search engines prefer structured text for ranking and retrieval.
✅ Faster AI Crawling – Lightweight Markdown improves indexing speed.
✅ Better Content Extraction – LLMs can fetch raw text for RAG-based applications.
✅ Scalable & Future-Proof – Works for blogs, docs, and AI knowledge bases.
Final Thoughts
With this approach, I made my Next.js blog serve Markdown dynamically, benefiting both humans and AI bots.
📂 Source Code: GitHub Repository
🌍 Live Website: sm-y.dev
I hope this guide helps you boost your blog’s AX-SEO and AI search visibility! 🚀
Subscribe to my newsletter
Read articles from Syed Muhammad Yaseen directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
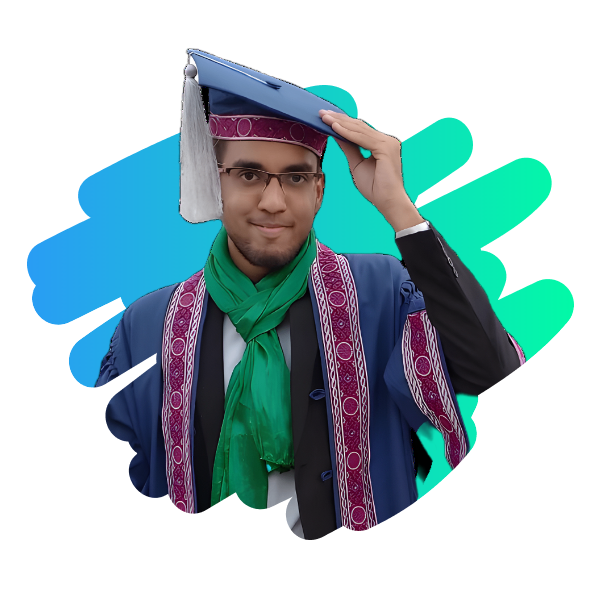
Syed Muhammad Yaseen
Syed Muhammad Yaseen
Hi there 👋 This is SMY Curious to know my standout highlights? Let me share some with you: 🎯 Professional Experience: ❯ Full Stack Engineer with ~ 4 years of expertise in the JavaScript ecosystem (Backend / Frontend / AWS). Consistently delivers high-quality SaaS applications by developing, testing, documenting, and maintaining while ensuring code quality and reliability through unit testing. ❯ Regularly learning and upskilling myself on various technical and soft skills by participating in initiatives, courses, and developing POCs. 🎯 Academic Experience: ❯ Pursued a Bachelor of Science in Computer Science with a 3.72 CGPA and a four-time Merit Scholarship holder. ❯ My academic accomplishments have been further recognized with awards, and I have actively contributed as a volunteer, expanding my horizons beyond traditional educational boundaries. 🎯 Publications: ❯ Passionate about leveraging technology to drive positive change, I am an avid writer and community contributor. I love sharing my insights, experiences, and knowledge through thought-provoking articles and engaging discussions. ❯ Discover a collection of my insightful articles on various topics by visiting my profile on hashnode 🌐 https://smy.hashnode.dev/ 🎯 Interests: ❯ At 4 years old, I was fascinated by computers, starting with gaming on DOS and exploring Pentium III, solidifying my fascination and paving the way for a lifelong journey. ❯ Fast forward to today, and I find myself immersed in the ever-evolving landscape of technology. I'm passionate about leveraging technology to solve real-world challenges and make a positive difference in our interconnected world. 👋 If you're passionate about technology too, or if you're eager to explore the vast opportunities it presents, let's connect 🤝 LinkedIn: https://www.linkedin.com/in/sm-y/